Fetching data from APIs using fetch() In Hindi
जावास्क्रिप्ट प्रोग्रामिंग लैंग्वेज में फ़ेच() फ़ंक्शन प्रोग्राम में एक ऑनलाइन नेटवर्क रिक्वेस्ट को प्रोसेस करने के लिए एक एडवांस्ड जावास्क्रिप्ट प्रोसेस या मेथड है. जैसे कि किसी नेटवर्क से एपीआई से डेटा इनफार्मेशन को कलेक्ट करना है। जहा फेच() को वेब ब्राउज़र में क्रिएट किया गया है, जहा फ़ेच फंक्शन एपीआई का बिल्ट-इन फीचर्स है। जहा फेच() आपको ओल्ड XMLHttpRequest के अपोजिट, फ़ेच() अधिक पॉवरफुल, फ्लेक्सिबल वेब ब्राउज़र क्लाइंट नेटवर्क रिक्वेस्ट फंक्शन है. जहा फेच() एक प्रॉमिस को रिटर्न करता है, जिससे जावास्क्रिप्ट प्रोग्राम में एसिंक्रोनस प्रोग्राम ऑपरेशन को मैनेज और कण्ट्रोल करना आसान हो जाता है।
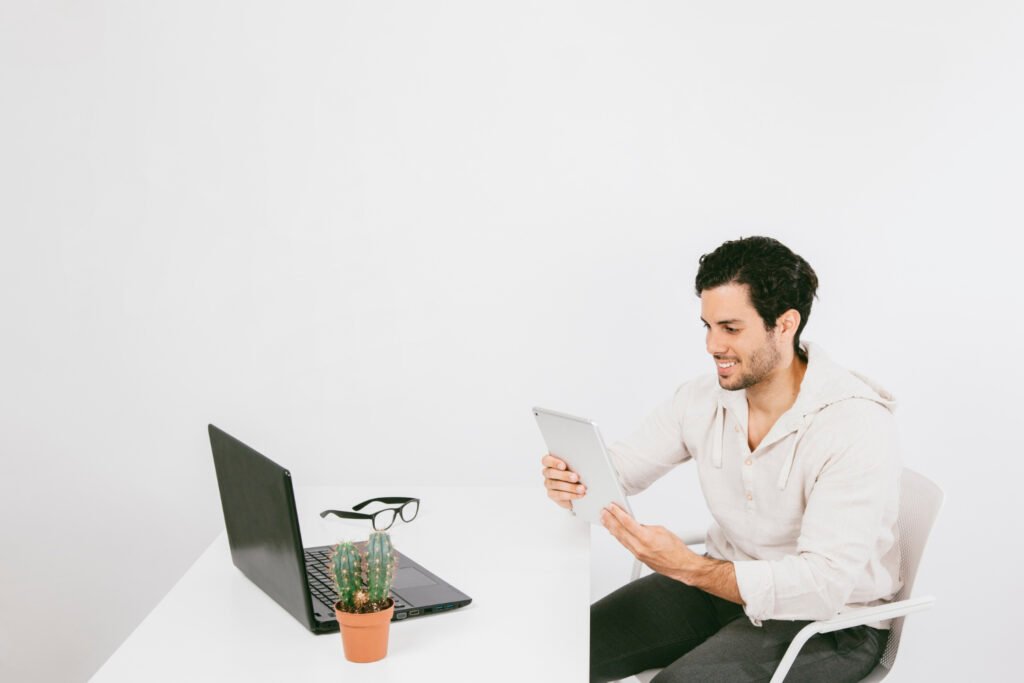
Basic syntax of fetch() in JavaScript.
The basic syntax of fetch() is as follows.
fetch(url, options)
.then(response => response.json()) // it Parse the JSON from the response location
.then(data => console.log(data)) // it use to Handle the data
.catch(error => console.error(‘Error:’, error)); // it use to Catch and log any request errors
- url – यह फेच फंक्शन में वह यूआरएल रिसोर्सेज लोकेशन है, जिससे आप क्लाइंट रिक्वेस्ट में डेटा को रिसीव करना चाहते हैं।
- options – यहाँ ऑप्शन एक ऑप्शनल वेब रिक्वेस्ट को कस्टमाइज करने के लिए एक कॉन्फ़िगरेशन ऑब्जेक्ट है, जैसे, मेथड, हेडर, बॉडी, आदि है।
जावास्क्रिप्ट प्रोग्राम में डिफ़ॉल्ट, फ़ेच() फंक्शन से डेटा रिसीव करने के लिए GET मेथड का उपयोग किया जाता है। जबकि, जावास्क्रिप्ट प्रोग्रामर अपनी वेब डेवलपमेंट ज़रूरतों के आधार पर इसे POST, PUT या अन्य HTTP मेथड से सेंड करने के लिए मैन्युअल कस्टमाइज कर सकते हैं।
Getting data from public API in JavaScript.
यहाँ जावास्क्रिप्ट प्रोग्राम में JSONPlaceholder एपीआई जैसे पब्लिक एपीआई से डेटा को रिसीव करने का एक बेसिक एक्साम्प्ल दिया गया है. जो प्रोग्रामर को वेब रिक्वेस्ट टेस्टिंग के लिए सैंपल JSON डेटा प्रोवाइड करता है।
Example of getting user data from JSONPlaceholder API in a JavaScript program.
fetch(‘https://jsonplaceholder.typicode.com/users’)
.then(response => {
// here it Check if the response is successful
if (!response.ok) {
throw new Error(‘current Network response is not ok’);
}
return response.json(); // it use to Parse the JSON data from the response
})
.then(data => {
console.log(data); // it use to Handle the fetched data
})
.catch(error => {
console.error(‘it produce a problem with the fetch operation -‘, error);
});
Here in this example
- यहाँ इस एक्साम्प्ल में हम https://jsonplaceholder.typicode.com/users एंडपॉइंट से यूजर लिस्ट को रिसीव करते हैं।
- जहा हम ऑपरेशन को टेस्ट करते हैं कि क्या रिस्पांस response.ok का उपयोग करके ऑपरेशन सक्सेस हुआ है। यदि ऐसा नहीं हुआ है, तो हम एक एरर को थ्रो करते हैं।
- इसके बाद प्रोग्रामर जरूरत के अनुसार response.json() फंक्शन का उपयोग करके JSON डेटा को पार्स करते हैं, जो एक प्रॉमिस को रिटर्न करता है।
- यदि ये सभी ऑपरेशन प्रॉपर्ली हो जाते है, तो प्रोग्रामर डेटा को कंसोल में लॉग करता हैं।
- यदि इस ऑपरेशन में कोई एरर जनरेट होती है. जैसे, नेटवर्क प्रोब्लेम्स, अमान्य JSON, आदि है, तो इसे catch() ब्लॉक में कैच किया जाता है।
Using fetch() with POST requests in JavaScript.
जावास्क्रिप्ट में एपीआई को क्लाइंट डेटा रिक्वेस्ट सेंड करने के लिए. जैसे, एक नया रिसोर्सेज क्रिएट करना, जावास्क्रिप्ट प्रोग्रामर वेब रिक्वेस्ट में मेथड, हेडर और बॉडी को इंडीकेट करने वाले ऑप्शन ऑब्जेक्ट को पास करके POST मेथड को अप्लाई कर सकते हैं।
Example of sending data with a POST request.
const newUser = {
name: ‘David’,
email: ‘david@yahoo.com’,
username: ‘david’
};
fetch(‘https://jsonplaceholder.typicode.com/users’, {
method: ‘POST’, // it use t Specify the HTTP method
headers: {
‘Content-Type’: ‘application/json’ // it use to Specify that we are sending JSON
},
body: JSON.stringify(newUser) // it use to Convert the JavaScript object into JSON
})
.then(response => response.json()) // it use to Parse the JSON response
.then(data => {
console.log(‘New user record construct -‘, data); // it use to Log the response from the server
})
.catch(error => {
console.error(‘Error:’, error); // it use to handle any post method errors
});
Here in this example.
- यहाँ हम एक ऑब्जेक्ट newUser को डिफाइन करते हैं, जिसे प्रोग्रामर एपीआई को सेंड करना चाहते हैं।
- यहाँ हम POST मेथड को इंडीकेट करते हैं, और Content-Type हेडर को application/json पर मूव कर सेट करते हैं. क्योंकि यहाँ हम JSON डेटा को सेंड कर रहे हैं।
- जहा वेब रिक्वेस्ट का मुख्य पोरशन newUser ऑब्जेक्ट का JSON स्ट्रिंग वर्जन है।
- यहाँ रिस्पांस JSON के रूप में पार्स की जाती है, और हम रिस्पांस को कंसोल में लॉग करते हैं।
Handling response status codes in JavaScript.
जावास्क्रिप्ट प्रोग्राम में यह जानने के लिए कि आपका एप्लिकेशन प्रॉपर्ली बिहेव कर रहा है, वेब रिक्वेस्ट में HTTP रिस्पांस पोजीशन कोड को ठीक से मैनेज करना इम्पोर्टेन्ट है। जैसे, एपीआई 404 (नॉट फाउंड) या 500 (इंटरनल सर्वर एरर) को रिटर्न कर सकता है, जिससे आपको किसी डेटा का उपयोग करने से पहले उसे टेस्ट करना होगा।
Handling HTTP status codes in JavaScript example.
fetch(‘https://jsonplaceholder.typicode.com/users’)
.then(response => {
if (!response.ok) {
throw new Error(`HTTP request error produce, check the Status: ${response.status}`);
}
return response.json(); // it Parse JSON where the response is successful
})
.then(data => {
console.log(data); // it use to Handle the data
})
.catch(error => {
console.error(‘it produce a problem with the fetch request data operation -‘, error); // it use to Handle any kind of request errors
});
Here in this example.
- यहाँ इस प्रोग्राम में हम टेस्ट करते हैं कि क्या वेब https response.ok ट्रू है, जो स्टेटस कोड 200-299 को इंडीकेट करता है।
- यदि यहाँ रिस्पांस सक्सेस्फुल नहीं होती है, तो हम प्रोग्राम डिबगिंग पर्पस के लिए स्टेटस कोड के साथ एक एरर को थ्रो करते हैं।
- यदि यहाँ रिस्पांस सक्सेसफुल होती जाती है, तो हम रिस्पांस को पार्स करने के लिए .json() फंक्शन मेथड का उपयोग करते हैं।
Async/Await syntax for fetch request in JavaScript.
जहा जावास्क्रिप्ट प्रोग्राम में fetch() फंक्शन एक प्रॉमिस को रिटर्न करता है, तो जावास्क्रिप्ट प्रोग्रामर अधिक रीडेबल प्रोग्राम एसिंक्रोनस कोड राइट के लिए async/await का उपयोग कर सकते हैं। जहा async/await प्रोग्रामर सिंक्रोनस कोड की तरह ही प्रॉमिसेस के साथ काम करने की परमिशन प्रोवाइड करते है।
Fetching data with async/await in JavaScript example.
async function getemployeeData() {
try {
const response = await fetch(‘https://jsonplaceholder.typicode.com/users’);
if (!response.ok) {
throw new Error(‘here Network response is not proper’);
}
const data = await response.json(); // it use to Parse the JSON response
console.log(data); // it use to Handle the data
} catch (error) {
console.error(‘it produce a problem with the fetch data operation -‘, error);
}
}
getemployeeData();
Here in this example.
- यहाँ इस प्रोग्राम में getemployeeData() फ़ंक्शन को async के रूप में डिक्लेअर किया गया है, जिसका मतलब है कि यह await का उपयोग इनसाइड में कर सकता है।
- यहाँ await का उपयोग fetch() फंक्शन को कॉल के साथ किया जाता है, जो प्रॉमिस ऑपरेशन सोल्व होने तक फ़ंक्शन को स्टॉप कर देता है।
- जहा .json() मेथड का भी वेट किया जाता है, जिससे यह सुनिश्चित हो सके कि आगे बढ़ने से पहले हमारे पास पार्स किया गया JSON डेटा होता है।
- यह ओवरव्यू .then() और .catch() चेनिंग की तुलना में प्रोग्राम कोड को अधिक रीडेबल बनाता है, स्पेशल्ली, जब आपके पास कई एसिंक्रोनस कॉल मौजूद हों।
Handling errors in fetch() in JavaScript.
यहाँ fetch() ऑपरेशन में, नेटवर्क एरर जैसे, कोई कनेक्शन नहीं या इनवैलिड रेस्पॉन्सेस जैसे, 404 ऑटोमेटिकली रूप से एरर के रूप में नहीं थ्रो की जाती हैं। तो यहाँ प्रोग्रामर को रेस्पॉन्सेस स्टेटस को मैन्युअल टेस्ट करना होगा।
Example of handling errors.
fetch(‘https://jsonplaceholder.typicode.com/users’)
.then(response => {
if (!response.ok) {
throw new Error(‘it Failed to handle fetch data. and Status is – ‘ + response.status);
}
return response.json();
})
.then(data => {
console.log(data);
})
.catch(error => {
console.error(‘it display Error-‘, error);
});
Here in this example above.
- यदि यहाँ रिस्पांस स्टेटस 200-299 रेंज आर्डर में डिस्प्ले नहीं है, तो हम एक एरर को थ्रो करते हैं।
- यहाँ catch() मेथड नेटवर्क प्रोब्लेम्स या इनवैलिड JSON पार्सिंग सहित फ़ेच प्रोसेस के दौरान होने वाली किसी भी एरर को कैच करता है।
Sending headers with fetch() in JavaScript.
यदि आपको अतिरिक्त हेडर ऑथेंटिकेशन, कंटेंट-टाइप, आदि को ऐड करने की जरूरत है, तो आप उन्हें जरूरत के अनुसार हेडर ऑप्शन में इंडीकेट कर सकते हैं।
Sending custom headers in JavaScript example.
fetch(‘https://jsonplaceholder.typicode.com/users’, {
method: ‘GET’,
headers: {
‘Authorization’: ‘Bearer myAccessToken’, // it use to Send an authorization token
‘Accept’: ‘application/json’ // it use to Tell the server we expect JSON in response order
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(‘display Error:’, error)); // it use to handle error
यहाँ इस उदाहरण में, कस्टम हेडर रिक्वेस्ट में मौजूद हैं। हेडर का उपयोग अक्सर रिक्वेस्ट ऑथेंटिकेशन, कंटेंट कम्यूनिकेट, और अन्य पर्पस के लिए किया जाता है।
Handling JSON and other data formats in JavaScript.
यहाँ response.json() मेथड का उपयोग आमतौर पर JSON से रिटर्न होने वाले एपीआई के लिए होता है, आपको अन्य प्रकार की रेस्पॉन्सेस को मैनेज और कण्ट्रोल करने की आवश्यकता हो सकती है, जैसे कि, टेक्स्ट या बाइनरी डेटा फॉर्मेट हो सकता है।
Handling text response in JavaScript example.
fetch(‘https://example.com/some-text’)
.then(response => response.text()) // it use to Parse the response as plain text file format
.then(data => console.log(data)) // it use to Handle the text file data
.catch(error => console.error(‘it display Error -‘, error));
यहाँ इस एक्साम्प्ल में, रेस्पॉन्सेस को सिंपल टेक्स्ट के रूप में पार्स करने के लिए response.text() मेथड का उपयोग किया जाता है।
Summary of getting data using fetch() method function in JavaScript.
- API Basic Syntax – जहा API से JSON डेटा रिसीव करने के लिए जावास्क्रिप्ट प्रोग्रामर fetch(url).then(response => response.json()) मेथड का उपयोग करते है।
- HTTP Methods – यहाँ वेब रिक्वेस्ट में POST, PUT, DELETE, आदि को सेंड करने के लिए इन मेथड का उपयोग करें।
- Error Handling – वेब रिक्वेस्ट में HTTP एरर को मैनेज करने और .catch() फंक्शन का उपयोग करके नेटवर्क प्रोब्लेम्स को कैच करने के लिए हमेशा response.ok को टेस्ट करें।
- Async/Await – जावास्क्रिप्ट प्रोग्राम में अधिक रीडेबल एसिंक्रोनस प्रोग्राम कोड के लिए async/await का उपयोग करें।
- Custom Headers – हेडर ऑप्शन में ऑथेंटिकेशन जैसे हेडर से पास करें।
- Data Format – जरूरी रेस्पॉन्सेस फॉर्मेट के आधार पर response.json(), response.text(), response.blob(), आदि, मेथड फंक्शन का उपयोग करें।