Java basics
If you wish to understand the fundamentals of java programming. So, some java programming language fundamentals are listed below.
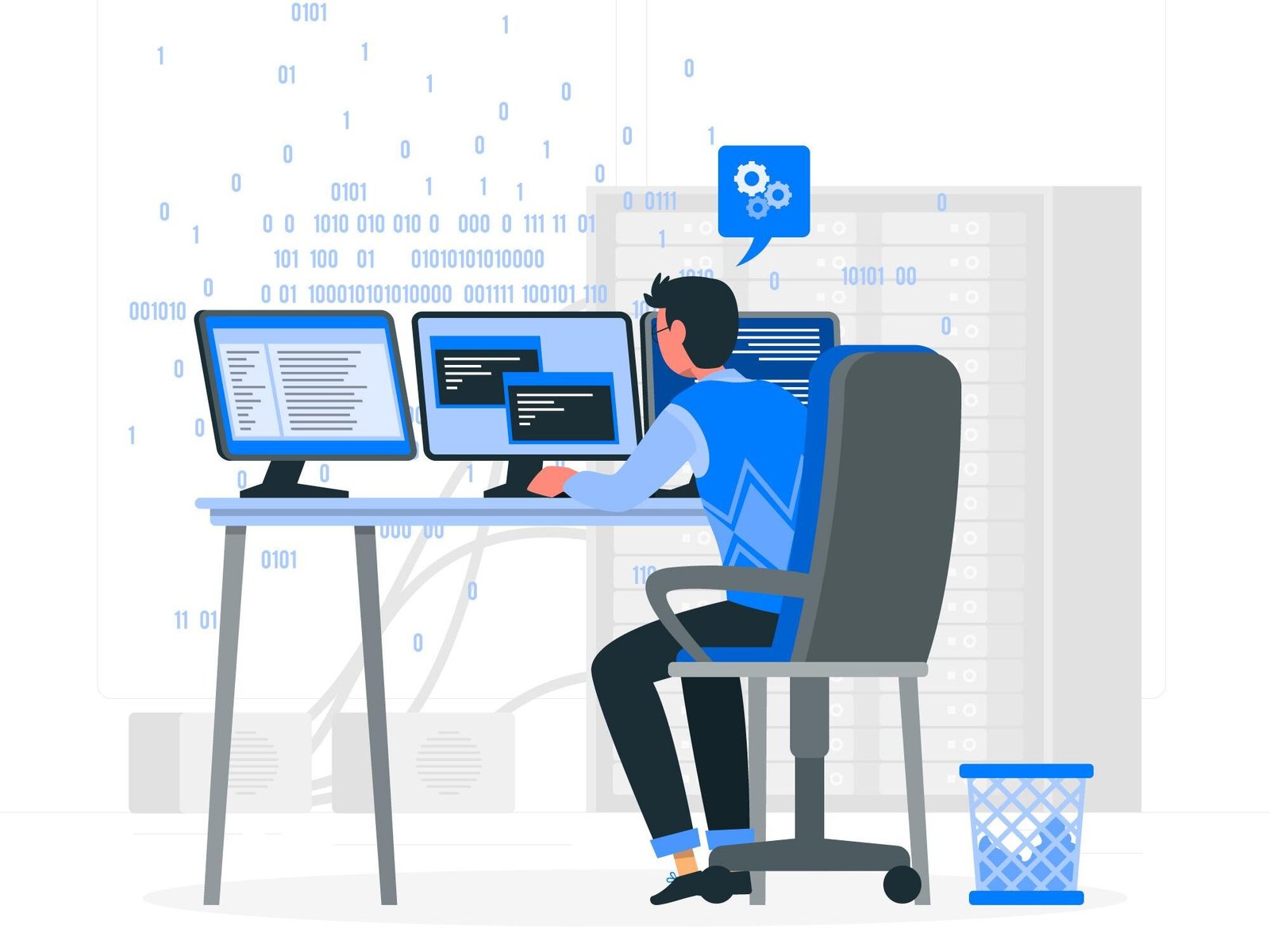
- Syntax – the syntax of java programming is similar to that of c programming. Use a semicolon and curly braces to close the statements to define a block of java program code. Every java program must have a class that serves as the program’s entry point and has a main method.
- Variables – in java programming, variables are defined with a specific kind of data type and can hold many forms of data, including integers, characters, or objects. Before being utilized, variables in java must be defined, and the assignment operator (=) is used to assign values to variables.
- Data types – java programming comes with a wide variety of built-in data types. These include reference types (such as string, array, object, etc.) And built-in types (such as int, double, boolean, etc.). The range of values that may be stored is determined by the specific characteristics of each java data type.
- Operators – java includes a number of operators that may be used to programmatically execute arithmetic, comparison, logic, and assignment operations. Examples include the mathematical operators +, -, *, and /, the comparison operators ==,!=, >, and, the logical operators &&, ||, and!, and the assignment operators =, +=, and -=.
- Control flow – provides information about java control flow. This comprises loops (for, while, do while), branch statements (break, continue, return), and conditional statements (if, else, switch). With the use of these statements, you may modify how a java program executes based on specific circumstances.
- Objects and classes – because java is an object-oriented language, everything is encapsulated within an object. Classes, which are used to specify a blueprint for building an object, include java objects as examples. A specific idea or entity’s data (attributes) and behavior (methods) are contained within classes.
- Methods – methods are specialized portions of code that may be used to run other blocks of code. In java, methods inside a class can be defined and called from other areas of the computer. Parameters (inputs) and return values can both be used in methods.
- Packages – java uses packages to group similar java classes and offer a mechanism for handling name issues. Programmers working in java have access to a standard library of classes divided into several packages. For instance, java.util, java.io, etc. To organize your classes, you may also make your own java packages.
- Exception handling – a built-in mechanism exists in java programming to deal with exceptions. Those are unforeseen occurrences that may occur while a java program is being executed. Try-catch blocks can be used to handle errors and catch them, keeping the program from being terminated suddenly.
- Input/output – when using java programming, you may manage input and output tasks using classes and methods. Using classes like file, filereader, filewriter, etc., you may conduct file input/output operations or receive input from the console using the scanner class.
These are some fundamental java ideas, as was previously explained. You will study increasingly complex subjects as you understand them, including java inheritance, polymorphism, interfaces, generics, and others. You may improve your comprehension of the java programming language by practicing coding, working on simple java projects, and studying java documentation and courses.
Java se development kit
The java se development kit (jdk) is a java programming environment used to create software applications and programs. The tools, libraries, and runtime environment required for program compilation, debugging, and execution are all included in the Java SE Development Kit (jdk).
The following Java development components are made available to Java developers by Jdk.
- Java source code (.java files) – is converted into bytecode (.class files) using the java compiler (javac). Which the Java virtual machine (jvm) is capable of running.
- Java Virtual Machine (JVM) – JVM is in charge of carrying out Java Bytecode execution. Java program memory management, garbage collection, and platform abstraction are all included in the runtime environment it offers for java programs.
- Java Runtime Environment (JRE) – The JRE is a subset of the JDK that contains the Java Virtual Machine (JVM) and necessary class library capabilities. Developmental tools (such as compilers) are excluded from this.
- Tools for development – The JDK comes with a number of Java development tools. Examples include the Java Development Kit (javak), Java Archive Tool (jar), Java Debugger (jdb), and others. These tools are used for java program compilation, packaging, and debugging.
- Class libraries – The jdk gives Java developers access to a number of common class libraries. Sometimes referred to as the Java API (application programming interface). These libraries contain Java methods and predefined classes. This is useful for creating Java apps. Include functions including file IO, networking, GUI creation, database integration, and more.
When you install and download JDK. You now have access to every piece of hardware and equipment needed to create Java applications. On your computer, you can write, build, and run Java code. Jdk is available in a number of downloadable versions. Each is associated with a certain java se release, such to jdk 11, jdk 17, etc.
Never forget that jdk is often used by java software developers to create java applications. If all you want to do is execute Java programs. The java runtime environment (jre), which is a subset of the jdk and provides the components needed to run java programs, may thus be all you need.
Java compile online
There are several platforms available right now for running Java programs online. Which enables direct Java program code compilation and execution from your web browser.
Some well-known platforms for running Java programs are shown below.
- Jdoodle – Available at https://www.jdoodle.com Jdoodle is an online editor and compiler for Java programs. It permits the use of many different programming languages, including Java, online. It offers a straightforward programming interface. Where you may write and run the Java source code. Additionally, you may enter command-line parameters into a Java program and test your code using various inputs.
- Replit https://replit.com/ – Replit is an internet platform for program coding (https://replit.com/). It offers many more programming development characteristics in addition to a Java program compiler. It offers a java integrated development environment (ide), allowing you to create, compile, and run java programs as needed. Additionally, you may work together with other Java developers, exchange the source code of your Java programs, and investigate projects made by the Java community at large.
- Onlinegdb https://www.onlinegdb.com/ – is a web-based integrated development environment (IDE). This encourages the development of several other computer languages, including Java. You can use your web browser to create Java program code, build Java programs, and run Java programs. In this case, the java program platform additionally offers debugging options and an input/output console.
- Paiza.io https://paiza.io/en/ – Java compiler and IDE are available through the online development environment paiza.io. You may create your Java program code in the provided editor, which offers features like code completion and syntax highlighting for Java programs. Within the platform, your Java code may be compiled, executed, and even shared.
Keep in mind that you may easily test and run Java program source code on various platforms without having to set up a local development environment. But if you’re working on substantial Java projects or want sophisticated Java programming capabilities. Therefore, it is advised to utilize a local Java programming environment on your PC, such as Eclipse, intellij Idea, or Netbeans.
Online compiler java
List of java online program run platform.
- Compilejava – https://www.compilejava.net/
- Coding ground – https://www.tutorialspoint.com/execute_java_online.php
- Paiza.io – https://paiza.io/en/projects/new?Language=java
- Onlinegdb – https://www.onlinegdb.com/online_java_compiler
- Replit – https://replit.com/languages/java
- Jdoodle – https://www.jdoodle.com/compiler/java
What is java se
Java se It stands for Java Standard Edition. It is a platform for development that offers the fundamental tools and libraries needed to create and execute Java program applications. The broader Java program development kit (jdk) includes Java SE. It offers tools for java program compilation, debugging, and packaging.
The drug developer is given a runtime environment (jre) via Java SE. This makes it possible for Java programs to run on a variety of hardware, software, and operating systems. The Java virtual machine (jvm) is a part of this. It consists of a collection of Java standard libraries and apis (application programming interfaces) and is in charge of running Java bytecode. It offers the Java developer capability for activities including input/output, networking, database access, and user interface creation.
Java programs can run on any platform since Java itself is intended to be platform-independent of all current hardware. It has a Java runtime environment that is compatible. By converting java source code into platform-independent bytecode, the ability to “write a java program once, run it anywhere in a compatible java environment” is realized. It can operate on any system equipped with the necessary Java runtime environment.
The core of Java programming, Java se, is utilized in a broad variety of applications. It also has additional features, desktop software, business systems, web applications, and mobile applications. It offers an extensive selection of libraries, frameworks, and tools in a mature and powerful environment. Which programmers may use to create dependable and scalable Java applications.
Java version 17
The release of Java SE 17 is referred to as Java version 17. It is a significant update to Java Standard Edition. The java programming language and platform received a number of new features, upgrades, and speed boosts with the introduction of java se 17 on September 14, 2021.
Here are some of the top improvements and new features included in Java SE 17.
- Sealed classes – Java software developers can regulate which Java classes can be subclasses of a certain class by using sealed classes. This offers inheritance greater power.
- Pattern matching for Switch – This feature adds pattern-matching capabilities to Java’s switch statement, enhancing its functionality. Hence enabling shorter, more expressive code.
- Improved pseudo-random number generator – Java SE 17 adds new implementations and interfaces for pseudo-random number creation. Which improves the security and performance of Java programs.
- Greater encapsulation of jdk internals – this improvement increases the encapsulation of internal java development kit (jdk) apis to increase security and maintainability.
- Foreign function and memory api (incubator) – This module belongs to the incubator class. It offers a common method of interfacing with native Java code and memory. Resulting in improved compatibility with native libraries.
These are some of the standout new additions and enhancements included in Java SE 17. To increase developer productivity, efficiency, and security, every Java version generally includes a combination of programming language changes, java libraries, updates, and new Java platform improvements.
Java 17 jdk
The java software development kit, or jdk, version 17 is referred to. Contains the Java program libraries and tools needed to construct Java SE 17 software applications, compile Java programs, and run Java programs. For Java developers to write Java code, they need Jdk tools. Make the java program execute on the java virtual machine (jvm) by compiling the java code to bytecode.
Java SE 17 is the current version. This refers to the Java programming language’s most recent major version. The Java Development Kit (JDK) and Java Runtime Environment (JRE) features are included in Java SE 17 and are used to create and run Java software applications. It offers the fundamental Java tools, libraries, and capabilities needed to create Java programs.
Java se downloads
Download Java SE (standard edition) if you’d like. These are some of your main possibilities, then.
Download link for the Oracle Java SE version (official).
- You may get Java SE from the official Oracle website. You may accomplish this by going to the Oracle Java SE Download Page.
Https://www.oracle.com/java/technologies/javase-jdk17-downloads.html
For a variety of contemporary computer operating systems, you may find the most recent jdk (java development kit) and jre (java runtime environment) releases here.
- Openjdk for Java is advised.
An open-source implementation of Java SE is called Openjdk. From the following websites, you may obtain openjdk software distributions here.
- Adoptopenjdk – https://adoptopenjdk.net/
- Builds openjdk – https://jdk.java.net/
These websites offer a selection of openjdk software distributions for different platforms and Java SE versions.
- Possibilities for vendor-specific Java distribution.
Many vendors offering their own distributions of Java SE may be found here.
Here are a few well-liked choices.
- Amazon corretto – https://aws.amazon.com/corretto/
- Azul zulu – https://www.azul.com/downloads/zulu-community/
- Red hat openjdk – https://developers.redhat.com/products/openjdk/download
All of these jdk distributions are built on the openjdk foundation and may include extra Java development tools, assistance, or enhancements tailored to the platform or environment of the vendor.
Make careful you choose the correct jdk version and distribution for your operating system when downloading Java SE from this page. In order to create Java applications. Therefore, pick jdk, or pick jre if you simply need to run Java programs.
Java se download
Download Java SE (standard edition) if you’d like. You may mostly follow these instructions.
- At first, you visit the download page for Oracle Java SE.
- Https://www.oracle.com/java/technologies/javase-jdk17-downloads.html
- Go to the “java se development kit 17 downloads” section by scrolling down.
- Decide which Java Development Kit (JDK) download is best for the operating system on your machine. Operating systems like Windows, macos, and Linux are frequently available choices.
- Read the Oracle Technology Networks License Agreement for Oracle Java SE, and accept it. To accept all the terms, tick the box.
- Now select the jdk distribution download link that corresponds to your operating system.
- You might now be requested to log in using an Oracle account. You can open a free Oracle account if you don’t already have one.
- The download should start instantly when you log into your Oracle account. If it doesn’t, try clicking the relevant Oracle download link once more.
- Find the downloaded file on your PC when the Oracle JDK version download has finished. The Java SE file in this instance will be in an executable installation format that is unique to your operating system.
- Run the downloaded installer and follow the java se installation instructions on-screen to install the java se development kit.
You will have access to the java compiler (javach), the java runtime environment (java), and other development tools offered by the jdk once you have successfully installed the java se development kit on your machine. The creation and execution of Java programs both need the use of these technologies.
Don’t forget to choose the proper JDK distribution and version for your operating system. Alternative Java SE distributions are also available. Like openjdk, which, based on your particular requirements and preferences, you may consider.
Learn java online
- Oracle java tutorials.
Https://docs.oracle.com/en/java/javase/index.html
- Java documentation and api.
Https://docs.oracle.com/en/java/javase/index.html
Java for beginners
If you’ve never programmed in Java before, if you want to learn, and if you’re entirely committed to learning, then read on.
A proposed learning route for Java beginners is provided below.
• Understanding fundamentals.
Start your java programming education by being familiar with the fundamental ideas of programming, such as variables, data types, operators, control structures (if statements, loops), and functions. To learn Java, you can consult online tutorials or beginner programming classes. They provide language-neutral coverage of java programming fundamentals.
- Create a java development environment.
On your laptop, install the Java Development Kit (jdk) program. It also contains the java compiler and other tools essential for creating java programs. You can pick an alternate java development distribution, such openjdk, or you can get the jdk version straight from the Oracle java se download website.
- Become familiar with the Java syntax and features.
If you have experience with the c or c++ programming languages, study the grammar of java. Therefore, learning Java, including how to declare java variables, construct java program loops, conditions, and functions (methods), as well as how to work with java arrays and collections, will be simple for you. Be familiar with Java’s object-oriented programming concepts, including classes, objects, inheritance, polymorphism, etc.
- Exercise your coding skills.
You may strengthen your understanding of Java principles by working through java coding exercises and Java-based programming difficulties. Java coding practice is available on websites like codingbat, leetcode, or hackerrank for newcomers.
- Get familiar with Java apis and libraries.
Investigate the most popular Java libraries and apis. A good example of this is the Java collections framework, which offers data structures like lists, sets, and maps. Know how to utilize Java libraries for working with dates and times, managing files, and performing input/output tasks.
- Create basic Java programs.
To put your skills to use, start by creating simple Java programs and apps. Make a little Java calculator, a text-based game, or a program that reads and writes Java files, for example.
- Learn the fundamentals of object-oriented programming (oop).
Learn about classes, objects, inheritance, polymorphism, and encapsulation to better understand object-oriented programming. These ideas are essential to the Java programming language and are crucial for creating larger, more sophisticated Java program development apps.
- Put project-based learning into action.
Work on brief assignments that incorporate various Java principles. You may use what you’ve learned in this way to develop whole applications and get experience.
- Look at Java tools and frameworks.
Once you’ve mastered the fundamental ideas of Java. So, you may investigate well-known Java frameworks and technologies like maven, spring, hibernate, or javafx. These frameworks can help you become a better Java developer and let you produce more complex Java software.
- Continue your practice and studying.
By tackling code problems, reading additional java books, taking online classes, and investigating increasingly complex subjects like multithreading, networking, database connection, and java design patterns, you may keep learning and practicing java programming.
The secret to learning java programming is continual practice and actual code. Be patient and persistent while you learn java, and never be afraid to ask for assistance from online forums or communities when you run into java programming problems.
Oracle java jdk
Java SE development kit officially given by Oracle Corporation is referred to as Oracle jdk (java development kit). It includes the programs and libraries needed to create, put together, and operate Java programs.
You will find some information and features regarding the Oracle JDK Platform Edition right here.
- Licensing – Oracle has switched to a new licensing approach as in java version 11. The Oracle JDK is now offered with two different licenses. One for corporate usage and the other for individual and development use. Oracle also offers long-term support (lts) versions. That has access to updates and security fixes for a long time.
- Features and components – Java Development Kit features are included in the Oracle JDK’s features and components. It consists of the Java runtime environment (java), the Java compiler (javac), and a number of tools and capabilities for creating Java programs. The Java api documentation and the Javafx framework for creating advanced graphical user interfaces (guis) are also included.
- Platform support – Oracle JDK is accessible on a wide range of platforms. Windows, Mac OS, and different linux-based distributions are all included in this. The requirements and installation procedures for the jdk vary depending on the platform.
- Versions and updates – The Oracle JDK offers a variety of versions that match various Java SE editions. These versions come with multiple additional capabilities in addition to Java SE 8, Java SE 11 (LTS), and Java SE 17 (LTS). For versions of the Oracle JDK that are supported, Oracle offers updates and bug fixes.
- Download and installation – The Oracle jdk may be downloaded from the Oracle website’s Oracle Java SE Downloads page. Your operating system will determine the installation procedure. In order to configure jdk properly, you might need to define environment variables like java_home and path.
While the Oracle JDK is an official distribution of Oracle, it is vital to remember this. Alternative distributions are furthermore offered. One example is openjdk, which offers a version of the Java Development Kit based on the open-source openjdk project. These substitute distributions offer features comparable to those of the Oracle JDK. License conditions and support choices, however, might change.
It is advised that you read and understand the licensing conditions and choose the right distribution depending on your needs and use case before downloading and utilizing the Oracle JDK.
Java 11 jdk
The software set made available by Oracle Corporation is known as Java 11 JDK (java development kit). Contains the programs and libraries needed to create Java applications that target Java SE 11 and to build and run Java programs.
Here are a few important details concerning the Java 11 JDK.
- Features – When compared to earlier versions, Java 11 contains a lot of new features, upgrades, and advancements. Java 11 has a number of significant features, including.
- The usage of var in the lambda expression parameters is made possible by the local variable syntax for lambda parameters feature. As a result, the Java program code is shorter.
- Httpclient (standard) – A new httpclient api function is included in Java 11 as a standard feature. It offers a more advanced and adaptable technique to manage replies to http client queries.
- Launch programs that use a single file of source code – Java 11 enables the direct execution of a single java source file without the need to manually compile it to bytecode.
- Epsilon garbage collector – Epsilon is a fresh experimental garbage collector that doesn’t really do memory reclamation, which may be advantageous in some circumstances.
- Long-term support (lts) – Java 11 is a long-term support (lts) release, meaning it will continue to get security updates and fixes for a longer length of time than non-lts versions. It is typically advised to utilize the lts release for business and production purposes.
- Platform support – Java 11 JDK is accessible on a variety of operating systems. That covers several Linux operating system distributions, Windows, and Mac OS. There are particular installation instructions and prerequisites for each operating system platform.
- Oracle jdk vs openjdk – Oracle offers both the Oracle JDK and the openjdk releases. Additional features and tools in the Oracle JDK, such business support, may be helpful in corporate environments. On the other hand, Openjdk is an open-source Java version of java se and offers features comparable to those of Oracle jdk. Though without paid Java support.
- Get and installation – To get the java 11 jdk, go to either the openjdk or the oracle java se download page. Choose the proper jdk distribution version for your operating system, download the installer or archive file, and then adhere to Oracle’s or the openjdk community’s installation instructions.
Make sure your development environment and tools are compatible with Java 11 before utilizing the java 11 jdk. To find out about any specific modifications or concepts added to Java 11, it is also advised to read the documentation supplied by Oracle or the openjdk community as well as the release notes.
Openjdk versions
The open-source Java platform implementation known as Openjdk has issued several updates throughout time. The most common openjdk versions are listed below.
- Openjdk 6 – Openjdk 6 is the open-source version of java SE 6 implementation. Up to the introduction of Java 7, it was frequently utilized. But it is no longer actively supported or updated.
- Openjdk 7 – this version offered the open-source Java SE 7 implementation. It provided a number of new features, such as try-with-resources, switch statements with strings, and enhanced concurrency tools.
- Openjdk 8 – The open-source version of Java SE 8 was called openjdk 8. Lambda expressions, the stream api, default methods in interfaces, and the new date and time api are just a some of the key improvements it provided.
- Openjdk 9 – With the introduction of the Java Platform Module System (jpms), Openjdk 9 introduced modularization. It enabled programmers to construct modules for applications that are more scalable and effective. Other additions in this version included the addition of the jshell tool and upgrades to the trash collector.
- Openjdk 10 – Compared to other major versions, openjdk 10 included fewer new features while still maintaining Java’s development. It included capabilities like as local-variable type inference (var) and enhanced garbage collectors.
- Openjdk 11 – As the first long-term support (lts) release under the new java release cycle, openjdk 11 represented an important milestone. The elimination of outdated apis, enhancements to the garbage collectors, and support for the http client api were just a few of the modifications and improvements it contained.
- Openjdk 12, 13, 14, 15, 16, 17 – Openjdk versions 12, 13, 14, 15, and 16 followed Java’s regular release cycle while introducing small enhancements and new capabilities. A variety of improvements and bug fixes were added to each edition.
Versions of Openjdk are normally in line with comparable Java SE versions, however there may be very minor variations between distributions offered by various manufacturers. To learn about the features, updates, and compatibility with your development environment of each openjdk version, it’s crucial to read the relevant release notes and documentation for that version.
Openjdk distributions are available for download via the openjdk website (https://openjdk.java.net/) or through companies like adoptopenjdk, azul zulu, and red hat openjdk that provide pre-built binaries based on openjdk.
Java development kit download
Java development kit (jdk), which you may download to your PC. You may thus largely follow the instructions listed below.
- At first, you visit the download page for Oracle Java SE.
- Https://www.oracle.com/java/technologies/javase-jdk17-downloads.html
- Go to the “java se development kit 17 downloads” section by scrolling down.
- Read the Oracle Technology Networks License Agreement for Oracle Java SE, and accept it. To accept the conditions, tick the box.
- Choose the correct jdk download for the operating system that is currently running on your machine. Operating system features for Windows, macos, and Linux are frequently available as selectable options.
- Right now, be certain to choose the jdk version you wish to download to your machine (for instance, jdk 17).
- Click the download link for the version of the jdk distribution that matches your operating system.
- You might be prompted to sign in with your Oracle account at this point. You can open a free Oracle account if you don’t already have one.
- The jdk download link needs to launch instantly when you log into your Oracle account. If not, try clicking on the relevant download link once again.
- Find the downloaded file on your PC when the Oracle JDK version download has finished. The download will be in an executable installation format that is unique to your operating system.
- Start the installer to begin installing the Java Development Kit, then follow to the on-screen directions.
The java compiler (javac), java runtime environment (java), and other development tools offered by the jdk will be available to you once the jdk software has been successfully installed. In order to create and run Java programs, several tools are necessary.
Lastly, don’t forget to choose the jdk distribution and version that are most suited to your operating system. Alternative JDK distributions are available as well. According to your own demands and preferences, you could consider options like openjdk.
Jdk java
Java development kit, or Jdk. It is a set of software that Oracle Corporation offers. Contains the development, compilation, and running resources and library features for Java programs.
Oracle jdk
The term “Oracle jdk” (java development kit) refers to the company’s official distribution of the Java Development Kit. It is a complete set of software. The runtime environment, libraries, and tools needed to create, build, and run Java programs are all included.
Java programming
Writing Java program code, compiling programs, and executing Java programs are all steps in the process of developing Java software applications or systems. The general-purpose programming language Java is quite popular. It is well known for its platform independence, adaptability, and simplicity in programming and software creation.
Java 11 download
Java se development kit 11 (jdk 11) download for your pc.
You may mostly follow these instructions.
- At first, you visit the download page for Oracle Java SE. Https://www.oracle.com/java/technologies/javase-jdk11-downloads.html
- Go to the “java se development kit 11 downloads” section by scrolling down.
- Read the Oracle Technology Networks License Agreement for Oracle Java SE, and accept it. To accept the conditions, tick the box.
- Choose the correct jdk download version for your computer’s operating system. Windows, Mac OS, and Linux are frequently offered as options.
- Right now, be careful to choose the jdk version you wish to download (for instance, jdk 11).
- Select the jdk distribution software download link that corresponds to your operating system.
- An Oracle account sign-in request could be made. You can open a free Oracle account if you don’t already have one.
- The download should start instantly when you log into your Oracle account. If not, try clicking on the relevant download link once again.
- Find the downloaded file on your PC when the Oracle JDK version download has finished. The download will be in an executable installation format that is unique to your operating system.
- To install JDK 11, launch the Oracle JDK installer and follow to all of the on-screen directions.
You will have access to the java runtime environment (java), the java compiler (javac), and other development tools after successfully installing jdk 11. When using JDK 11, these tools are necessary for both creating and running Java programs.
It is significant to remember that there are other distributions outside Oracle JDK, such as openjdk. Which offer the JDK 11 build. These substitute distributions offer features comparable to those of the Oracle JDK. However, there could be several license conditions and support choices.
Java language
Java is a high-level, general-purpose computer programming language that was created in the middle of the 1990s by Sun Microsystems, which is now owned by Oracle Corporation. Java programming is widely recognized for its ease of use, freedom from platforms, and wide support for java libraries.
Java download windows 11
You may primarily follow these instructions to obtain Java programs for Windows 11.
- To begin with, go to the Oracle Java SE download page at https://www.oracle.com/java/technologies/javase-jdk11-downloads.html.
- Go to the “java se development kit 11 downloads” section by scrolling down.
- Read the Oracle Technology Networks License Agreement for Oracle Java SE, and accept it. To accept the conditions of the software license, tick the box.
- Choose the correct Java Development Kit (JDK) download for Windows 11. Make sure to select the appropriate version (32-bit or 64-bit) and installation type (exe or zip) for your Windows system architecture.
- Select the Windows jdk distribution download link that best suits your requirements.
- An Oracle account sign-in request could be made. You may make a free account if you don’t already have one.
- The download should begin instantly after logging in. If not, try clicking on the relevant download link once again.
- Find the downloaded file on your computer when the download is finished. If you downloaded an executable file. Therefore, click it twice to launch the installation. Extract the contents of the zip file you downloaded to the proper place on your computer.
- To install JDK 11 on your Windows 11 PC, follow the on-screen instructions. You might be prompted to specify environment variables and select an installation directory. Similar to java_home and path, which are crucial for appropriately configuring java.
You will have access to the java compiler (javac), java runtime environment (java), and other jdk-provided development tools once you have successfully installed jdk 11 on your Windows 11 PC. When using JDK 11, these tools are necessary for both creating and running Java programs.
Please be aware that Windows 11 is a relatively new operating system and that support and updates from Oracle and other suppliers, including Java, may or may not be available at the time of use. Make careful to monitor the Oracle website for any changes or announcements about the compatibility of Java with Windows 11.
Download java windows 10
Java may be downloaded for Windows 10 if you wish to. You can consequently follow the instructions below.
- To begin with, go to the Oracle Java SE download page at https://www.oracle.com/java/technologies/javase-jdk11-downloads.html.
- Go to the “java se development kit 11 downloads” section by scrolling down.
- Read the Oracle Technology Networks license Agreement for Oracle Java SE, and accept it. To accept the conditions, tick the box.
- Choose the suitable Windows 10 jdk download. Make sure to select the appropriate version for the architecture of your existing operating system (32-bit or 64-bit) and the installation type you want (exe or zip).
- Choose the jdk distribution’s download URL that best suits your needs.
- An Oracle account sign-in request could be made. You can establish a free Oracle user account if you don’t already have one.
- The download should to start instantly when you log into your Oracle account. If not, try clicking on the relevant download link once again.
- Find the downloaded file on your computer when the download is finished. To launch the installer if you downloaded the exe file, double-click on it. Extract the contents of the zip file you downloaded to the proper place on your computer.
- To install JDK 11 on your Windows 10 PC, follow the on-screen instructions. You might be prompted to specify environment variables and select an installation directory. Similar to java_home and path, which are crucial for appropriately configuring java.
You will have access to the java compiler (javac), java runtime environment (java), and other jdk-provided development tools once you have successfully installed jdk 11 on your Windows 10 operating system. When using JDK 11, these tools are necessary for both creating and running Java programs.
Please be aware that Java works well with Windows 10 because it is a commonly used operating system. To check for any particular changes or announcements about java and windows 10 compatibility, it is always a good idea to visit the Oracle website.
Open jdk download
If you wish to get the Java version of the openjdk. Therefore, you may mostly follow the instructions below.
- Visit the adoptopenjdk webpage at https://adoptopenjdk.net/ at the beginning.
- A list of the many Java distributions and versions is accessible right here on the main page. Choose the openjdk version you wish to download to your computer. You can select from the several variants. Such include Java 8, Java 11, Java 17, and several others.
- Following your choice of version, you will be given alternatives based on your computer’s operating system and architecture. Select the one that best suits your system. For instance, you can select the Windows x64 version if you’re using Windows 10.
- You will be brought to a download page after choosing the proper version and distribution. You could also have the choice of selecting a jvm vendor or package type on this page. Click the “latest release” or “download” button after selecting the one you want.
- Here, an automated download for the Java program will begin. Depending on the distribution and version you choose, the file size and download time may change.
- Find the downloaded file on your PC when the openjdk file download is complete.
- If you downloaded an executable installation for Windows (like a.msi file). In order to install openjdk, double-click on the downloaded file and follow the on-screen directions. Extract the contents of any zip files you’ve downloaded to the proper location on your computer.
- If you downloaded a.dmg file and are a mac user. Therefore, double-click on it and follow to the prompts on the screen to install openjdk.
- If you downloaded a tar.gz file and are a Linux user. Extraction of its contents should then be done to the specified computer location. For installation instructions unique to your Linux distribution, consult the documentation included with the distribution.
The java development kit (jdk) is available once the openjdk program has been set up on your machine. Included in this are the openjdk-provided tools and libraries, the java compiler (javac), the java runtime environment (java), and more. When using openjdk to create and run Java programs, these tools are necessary.
Please be aware that openjdk is a free and open-source alternative to Oracle jdk for developing applications. Many different groups and organizations maintain it. A well-known openjdk distribution is the adoptopenjdk effort, which was previously discussed. It offers options for prebuilt download binaries for a variety of systems.