var, let, and const explained
In JavaScript web development script language, var, let and const data types are used to declare program variable data type. But in existing web development scripts, declared variable scope, reassignment, and use cases of variables may vary. JavaScript web developer must understand it better to write better and clean program source code.
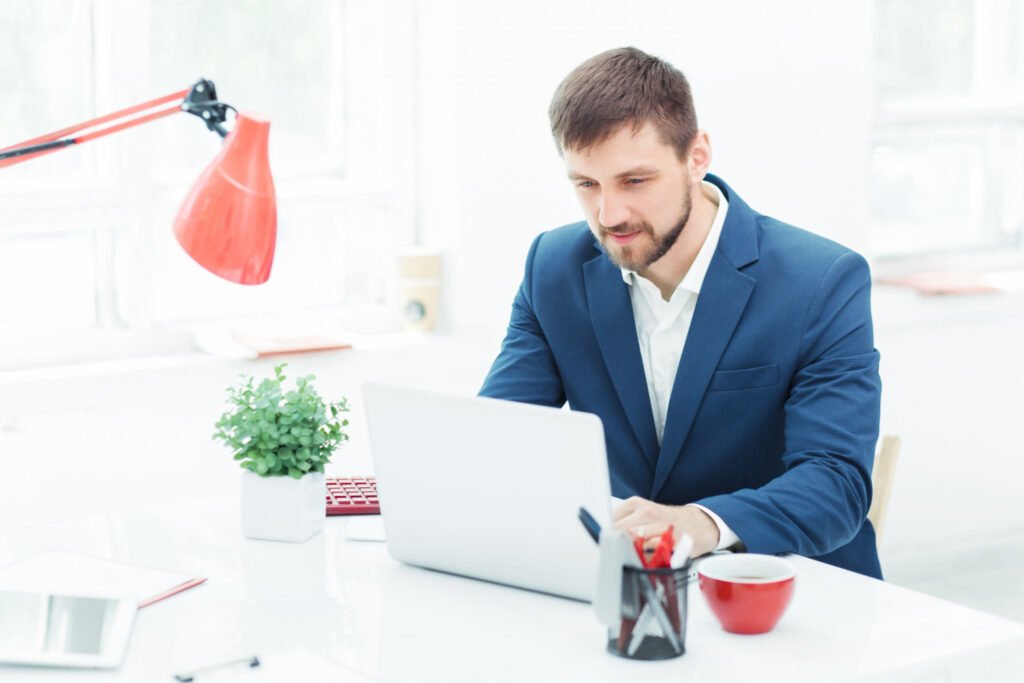
var variable data type.
var data type is one of the oldest ways to declare variables in JavaScript programming. var data type was introduced in JavaScript language in ES5 and earlier. var data type behaves a little differently from existing JavaScript data types. Which can sometimes produce you some different or unexpected results. Therefore, currently let or const data type variables are used in modern JavaScript web development language.
Main features of var data type in JavaScript.
- Function-scoped – In a JavaScript program, if the var data type is declared inside a function, then it is compatible only inside that current function. But, if it is declared outside a function, then the scope of this var data type variable becomes global.
- Can be redeclared – You can redeclare the same var data type variable multiple times within the same program scope.
- Hoisted – Variables declared with var data type appear at the top of their scope, which means they are accessible from anywhere in the program before their declaration, but they are initialized at the declaration.
var data type example in JavaScript programming.
var comp_name = “Vcanhelpsu”;
console.log(comp_name); // result is – Vcanhelpsu
var compname = “Vcandevelop”; // Here Redeclaring ‘compname’ is allowed with ‘var’variable data type
console.log(compname); // result is – vcandevelop
function sampleVar() {
var programming = “Javascript”;
console.log(programming); // result is – javascript
}
sampleVar();
console.log(programming); // Display Error: ‘programming’ is not defined (due to function scope)
Aspects of var data type usage.
- Redeclaration – As in JavaScript programming language, var data type variable allows multiple re-declarations. So sometimes it can create conflict or confusion in a large programming project codebase.
- Hoisting behavior – Due to hoisting features in JavaScript program, the variable declared with var data type variable can be accessed anytime before its declaration. Due to which you can get many unexpected results in the program output.
let Variable Data Type in JavaScript.
let data type variable was first introduced in JavaScript in ES6 ECMAScript 2015, and it is currently a modern method of declaring JavaScript variable data type. In JavaScript language, let data type is more compatible and accessible than var data type variable, where many problems can develop in the behavior of let data type var.
Key features of let data type in JavaScript.
- Block-scoped – Let program blocks in JavaScript programs are limited to the source code code enclosed by curly braces {}, not just the current program function where it is declared. This means that a variable declared with let data type is accessible only inside the program block in which it was declared by the JavaScript programmer.
- Reassignable – Let-declared variables in JavaScript programs can be reassigned values.
- Cannot be redeclared within the same scope – Unlike var variable data type declaration, you cannot re-declare the same variable inside the same current program block or function using let data type.
- Hoisting – Variables declared with let data type in JavaScript programs are hoisted to the top location of the block, but remember, they are not initialized until the program code execution reaches the let statement. Where programmers cannot access the variable before the variable declaration.
Example of let data type in JavaScript programming.
let compname = “Vcanhelpsu”;
console.log(compname); // result is – vcanhelpsu
compname = “Vcandevelop”; // Reassigning ‘compname’ is allowed in let data type
console.log(compname); // result is – vcandevelop
if (true) {
let programming = “Javascript”;
console.log(programming); // result is – Javascript
}
console.log(programming); // Display Error – ‘programming’ is not defined (due to block scope)
Advantages of let data type in JavaScript.
- Block scoping – Program variables declared in let data type are limited to the block in which they are declared. For example, inside a program loop or conditional, let data type prevents sudden overwriting processes and activities related to the scope.
- No re-declarations within the same scope – Let data type helps in reducing bugs related to accidentally re-declaring variables in a program.
const Data Type in JavaScript.
const data type in JavaScript program was also introduced for the first time in ES6, and const data type is used to declare constant variable data type in JavaScript. const data types are variables that should not be reassigned or modified once they are declared in a program.
Key features of const data type in JavaScript.
- Block-scoped – Just like let data type in JavaScript, const data type variables are limited to the same block, not the current program function.
- Unable to be reassigned – Once a variable is declared or assigned with const in a JavaScript program, the value of that const variable cannot be re-assigned.
- Unable to be redeclared – You cannot redeclare a const variable in the same scope in the current program.
- Hoisting – Const data type variables are hoisted in JavaScript programs, but there is a “temporal dead zone” present in JavaScript just like let data type, so you cannot access it before declaration in JavaScript program.
Examples of const data type in JavaScript.
const stud_name = “David”;
console.log(stud_name); // result is – david
stud_name = “Lalit”; // Display Error – New Value Assignment to constant variable
Working with Objects or Arrays with Const in JavaScript.
Const in JavaScript program prevents variables from automatically reassigning themselves, but it does not freeze the contents of the object or array in the current program. So you can still modify the properties or elements of the object or array declared with const as per your program development needs.
const employee = { emp_name: “Rock”, emp_age: 43 };
employee.age = 47; // This is allow mutating the object
console.log(employee.age); // the result is – 47
employee = { empname: “David” }; // Display Error – Assignment to constant variable
Example With Array.
const Course = [“Javascript”, “Css”, “Java”, “Html”];
Course.push(“Ruby”); // This is allowed mutating the array
console.log(Course); // resutl is – [ ‘Javascript’, ‘Css’, ‘Java’, ‘Html’, ‘Ruby’ ]
Course = [“Python”, “Java”]; // Error: Assignment to constant variable
Advantages of const data type in JavaScript.
- Immutability (reassignment) – const data type variable enforces the rule that once a const data type variable is declared or set in a program, it should not be modified again.
- Predictability – It reduces the program error probability due to sudden variable reassignment in the current program.
Var, let and const data type Comparison Summary.
Feature | Var data type | Let data type | Const data type |
Scope | Only Function-scoped | Only Block-scoped | Only Block-scoped |
Redeclaration | Var variable Allowed within the same scope | Let variable Not allowed within the same scope | Const variable Not allowed within the same scope |
Reassignment | Var variable Allowed | Let variable Allowed | Const variable Not allowed (constant) |
Hoisting | Var data type Hoisted to the top, initialized as undefined | Let data type Hoisted, but not initialized temporal dead zone | Const data type Hoisted, but not initialized temporal dead zone |
Uses of Multiple Data Types in JavaScript Conclusion.
- When the value of a variable will be modified in a JavaScript program, then you should use let data type.
- You should use const data type variables in JavaScript programs when the value of a variable should not change. For example, constants, or when referring to an object/array where the declared contents can be modified.
- Var data type is currently not used in JavaScript programming. This is because it can have several usage issues with scope and hoisting, which can create bugs or errors in a program.