Template literals for string interpolation
Template literals are powerful attributes or features in JavaScript programming that allow JavaScript programmers to perform string tasks in a more readable and flexible order. JavaScript template literals make string manipulation easier, especially when JavaScript programmers need to add variables or advanced program expressions inside strings.
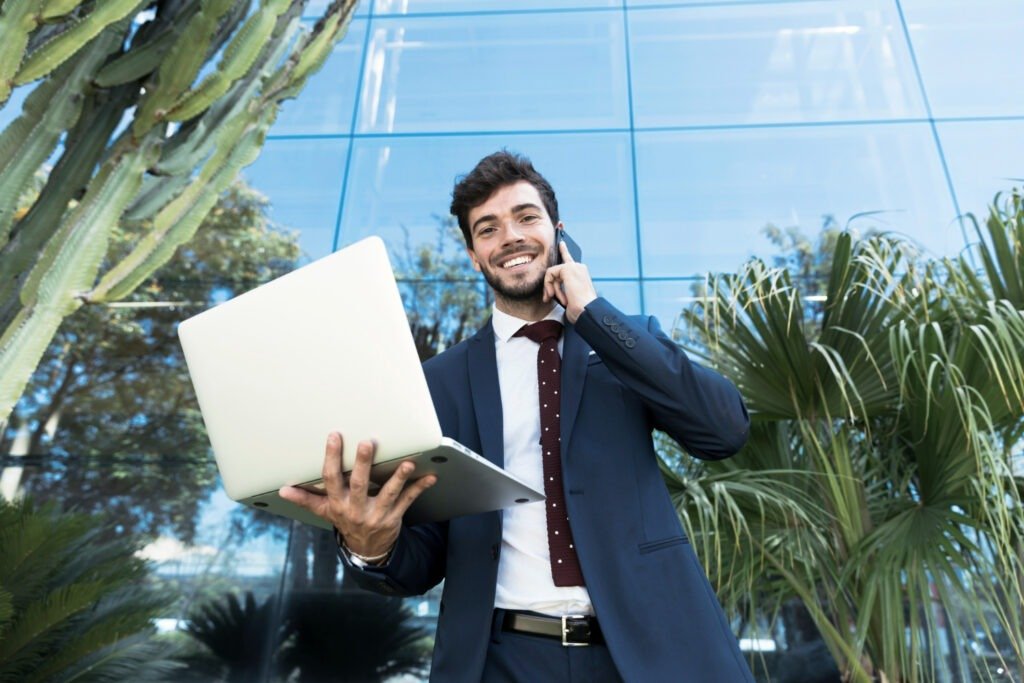
JavaScript template literal syntax.
Template literals in JavaScript programs are enclosed by backticks (`), and programmers can manually insert variables or expressions inside ${}.
`Test, ${expression}!`
Basic string interpolation in JavaScript.
Instead of concatenating strings by applying the + operator in a JavaScript program, you can apply template literals to insert variables directly into a string data type variable.
Example of interpolation in JavaScript.
let programming = “Javascript”;
let price = 999;
let text = `Welcome, to ${programming} and course price is – ${price}`;
console.log(text); // result is – Welcome, to Javascript and course price is – 999
Here, ${programming} and ${price} are two placeholder variables in the current program, which are replaced by the programming and price variable values in the current program.
Expression interpolation in JavaScript.
Javascript programmers can manually insert any valid program expression inside ${} along with program variables. It contains program calculations, user-defined function calls, and many other features.
Example of expression interpolation in JavaScript.
let p = 1;
let q = 2;
let total = `${p} + ${q} = ${p + q}`;
console.log(total); // result is – 1 + 2 = 3
In the above program, the program expression here contains the evaluated 3 results of ${p + q}, and the program result is previewed in the string.
Multi-line strings in JavaScript.
Template literals in JavaScript programs allow you to easily create multi-line strings without applying escape sequence characters such as \n.
Example of multi-line strings in JavaScript.
let text = `javascript is a popular programming
which is most used in web development
it enable us to do all web design and development task easier`;
console.log(text);
result is –
javascript is a popular programming
which is most used in web development
it enable us to do all web design and development task easier
With template literals in this program, new lines are secured, making it much easier to deal with multi-line string variable elements in the current program.
Embedding expressions in JavaScript.
JavaScript programming allows programmers to deal with more complex program expressions within the ${} syntax. This includes user-defined function calls, use of the ternary operator, and other JavaScript program expressions.
Example of embedding expressions in JavaScript.
let employee = { empname: “Ajit”, empage: 40, empcontact: 94141111111 };
let text = `Welcome, ${employee.empname} ${employee.empcontact}, Your Age Group is ${employee.empage >= 21 ? ‘ Adult’ : ‘Not Adult’}.`;
console.log(text); // result is – Welcome, Ajit 94141111111, Your Age Group is Adult
In this program, the ternary operator tests the age of an employee, and previews the appropriate string value by inserting it based on the result.
Tagging template literals in JavaScript.
You can apply the tag function to manipulate the result of a template literal in a JavaScript program before returning it. This is one of the more advanced features in JavaScript programming, it is used in JavaScript when JavaScript programmers need to process strings in a specific order.
Example of a template literal in JavaScript using a tag.
function upperCasefunc(strings, …values) {
let output = strings[0];
values.forEach((value, index) => {
output += value.toUpperCase() + strings[index + 1];
});
return output;
}
let stuname = “Vinay”;
let stuage = 22;
let display = upperCasefunc`Welcome, ${stuname}, Your age is ${stuage}`;
console.log(display); // result is – Welcome,vinay Your age is 22.
In this program, the JavaScript built-in uppercase function processes the string before returning it, converting the values inserted in the expression to uppercase characters.
Nesting template literals in JavaScript.
You can also use template literals inside other template literals, allowing for complex string interpolation.
Example of nesting template literals in JavaScript.
let stuname = “David”;
let stuage = 29;
let display = `student name is ${`a ${stuname} and student age is ${stuage}`}.`;
console.log(display); // result is – student name is a John and student age is 29.
Advantages of template literals in JavaScript.
- Interpolation – It allows you to insert variables or program expressions directly into a string in JavaScript.
- Multi-line string – You can easily create a multi-line string spanning multiple lines in a JavaScript program.
- Expression evaluation – Any valid JavaScript expression can be inserted inside ${} in a JavaScript program.
- Cleaner syntax – Avoids the need for concatenation (+) operator in JavaScript programs, making it easier for programmers to read and maintain the program code.
Example of template literals in practice in JavaScript.
Suppose you want to create a dynamic message for a student based on their priority in a JavaScript program.
let studentname = “Lalit”;
let courseitems = 1;
let coursePrice = 999.40;
let text = `Welcome, ${studentname} You select ${courseitems} items in your course, and Course price is – $${coursePrice}`;
console.log(text); // result is – Welcome, Lalit You select 1 items in your course, and Course price is – $999.4
In this program, programmers are dynamically creating a message with student information.
Conclusion of template literals in JavaScript programming.
Template literals in JavaScript programming are a great option to make your program code more readable and flexible, especially when you need to insert dynamic values into a declared program string. By using ${} for string interpolation in JavaScript, handling multi-line strings, and embedding complex JavaScript program expressions, you can create clearer and more maintainable program source code.