Declaration and initialization
Strings data type is usually used in C programming language to store character string array text or small paragraph information. Because C language stores and displays 1 byte single character in character data type by default. It is more convenient and easy to declare and process string as array in C program.
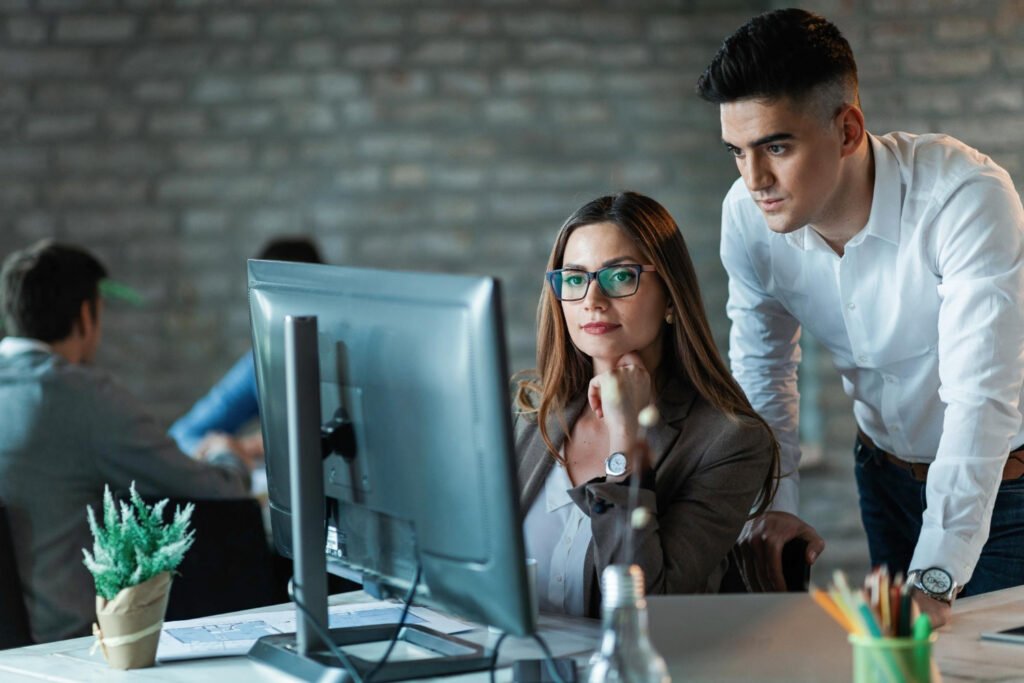
Declaration and initialization of string in C language.
Declaring character array in C program.
char emp_name[50]; // Here a character string emp_name array is declared.
Declare emp_name array with string literal in C program.
char emp_name[50] = “Rahul, Jain”;
Here you have declared a character string array of size 50 characters in C program emp_name. In which string named “Rahul, Jain” is displayed. Where emp_name string will store and process employee name information. Where the string ends it is terminated with null (‘\0’).
When you don’t know the actual string storage array storage.
char company[] = “Microsoft, technology”;
Here empty array of company name is declared in C program. C compiler displays and process array string elements in input string along with string length and string end point null terminator.
Declaring pointers to characters (string pointers) in C programs.
You can declare and initialize strings using pointers to character strings in C program.
char *emp_name = “Jatin, Patel”;
Here you will see, emp_name is pointer to a fixed character content array containing string named “Jatin, Patel”. Here in this example you have not declared the string size explicitly, because this pointer itself holds the string literal address.
About String Literals in C.
String Literals – Strings enclosed in double quotes “…” declared in C program are called string literals. Normal string literals are arrays of characters which are automatically terminated by a null character (‘\0’).
Null Terminator – C strings are null-terminated string terminated point, which means the declared string is automatically terminated with a null character (‘\0’) at the end. The null terminator is automatically used when you declare or initialize a string using string literals in C program.
Character arrays vs pointers in C – When you need to modify string content in C program. So character string array is used char[], similarly character pointers const char * is used to read only string.
String and Character Array Example in C.
#include <stdio.h>
int main() {
char emp_name[50] = “Lalit, Deora”;
char *address = “Rajasthan, India”;
printf(“\n employee name – %s”, emp_name);
printf(“\n employee address – %s”, address);
return 0;
}
In the above example emp_name and address both store different string “Lalit, Deora”. Where emp_name is a character string array. Address is a constant character string array pointer.