Opening, reading, writing, and closing files in PHP (fopen(), fread(), fwrite(), fclose())
In PHP web development scripts, there are some reserved file handling keywords to create and work with various types of files, and PHP provides you a set of built-in functions for file management or file handling. Which gives the web developer file handling control features like efficiently opening existing files, reading existing files, writing files in PHP webpage and closing files.
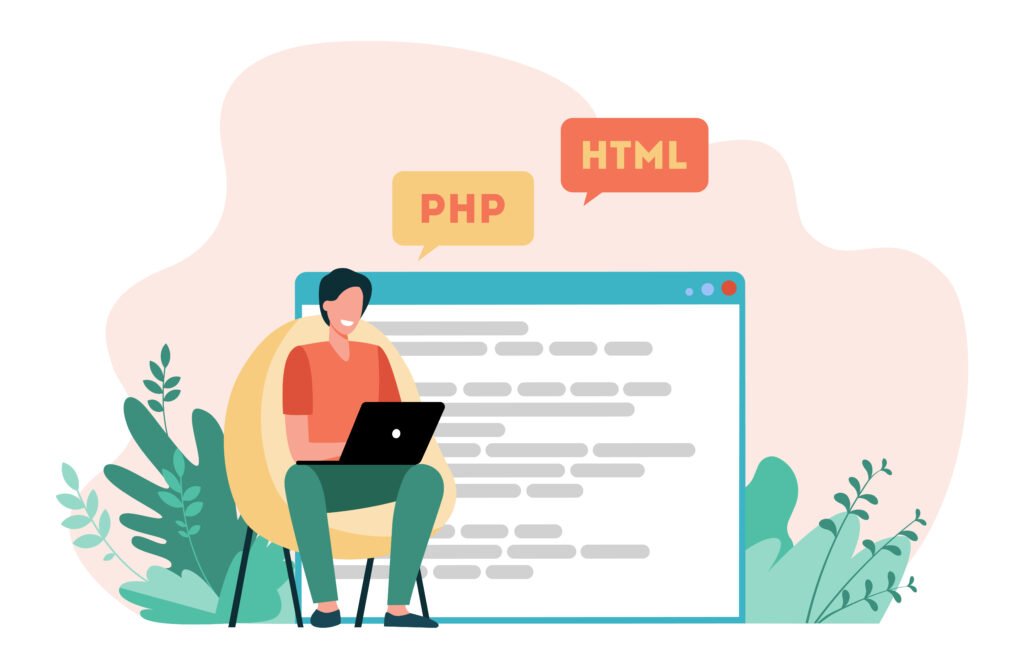
The basic functions used for file handling operations in PHP web development scripts are.
- fopen() – Opens a file in PHP web development script.
- fread() – Reads data from an existing file in PHP web development script.
- fwrite() – Writes data to a file in PHP web development script.
- fclose() – Closes a file after the file operation is completed in PHP web development script.
Opening a file with fopen() in PHP.
The fopen() function in PHP webpage is used to open a file in PHP. To open a file, you need two parameters.
First, we need the name of the file that you want to open.
The way to open an existing file.
The syntax of file open in PHP webpage.
$handle = fopen($filename, $mode);
File control mode in PHP webpage.
$filename – The path storage location of the existing file.
$mode – This is a string that indicates the file access mode, such as reading a file, writing a file, appending a file, etc. It defines the operation.
File handling normal mode in PHP webpage.
- ‘r’ – In PHP webpage, you can open a file in read mode. Here the file pointer starts from the beginning of the file.
- ‘w’ – Opens the file in PHP webpage in write mode. Here, if the file already exists in the PHP webpage, then its default content information is removed. If the file does not exist, it creates it with the file name.
- ‘a’ – Opens the file in PHP webpage in append mode for writing. If the file exists here, the data gets appended at the end. If the file does not exist already, it creates it first.
- ‘r+‘ – Opens a file in PHP webpage for reading and writing. Here the file pointer starts from the beginning of the file.
- ‘w+‘ – Opens the file in PHP webpage for reading and writing. If the file already exists here, it removes its previously stored data and information content.
- ‘a+’ – Opens the file in PHP webpage in append mode for reading the data and information in the existing file and appending new data and information at the end of the file.
Example to read file in PHP webpage.
$filename = “sample.txt”;
$handle = fopen($filename, “r”);
$handle = fopen($filename, “r”);
if ($handle) {
echo “Selected File opened successfully!”;
} else {
echo “File Unable to open.”;
}
Reading file with fread() in PHP webpage.
Once the file is opened with fopen(), you can read its content information using fread(). You pass the file handle and the number of bytes you want to read.
fread() Syntax in PHP webpage.
$content = fread($handle, $length);
fread() function where.
$handle – is the file handle name and file mode returned by the fopen() file handling function in PHP webpage.
$length – indicates the number of bytes to read from the file in PHP webpage. Here you can use the filesize() file handling function to get the size of the file.
Reading file in PHP webpage example.
$filename = “sample.txt”;
$handle = fopen($filename, “r”);
if ($handle) {
// Get the file size
$file_size = filesize($filename);
// Read the entire file content
$content = fread($handle, $file_size);
echo “File content: ” . $content;
fclose($handle);
} else {
echo “Unable to open file.”;
}
Writing to file with fwrite() in PHP webpage.
You can write data to a file using fwrite(). This function writes data to the file handle that you opened previously.
Syntax of fwrite() in PHP webpage.
fwrite($handle, $data);
Where in fwrite().
$handle – This is the file handle value returned by fopen() in PHP webpage.
$data – This is the data in PHP webpage that you want to write to the file.
Writing to file in PHP webpage example.
$filename = “sample.txt”;
$handle = fopen($filename, “w”);
if ($handle) {
$data = “Let add some new content to file.”;
fwrite($handle, $data);
echo “Data write successfully!”;
fclose($handle);
} else {
echo “Unable to open file.”;
}
Here in this example, the content information in the existing file will be overwritten because we have opened the file in “w” mode.
Appending to file in PHP webpage.
If you want to append file data and information to the bottom of the active file in your current PHP webpage without overwriting the file content information, you can open the file in append mode (‘a’ or ‘a+’).
Appending to file in PHP webpage example.
$filename = “sample.txt”;
$handle = fopen($filename, “a”);
if ($handle) {
$data = “\n Here we add content at the end of the file.”;
fwrite($handle, $data);
echo “Data append operation successfully!”;
fclose($handle);
} else {
echo “Unable to open file.”;
}
This appends the file content data information to the bottom end of the file without modifying the existing file data and information in this example.
Closing file with fclose() in PHP webpage.
In PHP webpage, after performing file operations such as reading or writing to a file, the file is closed with the fclose() function to empty the file resources.
fclose() syntax in PHP webpage.
fclose($handle);
fclose() in PHP webpage where.
$handle – This is the file handle information returned by fopen() in the current PHP webpage.
Example of fclose() in PHP webpage.
$filename = “sample.txt”;
$handle = fopen($filename, “r”);
// it used to Read the file content…
$content = fread($handle, filesize($filename));
echo “File content is – ” . $content;
// it used to Close the file
fclose($handle);
Complete example of reading, writing and closing a file in PHP webpage.
Here you get a complete example of opening a file, reading the file content information, writing the content to the file, and finally closing it.
$filename = “sample.txt”;
/// Open the file for reading and writing in PHP webpage
$handle = fopen($filename, “r+”);
if ($handle) {
// it used t Read the existing content of file
$content = fread($handle, filesize($filename));
echo “The Default Content is – ” . $content;
// it Move the file pointer to the end of the file
fseek($handle, 0, SEEK_END);
// Write new content to the file
$new_data = “\n Let add some new content to the file.”;
fwrite($handle, $new_data);
// Close the file
fclose($handle);
} else {
echo “Unable to open the file.”;
}
File Handling Error Management in PHP Webpage.
Before proceeding with any file reading or file writing operation in the current PHP webpage, always check if the file exists and is opened successfully. e.g.,
$handle = fopen($filename, “r”);
if ($handle === false) {
echo “Display Error when opening the file!”;
} else {
// it allow Proceed with reading or writing file
fclose($handle);
}
Basic Summary of File Functions in file handling.
Function | Description |
fopen() | Fopen() function used to Opens a file in a specified mode. |
fread() | Fread() used to Reads data from an open file. |
fwrite() | Fwrite() used to Writes data to an open file. |
fclose() | Fclose() function used to Closes an open file after reading/writing. |
Multiple Modes for file handling fopen() operation.
Mode | Description |
r | R mode used to Open for reading only. The file pointer is placed at the beginning. |
w | W mode used to Open for writing only. If the file exists, it is overwritten. |
a | A mode used to Open for writing only. If the file exists, data is appended. |
r+ | R+ mode used to Open for reading and writing. The file pointer is placed at the beginning. |
w+ | W+ mode used to Open for reading and writing. If the file exists, it is overwritten. |
a+ | A+ mode used to Open for reading and appending operation. |