Importing modules in python
In Python programming, importing some particular modules or packages for some specific programming operations allows you to use module source code organized in separate files or libraries present or supported in Python. This enables reuse of module program source code and modularity features in the program.
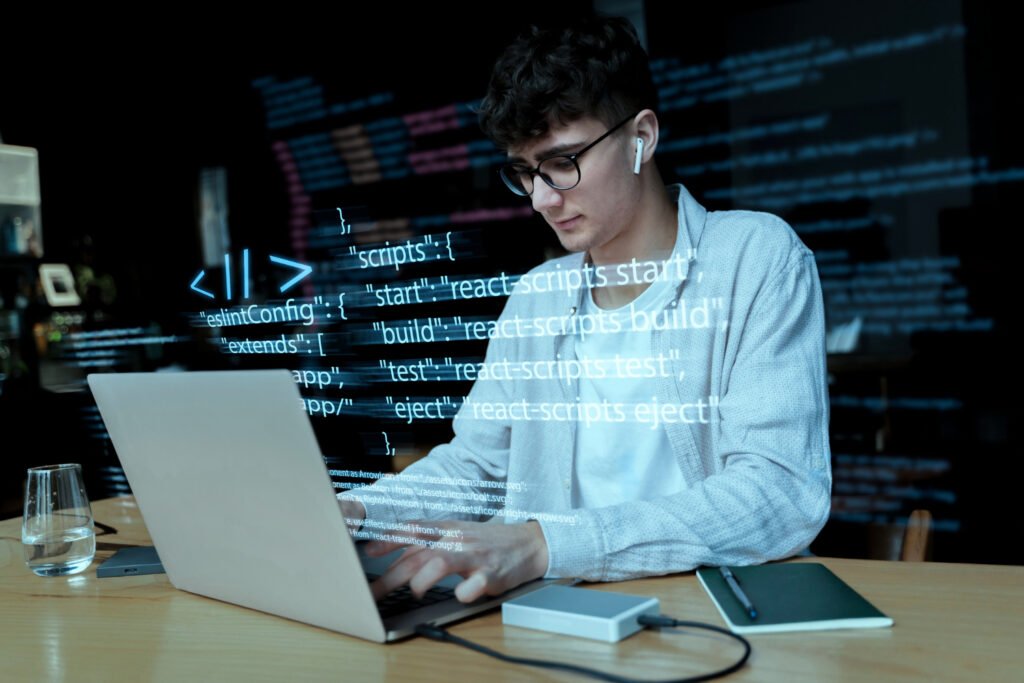
So let’s learn the steps to import different modules in Python.
Types of Python Module Imports.
Python programming provides several methods to import modules into the existing program.
python Importing Entire Module.
import module_name
Importing entire math module in Python Example.
import math
print(math.sqrt(81)) # the result is – 9.0
python Importing Specific Items from a Module.
from module_name import item_name
sqrt math module example.
from math import sqrt
print(sqrt(625)) # the result is – 25
Importing All Items from a Module (generally not use/restricted).
from module_name import *
This immediately imports all items (functions, classes, variables) from module_name called by the programmer into the current namespace of the program in Python. Sometimes conflicts may occur while calling import all modules completely, and it becomes a bit difficult to understand the program source code.
Renaming the imported module or item in Python.
import module_name as Alias
from module_name import item_name as Alias
Example of numpy module import in Python.
import numpy as np
from datetime import datetime as dt
Importing from a Python program package.
If your program module is part of a package (Python module directories), you can import it at the start of the Python program using dot notation.
import package_name.
module_name
from package_name import module_name
Example of pandas module import in Python.
import pandas.DataFrame
from sklearn import tree
Best practices for importing modules in Python programs.
Import only those module packages in Python program that you need to import in your existing program. Remember to avoid using from module_name import * in Python program.
Remember to be clear about where each item is imported and understand it better at the beginning of the program to increase the readability and program maintainability of Python module program code.
Organize Python imports.
Python programs Place all import statements either written or manually at the beginning of your Python program file, right after any module-level docstrings.
Group imports in Python programs in the following order.
Standard library imports
Related third-party imports
Local application/library-specific imports
Avoid circular imports in Python programs.
Circular imports in Python programs occur when two or more modules try to import each other directly or indirectly. Sometimes these multiple imports can cause runtime errors or unexpected program behavior.
Use aliases appropriately in Python programs.
Import aliases (such as alias_name) in Python programs can be helpful to shorten long import module names or avoid name conflicts.
So always choose meaningful and consistent program import module aliases to increase program code clarity.
Understanding __init__.py file in Python programs.
If a Python program directory is imported as a package, it must contain an __init__.py file (which may already be empty) to indicate to the program that it is part of a Python program import package.
Importing and using modules in a Python program.
So let’s learn how to import and use essential program modules in a Python program with an example.
# Importing standard library modules in Python
import math
print(math.sqrt(121)) # the result is – 11
# Importing and using an alias in a Python program
import numpy as np
array1 = np.array([4, 9, 6, 8, 10, 12, 7])
print(np.mean(array1)) # the result is – [ 4 9 6 8 10 2 7]
# Importing from Python package
from sklearn import tree
clf = tree.DecisionTreeClassifier()
# Importing Python local module
from test_module import test_function
test_function()
In the Python import module example above.
Importing Python’s built-in standard library module (math).
Importing a third-party library (numpy) with an alias (np).
Importing a package (sklearn) and its submodule (tree).
Importing a local module (test_module) and its function (test_function).
By following the different steps given above and knowing the different ways of importing different modules in a Python program, you can manage program dependencies in an effective order, increase Python program code organization as per the need, and take advantage of the rich ecosystem mechanism of Python libraries and packages for your programming project.