File operations (opening, closing, reading, writing)
File handling in C programming is used for various file operation tasks in C program. By file operation, you can perform file operation tasks like open, close, read, write, etc. in C program. Where you access the file operation function from standard input/output library I/O (stdio.h) header file in C program.
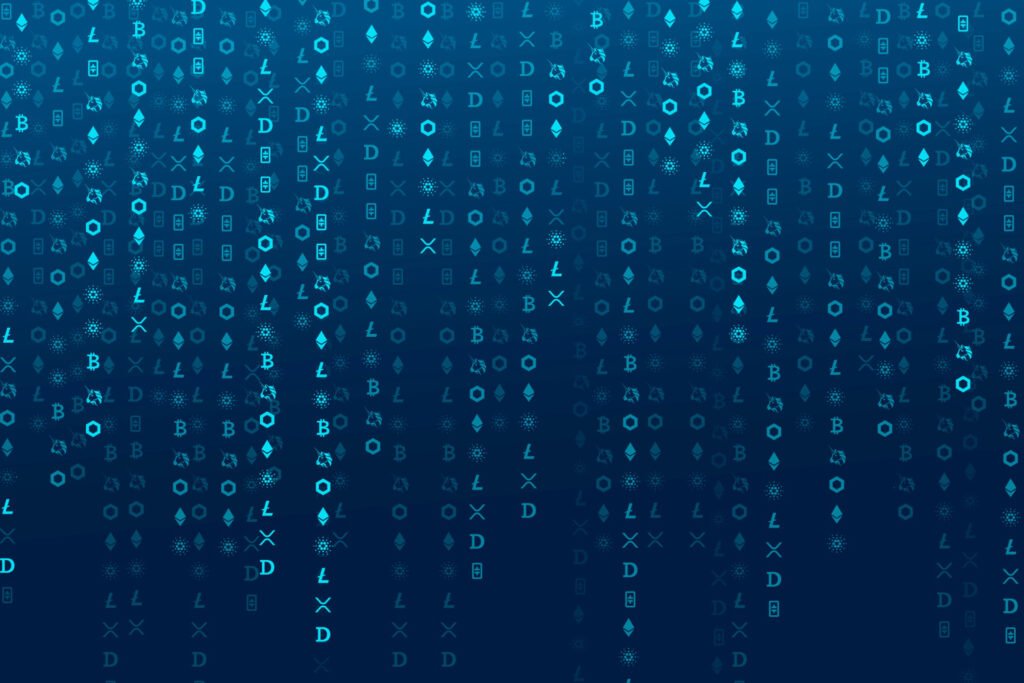
So, let’s understand file operation or file handling operation in C program.
Opening a file in C.
To open a file in C program, fopen() function built-in file library function is used. To open the file, you have to provide the name of the open file and the mode to open the file.
FILE *file = fopen(“test.txt”, “r”); // Open test file for reading file operation
FILE *file = fopen(“test.txt”, “w”); // Open test file for writing purpose only
FILE *file = fopen(“test.txt”, “a”); // Open test file for appending/adding some file content
Closing a File in c program.
If you want to close the file, you can use the fclose() file function.
fclose(filename);
Reading a File in C Program.
If you want to read any existing file in your C program, you can read text information from any file using many file reading file handling functions. Here are some popular file text information reading functions. Like, fgetc() function, fgets() function, and fread() function. etc.
fgetc(): // fgetc() function is used to read a single character from the file.
fgets(): // fgets() function is used to read a string text information from the existing file.
fread(): // fread() function is used to read binary data information from a file.
FILE *file = fopen(“test.txt”, “r”); // open test file for reading mode
if (file == NULL) {
perror(” Found Error While opening a test.txt file”);
return 1;
}
char txt = fgetc(file); // the fgetc function used to read a single character
printf(“\n %c”, txt);
char string[150];
fgets(string, 150, file); // the fgets function used to read a string
printf(“\n %s”, string);
Writing text information to a C program file.
If you want to write single character data or information in a C programming file. Then you use the fputc() function, fputs() function, or fwrite() function to write text information in file handling.
fputc(): // fputc() function is used to write a single character in a file.
fputs(): // fputs() function is used to write a string text information into a file.
fwrite(): // fwrite() function is used to write binary data and information into a file.
FILE *file = fopen(“test.txt”, “w”);
if (file == NULL) {
perror(“Error during opening file for writing”);
return 1;
}
fputc(‘T’, file); // fputc( ) function used to Write a single character into test file
fputs(“\n append text, into file”, file); // fputs( ) function used to Write a string text information
fclose(file);
Example of reading a file and writing inside a file in C programming.
So let’s create a program in C language. Which manages file handling operations like opening a file, writing to the file, closing the file, then reading and writing that file.
#include <stdio.h>
int main() {
FILE *file = fopen(“test.txt”, “w”); // Open a test.txt file for text writing
if (file == NULL)
{
perror(“you getting Error while opening file for writing”);
return 1;
}
// Write a single character or string to the test.txt file
fputs(“\n this is, file”, file);
fputs(“\n This is a test file write information”, file);
// Close the above test.txt file
fclose(file);
// Open a test.txt file for reading purpose
file = fopen(“example.txt”, “r”);
if (file == NULL) {
perror(“you getting Error while opening a file for reading purpose”);
return 1;
}
// let Read and print the content of the file according to need
char string[150];
while (fgets(string, 150, file) != NULL) {
printf(“%s”, string);
}
// fclose function used to close the file
fclose(file);
return 0;
}
Here above you have performed read and write operation of text and string in a file in C program. Where you learned file operations like opening text.txt file, closing file, reading and writing to file, etc.