Dynamic memory allocation (malloc, calloc, realloc, free) in hindi
C प्रोग्रामिंग में डायनेमिक मेमोरी एलोकेशन प्रोग्राम को रनटाइम पर मेमोरी का रिक्वेस्ट करने पर नई मेमोरी एलोकेशन, एक्सिस्टिंग मेमोरी को रीलॉक, और malloc फंक्शन द्वारा आवंटित मेमोरी स्पेस को free करने की अनुमति प्रदान करता है. जिससे प्रोग्राम वेरिएबल डेटा के विभिन्न टाइप्स को मैनेज करना और स्टोरेज मेमोरी का कुशल प्रबंधन करना अधिक लचीला और सुगम हो जाता है। सी प्रोग्राम में डायनेमिक मेमोरी एलोकेशन के लिए उपयोग किए जाने वाले कुछ पॉपुलर फ़ंक्शन यहाँ दिए गए है, जैसे, malloc() function, calloc() function, realloc() function, और free() function है.
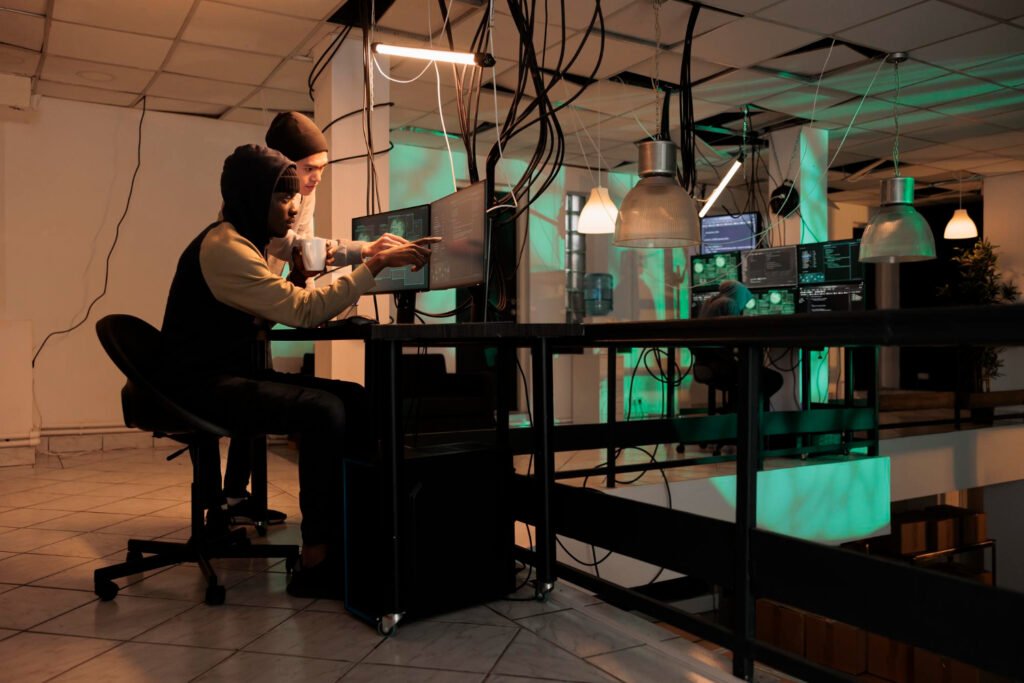
Examples of dynamic memory allocation in C programs.
Using malloc (memory allocation) in C.
malloc() फंक्शन सी प्रोग्राम में नए स्पेस बाइट्स में डिफाइन साइज की मेमोरी का एक नया ब्लॉक साइज अलॉट करता है।
syntax –
void* malloc(size_t size);
size – मौजूदा सी प्रोग्राम में नए स्पेस ब्लॉक को एलोकेशन करने के लिए बाइट्स की संख्या है।
यहाँ मेमोरी एलोकेशन के लिए void* पॉइंटर रिटर्न होता है, या ये फंक्शन मेमोरी एलोकेशन विफल होने पर NULL वैल्यू रिटर्न करता है।
Example of malloc() in C.
#include <stdio.h>
#include <stdlib.h>
int main() {
int *ptr = (int *)malloc(7 * sizeof(int)); // it allocates memory with array of 7 integer
if (ptr == NULL) {
printf(“\n Memory allocation process is unsucessfull!”);
return 1;
}
for (int p = 0; p < 7; p++) {
ptr[p] = p * 5; // assign the value to p
printf(“%d “, ptr[p]); // print the p array variable value
}
printf(“\n”);
free(ptr); // free() function free the allocated memory by maclloc function
return 0;
}
calloc (contiguous allocation) in C.
calloc फंक्शन का उपयोग ऐरे एलिमेंट्स के लिए कन्टीन्यूस मेमोरी एलोकेशन करना है. जहा calloc फंक्शन के उपयोग के साथ ऐरे के सभी बाइट्स को जीरो पर स्टार्ट करता है। यहाँ याद रखे की calloc फंक्शन एलोकेशन मेमोरी को शून्य पर स्टार्ट किया जाता है।
Syntax of calloc() in c.
void* calloc(size_t value, size_t size);
value – Number of elements with calloc.
size – the Size of each element in bytes.
यहाँ आल्लोट मेमोरी के लिए void* पॉइंटर रिटर्न करता है, या अलॉटमेंट विफल होने पर NULL वैल्यू को रिटर्न करता है।
Example of calloc() in C.
#include <stdio.h>
#include <stdlib.h>
int main() {
int *ptr = (int *)calloc(7, sizeof(int)); // it Allocates memory for 7 integer array start with 0
if (ptr == NULL) {
printf(“\n Memory allocation unsucessfull!”);
return 1;
}
for (int p = 0; p < 7; p++) {
printf(“%d “, ptr[p]); // it read and print the array values
printf(“\n”);
free(ptr); // free() function Free the allocated memory in array
return 0;
}
realloc (reallocate) in C.
सी प्रोग्राम में realloc() फंक्शन पहले से प्रोग्राम में अलॉट मेमोरी ब्लॉक का आकार नए आकर से बदल देता है. realloc फंक्शन कंटेंट को पुराने और नए आकारों के न्यूनतम तक मैनेज करता है।
realloc (reallocate) in C.
void* realloc(void* ptr, size_t size);
ptr – यहाँ ptr पहले से अलॉट मेमोरी ब्लॉक का पॉइंटर वैल्यू है।
size – यहाँ साइज पहले से अलॉट बाइट्स में नया आकार है।
यहाँ नए अलॉट मेमोरी के लिए void* पॉइंटर रिटर्न करता है, या यदि पुनः अलॉटमेंट विफल हो जाता है, यह तो NULL वैल्यू रिटर्न करता है।
Example of realloc() in C.
#include <stdio.h>
#include <stdlib.h>
int main() {
int *ptr = (int *)malloc(7 * sizeof(int)); // it Initialize memory allocation with 7 integers
if (ptr == NULL) {
printf(“\n the Memory allocation is unsuccessfull!”);
return 1;
}
for (int p = 0; p < 7; p++) {
ptr[p] = p * 5; // Assign value to p variable
}
ptr = (int *)realloc(ptr, 12 * sizeof(int)); // it Resize to hold 12 new integers value with realloc function
if (ptr == NULL) {
printf(“\n the Memory reallocation unsuccessfull!”);
return 1;
}
for (int p = 7; p < 12; p++) {
ptr[p] = p * 12; // it Assign new values to array element
}
for (int p = 0; p < 12; p++) {
printf(“%d “, ptr[p]); // it print the all array element values
}
printf(“\n”);
free(ptr); // the free () used to Free the allocated memory in array
return 0;
}
free (deallocation) function in C language.
फ्री फंक्शन सी प्रोग्राम में पहले से अलॉट मेमोरी ब्लॉक को स्टोरेज लोकेशन से डीलोकेशन करता है. यहाँ free() का सिंपल उपयोग अलॉट मेमोरी बाइट को फ्री करने में होता है।
Syntax of free() in C.
void free(void* ptr);
ptr – यहाँ ptr वेरिएबल फ्री होने वाले मेमोरी ब्लॉक का पॉइंटर है।
यहाँ कोई रिटर्न वैल्यू नहीं।
Example of free() in C.
#include <stdlib.h>
void testFunction() {
int *ptr = (int *)malloc(50 * sizeof(int)); // it allocate 50 byte memory
if (ptr != NULL) {
// use the exisitng memory
free(ptr); // now the free () used to free the memory when we need to free them
ptr = NULL; // assign pointer to null
}
}