Dictionaries in Python
In Python programming, the dictionary (dict) data type is a powerful data storage element structure that helps Python programmers to store declared dictionary data elements in key-pair order. Remember, Python dictionary data types are mutable, unordered collections of dictionary objects. Where in each Python program, declared dictionary items are stored and processed in key-pair order.
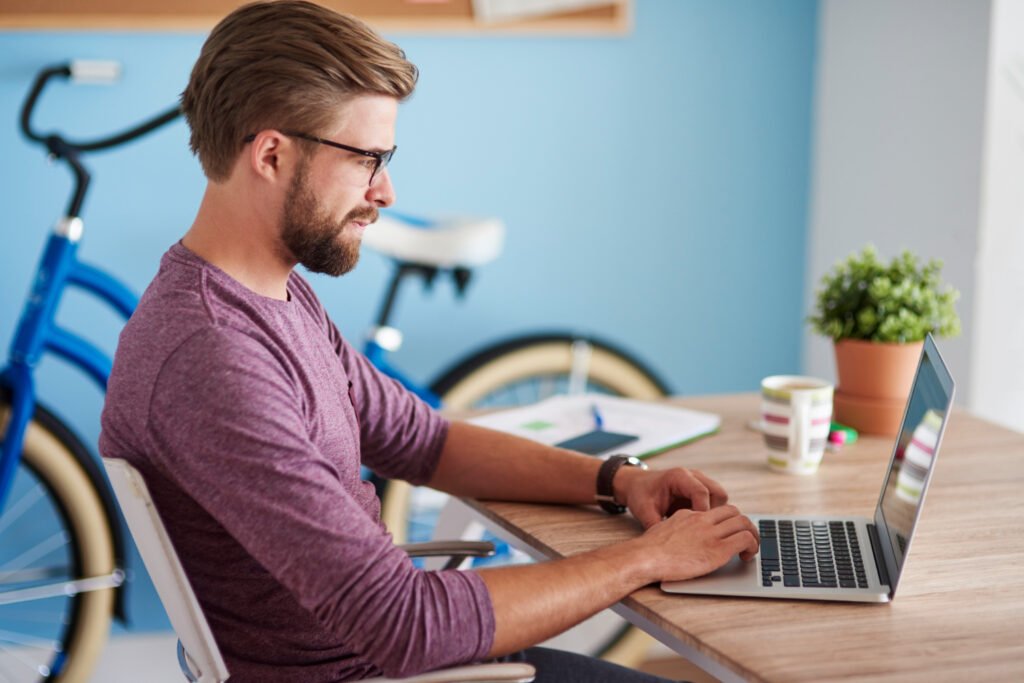
So let’s understand the dictionary data type better in Python programming.
Creating a dictionary data element in Python.
If you want to declare a dictionary data type in a Python program, you can create a dictionary data by enclosing different dictionary data elements in key-pair combinations with curly braces {} along with the comma operator.
# let Creating an no element dictionary in Python
sample_dict = {}
# let create a dictionary element with its key-pair element
cloth = {
“shirts”: 9,
“t shirts”: 8,
“trowser”: 11
}
# let create a python dictionary data type with multiple combination of data values
employee = {
“emp_name”: “ajit”,
“emp_age”: 45,
“salary”: 999.00
}
Basic python dictionary example.
# let create a dictionary element with its key-pair element
clouth = {
“shirts”: 9,
“t shirts”: 8,
“trowser”: 11
}
# let create a python dictionary data type with multiple combination of data values
employee = {
“emp_name”: “ajit”,
“emp_age”: 45,
“salary”: 999.00
}
print(clouth[“shirts”]) # the result is – 9
print(clouth[“trowser”]) # the result is – 11
print(employee[“emp_name”]) # the result is – ajit
print(employee[“salary”]) # the result is – 999.0
Accessing Python Dictionary Elements.
You can access the values associated with the keys in a dictionary declared in Python program by using square brackets [] and dictionary key.
# Accessing values by Python dictionary data key
# let use Python dictionary data Accessing values by its key value
print(clouth[“shirts”]) # the result is – 9
print(employee[“emp_name”]) # the result is – ajit
If the dictionary key does not exist, accessing the dictionary data will generate a key error. To avoid this, you can use the Python get() method, which allows you to specify default values when the dictionary data key is not found.
print(clouth.get(“shirts”, 0))
print(clouth.get(“trowser”, 0))
Modifying Python dictionary data.
Dictionary data types declared in a Python program are mutable, so you can modify your dictionary keys by defining new values, adding new key-value pairs, or modifying existing dictionary elements by adding or deleting existing key values.
# let Modifying dictionary existing element values
clouth[“shirts”] = 13
print(clouth) # the resut is – {‘shirts’: 13, ‘t shirts’: 8, ‘trowser’: 11}
# let Adding or deleting new key-values to the existing dictionary
clouth[“vimal shirt”] = 15
print(clouth) # the resut is – {‘shirts’: 13, ‘t shirts’: 8, ‘trowser’: 11, ‘vimal shirt’: 15}
# let Deleting Python dictionary data element key-value pairs
del clouth[“trowser”]
print(clouth) # the resut is – {‘shirts’: 13, ‘t shirts’: 8, ‘vimal shirt’: 15}
Python dictionary operations.
In a Python program, you can perform various programming operations on a dictionary, such as checking for members (in), repetition on keys, values or items, and finding the length (len()).
# let check member in Python dictionary member
print(“shirts” in clouth) # the result is – true
print(“trowser” in clouth) # the result is – true
# let repeatition over Python dictionary keys values
for key in clouth:
print(key)
# let repeat over Python dictionary values
for value in clouth.value():
print(value)
# let repeat on move over Python dictionary key-value pairs
for key, value in clouth.items():
print(key, value)
# let findout the Length of Python dictionary element
print(len(clouth))
Python Dictionary Comprehension.
Similar to list comprehensions in Python programming, you can create dictionary data elements using dictionary comprehensions in Python programs.
# let Create a dictionary of square root of numbers from 2 to 10
sqrt = {p: p**2 for p in range(2, 10)}
print(sqrt) # let teh result is – {2: 4, 3: 9, 4: 16, 5: 25, 6: 36, 7: 49, 8: 64, 9: 81}
Python nested dictionaries.
Dictionaries can be included as other dictionary values inside Python programs, allowing you to create nested dictionary data structures.
# let create Python Nested Dictionary
developer = {
“lalit”: {“age”:40, “web developer”: “forntend developer”},
“ajit”: {“age”:39, “python developer”: “backend developer”}
}
print(developer[“lalit”]) # the result is – {‘age’: 40, ‘web developer’: ‘forntend developer’}
print(developer[“ajit”]) # the result is – {‘age’: 39, ‘python developer’: ‘backend developer’}
Use of Dictionaries in Python.
Dictionary Mapping – When you need to map keys to compatible values in a Python program, you can use the dictionary data type element.
Dictionaries Faster Lookup – Dictionaries provide fast lookup time features to access dictionary values based on dictionary keys in a Python program.
Dictionaries Flexible Data Structure – Use dictionary data type for structure data in Python programming. Where each part of the stored dictionary data information is associated with a unique key.