CASE and IF Statements
In SQL database table, both CASE and IF statements are used to handle or perform conditional and logical expressions in database table. While Case and If statements allow you to apply conditional logic expressions within a query to modify, customize, or filter table data based on some particular specific conditions. While both Case statement and If statement are used to execute conditional database table expressions.
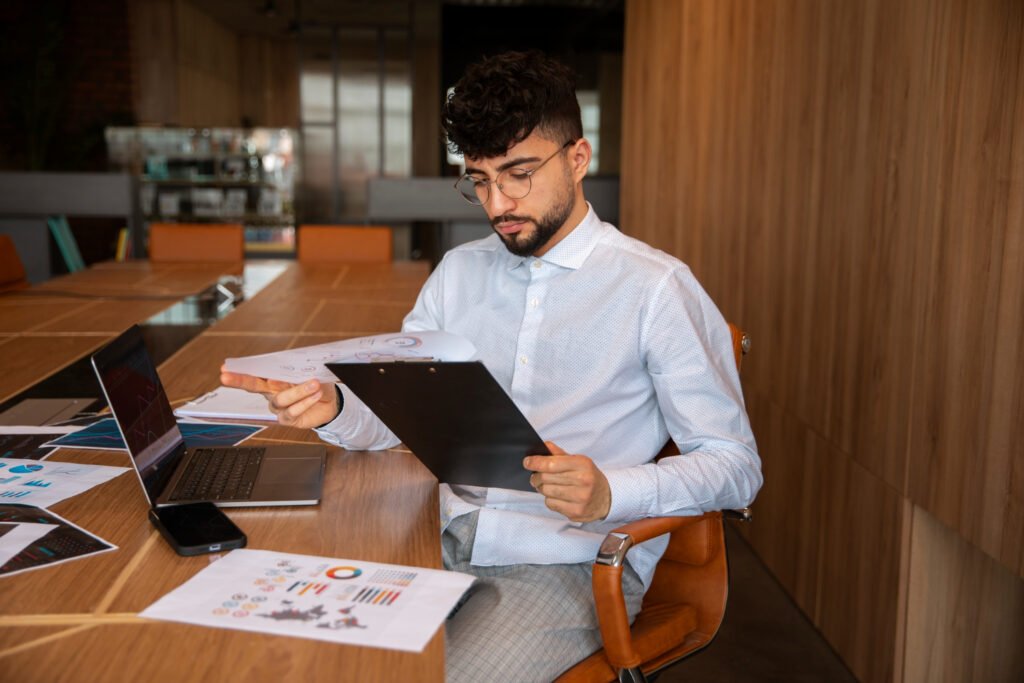
CASE Statement in SQL Database Table.
CASE statement in SQL table is a flexible and better method of applying conditional logic in SQL. It helps database operator to manage and perform multiple database activities based on multiple case statements, where CASE statement in SQL database table is used to perform basic to advanced database query operation with case statement in both SELECT clause (to return multiple table column values) and WHERE clause (to filter database table data based on particular condition).
There are two types of CASE statements in SQL database table.
- Simple CASE – It compares an expression in existing SQL database table to multiple possible values.
- Searched CASE – It evaluates conditions in existing SQL database table (using boolean programming expressions) and produces database query result based on the first condition you use, which is the true result.
Syntax for simple CASE statement in SQL.
SELECT column_name,
CASE column_name
WHEN value1 THEN result1
WHEN value2 THEN result2
ELSE default_result
END AS alias_name
FROM table_name;
Syntax for searched CASE statement in SQL.
SELECT column_name,
CASE
WHEN condition1 THEN result1
WHEN condition2 THEN result2
ELSE default_result
END AS alias_name
FROM table_name;
Example of simple CASE statement in SQL database table.
Suppose you have a SQL database table named orders with some table columns like order_id, status and amount in default table column. Here you want to classify each order group by setting the condition of each order as “complete”, “pending” or “cancelled”.
Conditions in SQL Database Table Explanation.
SELECT order_id,
CASE status
WHEN ‘C’ THEN ‘Complete’
WHEN ‘P’ THEN ‘Pending’
WHEN ‘X’ THEN ‘Cancel’
ELSE ‘Unknown’
END AS order_status
FROM orders;
Here in the existing SQL database table, the CASE expression analyzes and tests the values of the condition column and returns a string description value for each condition (‘complete’, ‘pending’, ‘cancelled’) in the orders table.
Here in this case expression, if any condition does not match properly, then it returns the value ‘unknown’.
Example of CASE statement searched in SQL database table.
Here in the SQL database table, you want to display the employees classified on the basis of their salary. Then you can use the searched CASE statement.
Example of the Searched CASE Statement.
SELECT employe_id, name, salary,
CASE
WHEN salary < 20000 THEN ‘Low’
WHEN salary BETWEEN15000 AND 50000 THEN ‘Medium’
WHEN salary > 60000 THEN ‘High’
ELSE ‘Unknown’
END AS salary_category
FROM employe;
CASE statement in SQL database table explanation.
Here the CASE expression in the existing SQL database table aggregates the salary of each employee, and classifies them in the order of “low”, “medium” or “high” based on the salary range.
If the salary in the existing SQL database table is not in any of the given ranges, it returns the value ‘Unknown’.
IF Statement in SQL in SQL Database Table.
The IF statement in SQL is commonly used to handle conditionals when writing if condition expressions in procedural SQL operations, such as stored procedures or functions in MySQL or SQL Server databases. The if condition statement is often used to manage condition control flow logic. You can use IF statement to display queries in SQL database table, you can manage and control your database table operations with IF statement based on multiple filters.
Syntax of IF statement in SQL database table.
SELECT column_name,
IF(condition, value_if_true, value_if_false) AS alias_name
FROM table_name;
- IF condition – Used to evaluate a particular IF statement condition in an existing SQL database table.
- IF value_if_true – It returns the value if the IF statement condition is true in the existing SQL database table.
- IF value_if_false – It returns the value if the IF statement condition is false in the existing SQL database table.
Example of IF statement in SQL database table.
Here in the existing SQL database table, if the employee’s salary is less than Rs 20,000 then you want to classify them as “Junior” employee, and if their salary is greater than or equal to Rs 20,000 then you want to classify them as “Senior” employee.
SELECT employe_id, name, salary,
IF(salary < 20000, ‘Junior’, ‘Senior’) AS employe_level
FROM employe;
Explanation of IF statement in SQL database table.
It evolves the IF statement in an existing SQL database table to check if the salary is less than or equal to 20000. If the IF statement is true, it returns the ‘junior’ value; otherwise, it returns the ‘senior’ value in the table.
Key Differences Between CASE and IF Statements
Feature | CASE Statement | IF Statement |
Syntax | Case statement is More flexible, can handle multiple database statement conditions with WHEN and ELSE. | If statement is Simpler, evaluates one table condition and returns either a value in true or false result order. |
Use Cases | Case statement is Suitable for both SELECT, UPDATE, DELETE, and WHERE clauses with sql table. | Where if statement is Primarily used in MySQL SELECT queries but more commonly used in stored procedures or functions with programming concept. |
Multiple Conditions | With case statement Can handle multiple conditions (using WHEN, THEN) clause. | With if statement Evaluates a single condition. (More suited for simple conditions). |
Support | Case statement Standard in all SQL (works across most databases) version. | Where if statement More commonly used in MySQL, less common in standard SQL queries with database table. |
CASE and IF statements in SQL database table.
- CASE in SELECT clause – It helps in complex conditional logic in queries in existing SQL database tables, especially when handling multiple conditions or categories.
- IF in MySQL – The IF statement helps in testing simple logic within SELECT queries or inline table column expressions while dealing with basic conditional operations in existing SQL database tables.
CASE and IF statement inference in SQL database tables.
- SQL CASE Statement – Used for evolution of multiple conditions or expressions, more flexible and robust, in any existing SQL database table, you can apply case statement in all major SQL databases.
- SQL IF Statement – This is a simple statement in an existing SQL database table, you can use the If statement mainly in MySQL or other procedural SQL. Where in the If statement you only need the result of the binary true/false condition.