Bitwise operators in c
Bitwise operators in C language manipulate individual bits within an integer type in a storage location. Bitwise operators are commonly used in low-level computer programming, such as low-level computer programming, systems programming, embedded systems, computer hardware, and other applications.
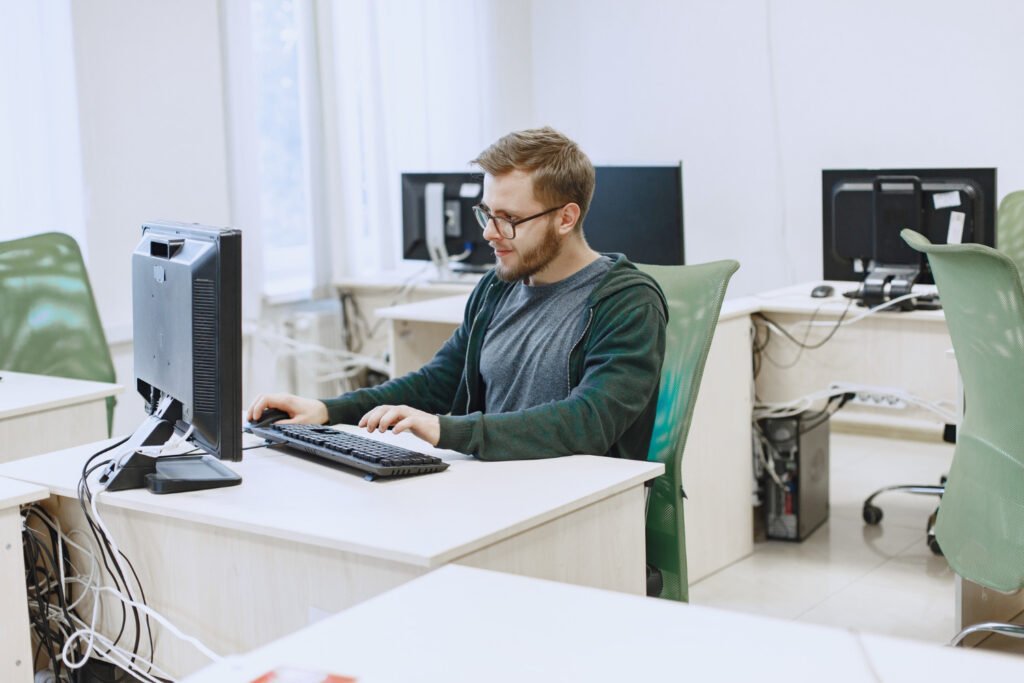
Types of Bitwise Operators in C Language.
- AND (&)
- OR (|)
- XOR (^)
- NOT (~)
- Left Shift (<<)
- Right Shift (>>)
C Language Bitwise AND (&) Operator.
The AND operator in C language compares each bit of its operands. In the AND operator, if both bits are 1, then the result is 1, otherwise, the result is 0.
AND Operator Example in C Language.
#include <stdio.h>
int main() {
int p = 7;
int q = 9;
int output = p & q;
printf(“\n p & q and operator is – %d\n”, output);
return 0;
}
Bitwise OR (|) operator in C.
The OR operator compares each bit of its operands. If at least one bit is 1, the result is 1, otherwise, the result is 0.
Bitwise OR example.
#include <stdio.h>
int main() {
int p = 9;
int q = 7;
int output = p | q;
printf(“\n the or operator p | q = %d”, output);
return 0;
}
Bitwise XOR (^) in C.
The Bitwise XOR operator in C language compares each bit of its operands. If the bits are different elements, the result is 1, otherwise, the result is 0.
Example of Bitwise XOR (^).
#include <stdio.h>
int main() {
int p = 4;
int q = 6;
int output = p ^ q;
printf(“\n p ^ q xor values is – %d”, output);
return 0;
}
Bitwise NOT (~) in C.
C Language Bitwise NOT Operator It reverses all the bits value of its operand.
Example of Bitwise NOT in C.
#include <stdio.h>
int main() {
int p = 7;
int output = ~p;
printf(“\n the bitwise not ~p = %d”, output);
return 0;
}
Left Shift (<<) Operator in C.
The left shift bitwise operator in C program moves the bits to the left by the specific location position. It then ignores the shifted storage bits location, and moves zero bits from the right.
Left shift bitwise operator example in C.
#include <stdio.h>
int main() {
int p = 7;
int output = p << 1;
printf(“the left shit operator is p = << 1 = %d”, output);
return 0;
}
Right Shift (>>) Operator in C.
The right shift operator in C language shifts the bits to the right in a specific location. In unsigned data types, the data bits shifted from the end are ignored, and zero bits are shifted from the left. For unsigned data types, the sign bit is used to fill the leftmost bits (arithmetic move).
Right Shift Operator Example.
#include <stdio.h>
int main() {
int p = 7;
int output = p >> 1;
printf(“\n right shit operator p >> 1 location is – %d\n”, output);
return 0;
}
Bitwise Operations in C Program.
Setting a Bit in C Program Example.
To set a specific bit location to 1, you use the bitwise OR operator with a mask in which the desired bit is moved by 1 location.
#include <stdio.h>
int main() {
int p = 9;
int bit_loc_set = 1;
int output = p | (1 << bit_loc_set);
printf(“\n the output after setting the bit location – %d – %d”, bit_loc_set, output);
return 0;
}
Clearing a bit in C example.
To clear a specific bit to 0 in a C program, you use the bitwise AND operator with a mask that stores the desired bit at the 0 location.
#include <stdio.h>
int main() {
int p = 7;
int bit_loc_clear = 3;
int output = p & ~(1 << bit_loc_clear);
printf(“\n the output after the clearing bit – %d %d\n”, bit_loc_clear, output);
return 0;
}
Bit toggling in C example.
In a C program, to toggle a particular bit (reverse it), the bitwise XOR operator is used with a mask with the desired bit stored at location 1.
#include <stdio.h>
int main() {
int p = 7;
int bit_loc_toggle = 0;
int output = p ^ (1 << bit_loc_toggle);
printf(“\n the output after toggling bit is – %d %d\n”, bit_loc_toggle, output);
return 0;
}
Example Checking a Bit in c.
In a C program, to check whether a specific bit is set, the bitwise AND operator is used with a mask with the desired bit set at location 1.
#include <stdio.h>
int main() {
int p = 7;
int bit_loc_check = 5;
int output = p & (1 << bit_loc_check);
if (output) {
printf(“\n the checking Bit is set to – %d”,bit_loc_check);
}
else { printf(“\n the checking Bit is not set to – %d”,bit_loc_check);
} return 0;
}