Basics of pointers
Pointers are a fundamental concept in C programming. It allows the C programmer to directly work with the memory address of different program variables declared in the program. Pointers allow you to access and manipulate the memory address location of data type variables indirectly. This allows dynamic memory allocation of data type variables in C language, passing program arguments by reference through pointer, and efficiently performing programming operations with array data type addresses and strings elements.
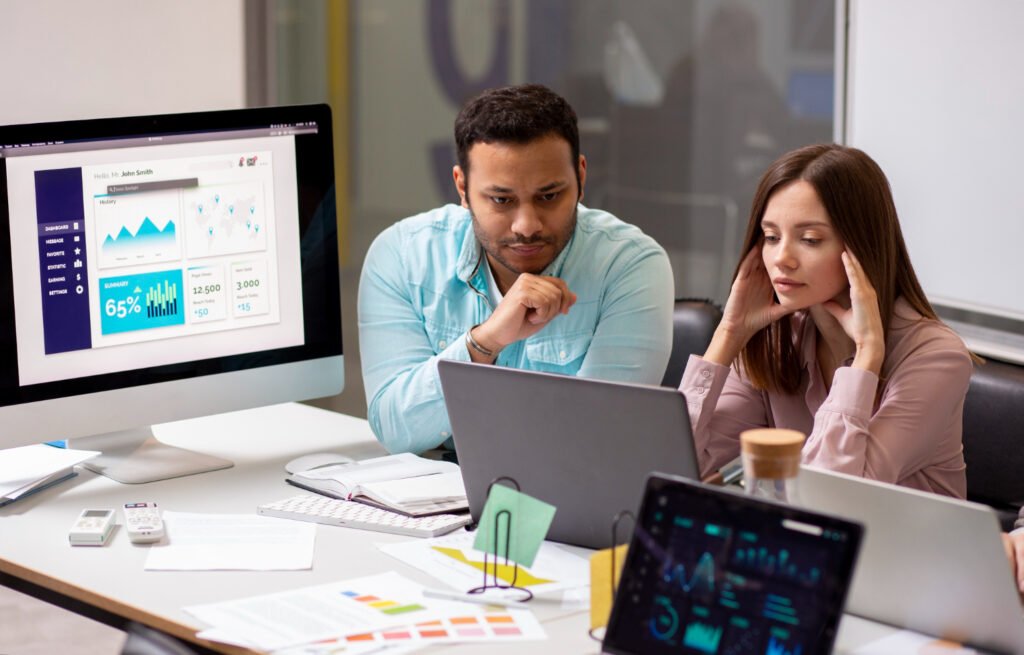
So, let’s know the pointer in C programming.
What is a pointer in C?
A pointer in C language is a variable memory address location checker or variable memory address holder. It stores the memory address of a variable declared in another program in a storage location. Instead of holding a pointer to a variable value directly in C language, the pointer holds the variable storage address where the used variable value is stored in the memory.
Declaring pointers in C.
To declare a pointer variable in a C program, you use the * (asterisk) star special character operator. Here you must remember that the type of the pointer must match the data type of the variable whose address it will store.
int *ptr1; // declare pointer to integer data type
char *name_ptr; // pointer to declare as name character
float *salary;
Initializing Pointers in C.
Here, ptr is an integer pointer variable, and name_ptr is a character pointer variable to a character. salary is a float floating pointer variable.
Pointers in a C program can be initialized by pointing to special program variables or memory locations using the address-of operator & ampersand operator.
int decimal = 1;
int *ptr = &decimal; // Here ptr now points to the memory address of &decimal
Accessing values through pointers in C.
In C language, to access the program variable memory value stored at the memory address to which the pointer points, you use the dereference operator * (asterisk) operator.
int value1 = *ptr; // Displays the variable value stored at the memory address that ptr indicates (which is a decimal variable)
Pointer Arithmetic Operations in C.
Pointer data types in C programs can be operated by increment or decrement operator to point to the next or previous memory address of their data type.
int arrayvalue[6] = {5, 9, 4, 2, 1,3 };
int *ptr = arrayvalue; // set pointer to the first element of the array
printf(“\n %d”, *ptr); // Output – 5 (display array element value at first element)
ptr++; // ptr++ increment operator pointer move to the next element in array
printf(“\n %d”, *ptr); // Output – 9 (display array value at second element in list)
Void Pointer in C.
Void pointer is a pointer type in C language which does not indicate any memory address of the variable declared in the C program. Void pointer is often used to indicate that the pointer is not currently pointing to any valid object.
int *ptr = NULL; // declare ptr to a null pointer type
Pointer and Array in C.
Array data type in C language is very much related to pointer. When you use array data type variable name without index in C, it acts as a pointer address for the first data element of the array.
int arrayelement[5] = {9 , 8, 4, 2, 6 };
int *ptr = arrayelement; // ptr int variable points to the first element of the arrayelement
printf(“\n %d”, *ptr); // Output – 9 (display the first element in array)
ptr++; // ++ operator move to next array element in array series
printf(“\n %d”, *ptr); // Output – 8 (display the second array element)
Pointer and Function in C.
Pointer operator is commonly used in C functions to pass program variable arguments via call by reference. So that the function can modify the original function variable.
void incvalue(int *ptr) {
(*ptr)++; // use ptr operator to Increment the value to the address ptr points to location
}
int main() {
int decimal = 1;
incvalue(&decimal); // it use to pass the address of decimal to the function
printf(“\n Increase the value with increment operator – %d”, decimal);
// Output – Incremented value – 2
return 0;
}
Dynamic Memory Allocation in C.
In C language, dynamic memory allocation is done using built-in functions like pointers operators malloc(), calloc(), and realloc() which allocate memory for variables at program runtime.
int *ptr = malloc(sizeof(int)); // malloc function used to allocate memory for an integer variable
*ptr = 2; // now assign a value to the allocated memory
// free function free the allocated memory when not needed in allocated variable
free(ptr);
Pointer to Pointer (Double Pointer) in C.
In a C program, a pointer indicates another pointer. Which is called double pointer or pointer to pointer operation. Which indirectly creates a series of pointers.
int decimal = 1;
int *ptr = &decimal;
int **ptr_to_ptr = &ptr;
printf(“\n %d”, **ptr_to_ptr); // Output – 1 (display the value at the address stored in ptr variable)
Conclusion About Pointers.
To master program variable addresses in C programming, it is important for you to understand pointers. Because pointers enable us to do efficient C program memory management, manipulation of data structures and execution of advanced program algorithms. Pointer data types are specially used to deal with any data type variable memory address.