Arithmetic operators
Arithmetic operators in C programming language are used to perform mathematical program operations on programming operands (variables or program values). These operations include adding, subtracting, multiplying, dividing, finding modulus, etc. of program values.
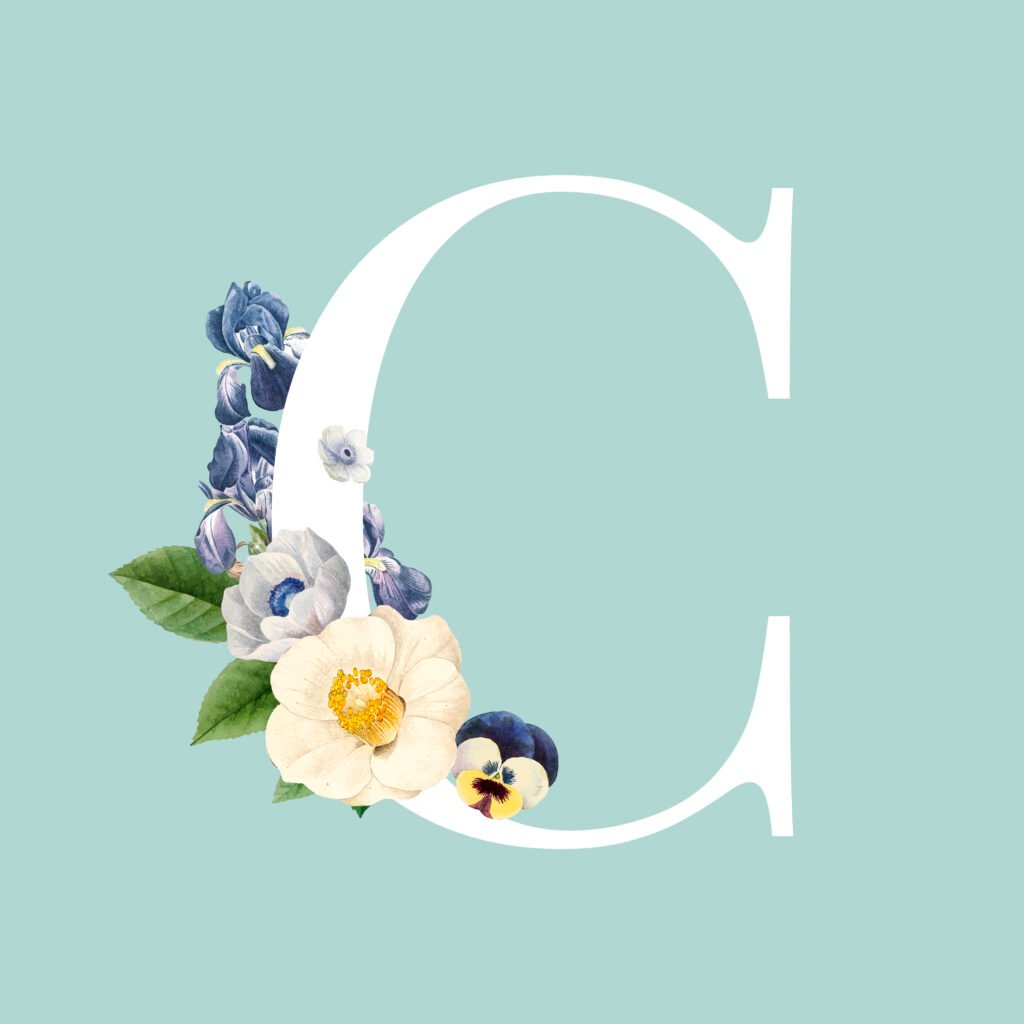
Here are the basic arithmetic operators in C programming.
Addition (+).
Plus operator adds different program variable values or two operands together in a C program.
Addition (+) operator.
int p,q;
sum=1 + 3
output = 1 + 3; // result is 4
Subtraction (-) operator.
Minus arithmetic operator subtracts second operand from first in a C program.
Subtraction (-) Example.
int p,q;
sub=3 – 2
output = 3 – 1 ; // result is 2
Multiplication (*) operator.
The multiplication arithmetic operator is used to multiply two numeric variable operands in C programming.
Multiplication (*) Example.
int p,q;
mul =3 * 2
output = 3 * 2 ; // result is 6
Division (/) Operator.
Division operator in C program declares two different program variable values and returns the remainder or value when the first operand is divided by the second.
division (/) Example.
int p,q;
div =6 / 2
output = 6 * 2 ; // result is 12
Modulus (%) Operator.
Division operator in C program declares two different program variable values and returns the remainder or value when the first operand is divided by the second.
Moduluus (%) Example.
int p,q;
mod = 10 % 3
output = 10 % 3 ; // The result is 1, here you will see the reminder.
Increment (++) operator.
Increases the value of any C program variable value operand by 1.
Increment (++) operator Example.
int p = 1;
p++; // The new value of p is now 2
Decrement (–) operator.
Decreases the value of any C program variable value operand by 1.
Decrement (–) operator Example.
int q = 3;
q–; // The new value of q is now 2
Arithmetic operators.
Arithmetic operators in C programming can be used to manage mathematical functions or operations with program variables or constant values.
Special brackets can be used to control the order of arithmetic operations.
Be aware of integer division in C, where the fractional part is truncated.
The modulus operator does not apply to floating-point or float numbers.
Arithmetic operators are fundamental in C programming as well as other programming languages for performing mathematical calculations and manipulating numerical data.