Error handling in c hindi
सी प्रोग्रामिंग फाइल हैंडलिंग एरर मैनेजमेंट में किसी C प्रोग्राम में फ़ाइल ऑपरेशन के दौरान होने वाले पर्टिक्युअलर फाइल एरर को मैनेज और कण्ट्रोल किया जाता है. एरर मैनेजमेंट से फाइल ऑपरेशन के दौरान होने वाले एरर का पता किया जाता है. कई बार फाइल को ओपन करते समय फाइल ओपन, रीड, राइट, अपेण्ड, ऑपरेशन में होने वाली विफलताओं की एरर को मैनेज कर सकते है.
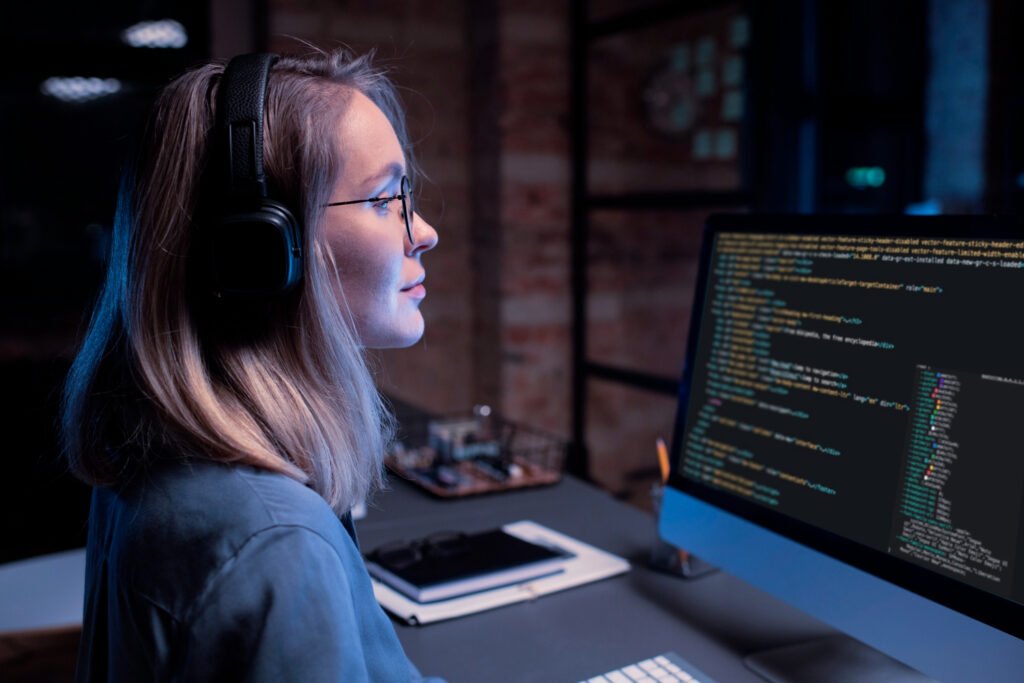
So let’s understand error management in C Programming File Handling in a better way.
जयादातर समय में जब हमें फ़ाइल हैंडलिंग ऑपरेशन को करते समय ध्यान रखना होगा। की आपके द्वारा उपयोग किये जाने वाले फाइल टास्क जैसे, fopen(), fclose(), fread(), और fwrite() जैसे फ़ंक्शन के रिटर्न वैल्यू को टेस्ट करे की फाइल ऑपरेशन में वो सफल या विफल हुए है.
File opening error example in C program.
यदि आप मौजूदा प्रोग्राम में किसी फाइल को रीड मोड में ओपन करते है. और किसी कारणवश आप फाइल को ओपन नहीं कर सकते है. तो इस कंडीशन में fopen() फ़ंक्शन NULL वैल्यू को रिटर्न करता है।
FILE *file = fopen(“test.txt”, “r”); // open file in read mode purpose
if (file == NULL) {
perror(“you face Error opening file operation “);
return 1;
}
Closing the file in file handling.
हमेशा याद रखे जब आप फाइल हैंडलिंग प्रोग्राम में किसी फाइल को ओपन करते है. और जब फाइल ऑपरेशन पूरा हो जाता है, तब fclose() फ़ंक्शन सफल होने पर 0 और विफल होने पर EOF(end of file) वैल्यू को रिटर्न करता है।
if (fclose(file) != 0) {
perror(“you face Error when closing file”);
return 1;
}
Reading data from file in file handling.
फाइल हैंडलिंग में किसी फाइल से डाटा पढ़ने के लिए fread() फंक्शन का उपयोग करते है। fread() फाइल में डाटा और इनफार्मेशन को रीड करता है. यदि यह फाइल रीडिंग में कोई फाल्स वैल्यू प्रोडूस होती है, तो इस कंडीशन में एरर या फ़ाइल क्लोज़ कंडीशन बन सकती है।
size_t output = fread(buffer, sizeof(char), sizeof(buffer), file);
if (result != sizeof(buffer)) {
if (feof(file)) {
printf(“the cursor move End of file reached.\n”);
} else if (ferror(file)) {
perror(“otherwise Error reading file process”);
}
}
Writing to file in file handling.
यदि आप मौजूदा फाइल में कुछ टेक्स्ट या इनफार्मेशन लिखना चाहते है, तो fwrite() फ़ंक्शन का उपयोग करते है, यह फंक्शन फाइल में मौजूदा नंबर्स ऑफ़ करैक्टर वैल्यू इनफार्मेशन को रिटर्न करता है। यहाँ यदि यह कमांड/कोड रिक्वेस्ट वैल्यूज से कम वैल्यू को रिटर्न करता है, तो फाइल राइटिंग प्रोसेस में एरर उत्पन्न होती है।
size_t output = fwrite(buffer, sizeof(char), sizeof(buffer), file);
if (output != sizeof(buffer)) {
perror(“you face Error when writing to the file”);
return 1;
}
Error Handling Example of File Handling in C Programming.
यहाँ आपको एक फाइल हैंडलिंग उदाहरण मिलता है. जो फ़ाइल हैंडलिंग में फाइल को खोलने, फाइल को पढ़ने, फाइल में लिखने और फाइल को बंद करने में होने वाले एरर मैनेजमेंट कोड को प्रदर्शित करता है।
#include <stdio.h>
#include <stdlib.h>
int main() {
FILE *file;
// lets Open a file for writing operation
file = fopen(“test.txt”, “w”);
if (file == NULL) {
perror(“you can face Error opening file for writing operation”);
return 1;
}
// lets Write to the content of the file
const char *info = “file, handling \n lets test the file operation\n”;
size_t output = fwrite(text, sizeof(char), strlen(info), file);
if (output != strlen(info)) {
perror(“it cause Error writing to the file”);
fclose(file);
return 1;
}
// Close the file
if (fclose(file) != 0) {
perror(“if any Error closing file after writing to file”);
return 1;
}
// lets Open the file for reading operation
file = fopen(“test.txt”, “r”);
if (file == NULL) {
perror(“you see the Error when opening file for reading purpose”);
return 1;
}
// lets finally Read and print the content of the test file
char value[256];
while (fgets(value, sizeof(value), file) != NULL) {
printf(“%s”, value);
}
if (ferror(file)) {
perror(“you see the error reading from file”);
fclose(file);
return 1;
}
// lets Close the file
if (fclose(file) != 0) {
perror(“you see the error closing file after the file reading”);
return 1;
}
return 0;
}
File handling library functions used in error management.
Opening a file – fopen() फंक्शन का उपयोग फाइल ऑपरेशन में फ़ाइल को लिखने और पढ़ने के लिए और फाइल को खोलने के लिए किया जाता है। यदि fopen() ऑपरेशन विफल हो जाता है, तो perror() एक एरर मैसेज प्रिंट करता है, और प्रोग्राम तुरंत एग्जिट हो जाता है।
Writing text to a file – fwrite() फंक्शन फाइल ऑपरेशन के दौरान किसी फाइल फ़ाइल में डेटा और इनफार्मेशन को लिखता है। यदि यह यूजर की अपेक्षा से कम बाइट्स इन्फो को लिखता है, तो एक एरर मैसेज प्रिंट करता है, फ़ाइल राइटिंग प्रोसेस बंद हो जाती है, और प्रोग्राम तुरंत एग्जिट हो जाता है।
Reading data from a file – फाइल हैंडलिंग प्रोसेस में fgets() फंक्शन फ़ाइल से टेक्स्ट लाइन्स को पढ़ता है। यदि फाइल लाइन्स रीडिंग में कोई त्रुटि होती है, तो ferror() एक एरर मैसेज प्रिंट करता है, और फ़ाइल तुरंत एग्जिट हो जाती है.
Closing a file – fclose() फंक्शन का उपयोग फाइल हैंडलिंग में फ़ाइल को बंद करने के में होता है। यदि यह फाइल ऑपरेशन्स विफल हो जाता है, तो एक एरर मैसेज प्रिंट करता है और प्रोग्राम तुरंत एग्जिट हो जाता है।