Dictionaries in Python In Hindi
पायथन प्रोग्रामिंग में डिक्शनरी (dict) डाटा टाइप एक पॉवरफुल डेटा स्टोरेज एलिमेंट स्ट्रक्चर है. जो पाइथन प्रोग्रामर को की-पेअर आर्डर में डिक्लेअर डिक्शनरी डेटा एलिमेंट को स्टोर करने में सहायता प्रदान करता है। याद रहे पाइथन डिक्शनरी डाटा टाइप परिवर्तनीय होते है, जहा डिक्शनरी ऑब्जेक्ट्स के अनऑर्डर कलेक्शन हैं. जहाँ प्रत्येक पाइथन प्रोग्राम में डिक्लेअर डिक्शनरी आइटम को की-पेअर आर्डर में स्टोर और प्रोसेस किया जाता है।
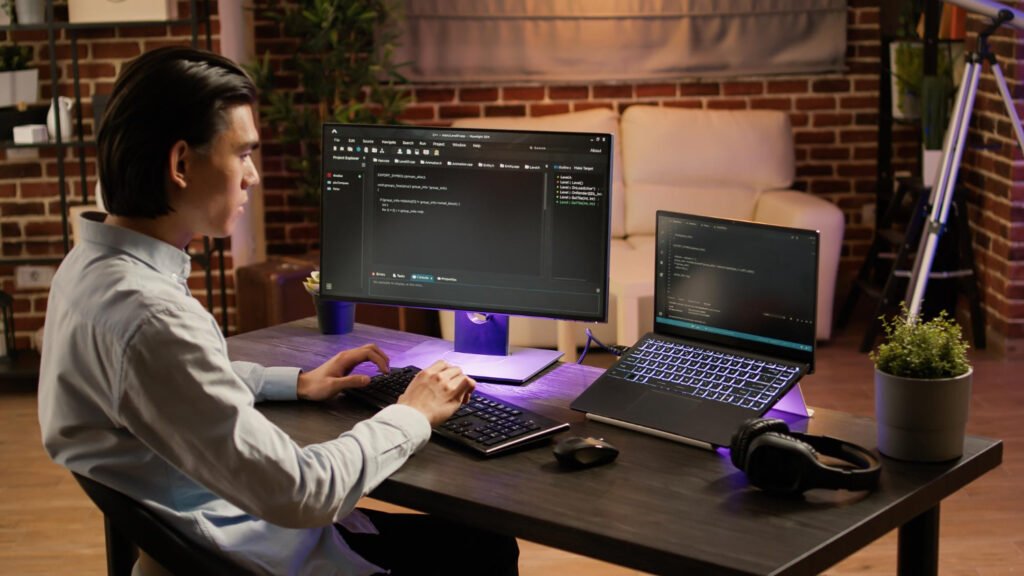
So let’s understand the dictionary data type better in Python programming.
Creating a dictionary data element in Python.
यदि आप पाइथन प्रोग्राम में डिक्शनरी डाटा टाइप को डिक्लेअर करना चाहते है. तो आपको कॉमा ऑपरेटर के साथ अलग अलग डिक्शनरी डाटा एलिमेंट को की-पेअर कॉम्बिनेशन में कर्ली ब्रेसिज़ {} के साथ संलग्न करके एक डिक्शनरी डाटा क्रिएट कर सकते हैं।
# let Creating an no element dictionary in Python
sample_dict = {}
# let create a dictionary element with its key-pair element
clouth = {
“shirts”: 9,
“t shirts”: 8,
“trowser”: 11
}
# let create a python dictionary data type with multiple combination of data values
employee = {
“emp_name”: “ajit”,
“emp_age”: 45,
“salary”: 999.00
}
Basic python dictionary example.
# let create a dictionary element with its key-pair element
clouth = {
“shirts”: 9,
“t shirts”: 8,
“trowser”: 11
}
# let create a python dictionary data type with multiple combination of data values
employee = {
“emp_name”: “ajit”,
“emp_age”: 45,
“salary”: 999.00
}
print(clouth[“shirts”]) # the result is – 9
print(clouth[“trowser”]) # the result is – 11
print(employee[“emp_name”]) # the result is – ajit
print(employee[“salary”]) # the result is – 999.0
Accessing Python Dictionary Elements.
आप पाइथन प्रोग्राम में स्क्वायर ब्रैकेट [] और डिक्शनरी की का उपयोग करके पाइथन प्रोग्राम में डिक्लेअर डिक्शनरी में की से जुड़े वैल्यूज को एक्सेस कर सकते हैं।
# Accessing values by Python dictionary data key
# let use Python dictionary data Accessing values by its key value
print(clouth[“shirts”]) # the result is – 9
print(employee[“emp_name”]) # the result is – ajit
यदि डिक्शनरी की मौजूद नहीं है, तो डिक्शनरी डाटा को एक्सेस करने पर की एरर उत्पन्न होगी। इससे बचने के लिए, आप पाइथन get() मेथड का उपयोग कर सकते हैं, जो आपको डिक्शनरी डाटा की न मिलने पर डिफ़ॉल्ट वैल्यूज को निर्दिष्ट करने की अनुमति देता है.
print(clouth.get(“shirts”, 0))
print(clouth.get(“trowser”, 0))
Modifying Python dictionary data.
पाइथन प्रोग्राम में डिक्लेअर डिक्शनरी डाटा टाइप परिवर्तनशील होते हैं, इसलिए आप अपनी डिक्शनरी कीस को नए वैल्यूज डिफाइन करके, नए की वैल्यूज पेअर जोड़कर या मौजूदा की वैल्यूज ऐड या डिलीट कर मौजूदा डिक्शनरी एलिमेंट्स को मॉडिफाई कर सकते हैं।
# let Modifying dictionary existing element values
clouth[“shirts”] = 13
print(clouth) # the resut is – {‘shirts’: 13, ‘t shirts’: 8, ‘trowser’: 11}
# let Adding or deleting new key-values to the existing dictionary
clouth[“vimal shirt”] = 15
print(clouth) # the resut is – {‘shirts’: 13, ‘t shirts’: 8, ‘trowser’: 11, ‘vimal shirt’: 15}
# let Deleting Python dictionary data element key-value pairs
del clouth[“trowser”]
print(clouth) # the resut is – {‘shirts’: 13, ‘t shirts’: 8, ‘vimal shirt’: 15}
Python dictionary operations.
पाइथन प्रोग्राम में आप डिक्शनरी पर विभिन्न प्रोग्रामिंग ऑपरेशन्स कर सकते हैं, जैसे मेंबर्स (in) की जाँच करना, कीस, वैल्यूज या आइटमों पर रेपीटीशन करना और लंबाई (len()) को पता करना आदि है।
# let check member in Python dictionary member
print(“shirts” in clouth) # the result is – true
print(“trowser” in clouth) # the result is – true
# let repeatition over Python dictionary keys values
for key in clouth:
print(key)
# let repeat over Python dictionary values
for value in clouth.value():
print(value)
# let repeat on move over Python dictionary key-value pairs
for key, value in clouth.items():
print(key, value)
# let findout the Length of Python dictionary element
print(len(clouth))
Python Dictionary Comprehension.
पाइथन प्रोग्रामिंग में लिस्ट कॉम्प्रिहेंशन के समान, आप पाइथन प्रोग्राम में डिक्शनरी कॉम्प्रिहेंशन का उपयोग करके डिक्शनरी डाटा एलिमेंट को क्रिएट कर सकते हैं।
# let Create a dictionary of square root of numbers from 2 to 10
sqrt = {p: p**2 for p in range(2, 10)}
print(sqrt) # let teh result is – {2: 4, 3: 9, 4: 16, 5: 25, 6: 36, 7: 49, 8: 64, 9: 81}
Python nested dictionaries.
पाइथन प्रोग्राम में डिक्शनरी के अंदर अन्य डिक्शनरी वैल्यूज के रूप में शामिल किया जा सकता है, जिससे आप नेस्टेड डिक्शनरी डेटा स्ट्रक्चर बना सकते हैं।
# let create Python Nested Dictionary
developer = {
“lalit”: {“age”:40, “web developer”: “forntend developer”},
“ajit”: {“age”:39, “python developer”: “backend developer”}
}
print(developer[“lalit”]) # the result is – {‘age’: 40, ‘web developer’: ‘forntend developer’}
print(developer[“ajit”]) # the result is – {‘age’: 39, ‘python developer’: ‘backend developer’}
Use of Dictionaries in Python.
Dictionary Mapping – जब आपको पाइथन प्रोग्राम में कीस को कम्पेटिबल वैल्यूज से मैप करने की आवश्यकता हो, तो डिक्शनरी डाटा टाइप एलिमेंट का उपयोग कर सकते है।
Dictionaries Faster Lookup – पाइथन प्रोग्राम में डिक्शनरी कीस के आधार पर डिक्शनरी वैल्यूज तक पहुँचने के लिए फ़ास्ट लुकअप समय फीचर्स प्रोवाइड करती हैं।
Dictionaries Flexible Data Structure – पाइथन प्रोग्रामिंग में स्ट्रक्चर डेटा के लिए डिक्शनरी डाटा टाइप का ही उपयोग करें। जहाँ स्टोर डिक्शनरी डाटा इनफार्मेशन का प्रत्येक भाग एक यूनिक कुंजी से जुड़ा होता है।