What is ord python
The ord() function is an integrated function in the Python programming language. returns the provided character’s assci character value or Unicode code. A numerical value is a Unicode code point. This, according to the Unicode standard, symbolizes a character in a particular alphabet. This is the character recognition encoding scheme. It accommodates a large selection of characters from several keyboard writing systems.
Programmers only need to provide one argument to the Ord() method. This is the character whose Unicode code points you wish to get. Both ASCII and non-ASCII character actions may be performed with it.
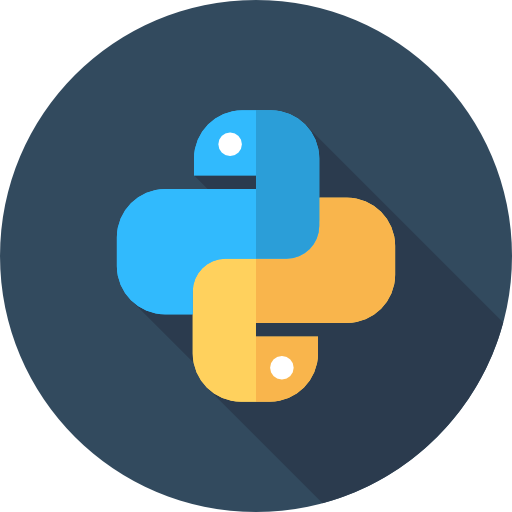
what and how to python
Here is an example of how to use the Ord() function.
Syntax
char = ‘a’
char1 = ‘B’
first_ord_char = ord(char)
first_ord_char1 = ord(char1)
print(“\n”,first_ord_char)
print(“\n”,first_ord_char1)
symbol = ‘$’
symbol_ord = ord(symbol)
print(“\n”,symbol_ord)
What is numpy in python
A potent array library for Python is called NumPy (Numerical Python). It offers a broad variety of mathematical operations to programmers, as well as assistance with single arrays, multi-dimensional arrays, and matrices, to operate on arrays effectively. A different third-party package is called numpy. It is frequently used in tasks involving mathematical arrays, machine learning, and scientific computing.
How to import numpy in python programming
import numpy as np
Program Example
import numpy as np
# declare a one-dimensional NumPy array
array = np.array([12, 11, 34, 25, 66])
print(“\n”,array)
How to run python script
To run a script, just comply with these guidelines.
- You must first create or open a text file in which to enter the script program code. Save the script as test.py, for instance, and give it the.py extension. Ensure that the file contains only valid Program code.
- Launch a terminal or command-line interface on your computer.
- Open the directory in which the script is stored. To modify the path to a directory, use the cd command.
- Run the script by entering and the name of the test file once you have navigated to the relevant directory.
Example
python test.py
f Python 2 and Python 3 are both installed. To execute this script with Python 3, you might thus need to provide Python3 rather than Python2.
Python 3.0 test
Your Program test file will now run when you press the Enter key to execute the command. The terminal will show the output of the application. if no software fault happens.
What is pip python
Pip is a Python package manager for installing libraries or modules. It is a command-line utility for cui. which enables you to install, upgrade, and manage packages for Python from the available package repositories, including the Professional Essential Package Index (PEPI). Installing third-party libraries and dependencies in the environment for development is made easier by Pip. Those are absent from the standard libraries installed by default.
Python’s pip makes it simple to install packages when you need them by naming them or giving the package’s URL. If you wish to install a package in your current environment, it resolves any dependencies and installs them. Additionally, PIP offers a number of tools for managing packages, removing already installed packages, and updating packages.
How to read a text file in python
Python’s built-in open() file read function and other file handling techniques can be used to read an existing text file.
Here is an example of the file open function.
Syntax
# Open the below text file in read-only mode
file = open(“test.txt”, “r”)
# Read the test.txt file contents from the file
contents = file.read()
# Close the above open test.text file
file.close()
# Print the existing contents in the test.txt file
print(“\n”,contents)
The open() function is used to open the file “example.txt” in read mode (“r”) in the code snippet above. Change “example.txt” to the location of your text file. You may use the read() method to read a file’s whole contents as a string after opening it.
What is a variable in python
A variable is a named reference to a value of a stated program data type that is kept in computer memory in Python applications. During the execution of a program, variables are used to store and control the value of data. Program variables serve as data storage spaces for many kinds of values. Declare integers, texts, lists, or other objects and data types much like.
when a variable is declared. So, you use the operator assignment (=) to give a variable a value. Any valid data type may be used as the value.
Here is one instance.
Declaration of several variables
# Assigning multiple values to a different variable
name = “David”
contact = 12345678910
weight = 75.10
is_id = True
In the example above, the variable name stores the string “david”, contact stores the integer 12345678910, weight stores the float 75.10 data type variable, and is_id stores and represents the boolean value true.
How to run a python script
if you wish to execute a Python application or script. Thus, you can carry out these actions.
- Create a new Python program file with myfile.py extensions, such as myfile.py, to turn it into a script. To create Program software scripts, you can use any text editor or integrated development environment (IDE).
- Put the code you’re using in the script file. ensuring that it uses proper syntax.
- Launch a terminal or command-line interface on your computer.
- Locate the directory containing the application script using the cd command (change directory).
- When you are in the right directory, you may launch the script by entering and the script’s file name.
example.
python myfile.py
To execute the script with Python 3 if you have both Python 2 and Python 3 installed, you might need to provide python3 instead of python.
python 3 code
python3 myfile.py
Once you’ve entered the command, your application script will begin to run. If the Python logic code is right, the output, print, will be seen on the terminal.
What is self in python
A common name in Python programming is self. It is used in method declarations within a class as the first parameter. It refers to the class instance that the method is being called on. Self is often referred to as a noun. However, you are free to give this parameter any name.
The methods inside a class that is defined in a program often accept self as the first parameter. It enables methods to interact with other methods, instance variables, and variables specific to that instance.
How to learn python
Python programming may be exciting for you to learn. For new programmers, the following stages and resources are provided. It might assist you in beginning and continuing your study of programming.
- You should first be familiar with the fundamentals of programming.
- Enroll in current online courses.
- For further information, thoroughly read the documentation.
- To better grasp, practice writing simple code.
- Participate in broader communities online.
- Create some easy-to-understand projects.
- Consult superior advanced books.
- Attend offline events and meetings online.
- Participate in or contribute to the open-source community.
- Continue learning over time if you want to master it.
What is a method in python
A method is a built-in function when programming in Python. which is a method that is attached to an instance or object of a class that is declared within a declare class. Methods are used to describe an object’s behavior and carry out particular tasks or computations that are part of the class.
Like functions, methods in may access the attributes and other methods of an object and are tied to them. they are called with dot notation. Any method name is followed by an object instance.
Python how to call a function
parenthesis () are added after the function name to call the function. You can write or provide parameters inside parentheses if the function calls for them.
This is a straightforward illustration of how to call a straightforward function without any parameters.
Syntax
def welcome(): # create welcome function
print(“Welcome to Vcanhelpsu \n”)
# Calling the welcome function
welcome()
In the above program code, the welcome() function is defined. which simply prints “Welcome to Vcanhelpsu” to the console. To call a function, you just write welcome().
What does // do in python
The floor division operation is carried out through Python’s // operator. Likewise known as integer division. The greatest integer value is returned by this defined variable after dividing the left operand by the right operand. which falls below or holds the same value as the quotient.
This serves as an illustration of how to utilize the // operator.
example
# Floor division example
floor_div = 27 // 3
print(“\n”,floor_div) # print floor dive values
print(29 // 2) # print 29 floor division
In the example above, 27 // 3 does floor division, dividing 27 by 3. The result is 9 because the greatest integer less than or equal to the quotient of 29 divided by 3 gives 9.
What is a constructor in python
A constructor is a unique declaration method inside a class in Python programming. which, when an object of that class is generated in the existing program, is automatically invoked. It is used to conduct any extra setup tasks necessary for the object, such as initializing the attributes of a class object.
The constructor method is called __init__(), which means initialize.
What is s in python
The variable name s is not reserved or specified in the programming language. Simply said, it is an identifier that may be used to stand in for a program variable. The context in which a variable is used in a program determines its meaning and function.
S is frequently used as a universal standard to represent a string variable in numerous program code examples and programming jobs.
Example
text=”Vcanhelpsu”
s = “Welcome to Vcanhelps”
print(“\n Simple String output -“,text)
print(“\n Default Store String Value -“,s)
In the above example, s is used to store the string value “Welcome to Vcanhelps”. And later it is printed on the screen or console.
How long does it take to learn python
The length of time needed to learn Python programming might differ significantly based on a number of variables. Include any past programming experience you may have, how much time you have spent learning programming, how well you comprehend program logic, and the goals you have for your code.
Here are a few general things to think about when learning Python programming.
- Prior programming experience is required.
- Begin studying methodologies and resources.
- programming time commitments must be adhered to precisely.
- You must have a thorough comprehension of the logic, code, conditions, statements, and other elements of programming.
How to call a function in python
you may primarily follow these steps to call a function.
Define a Python Function – a function is first defined by using the def keyword, followed by the function name and any necessary function parameters in parentheses ().
Call a function – you may call a defined function by writing its name followed by parentheses (), which will run the function’s code.
def div_numbers(p, q):
return p / q
div = div_numbers(10, 2)
print(“\n Function call Result is -“,div)
How to install python package
You use a package manager called pip to add packages to existing Python code. It also serves as the default package installer for the development environment.
The procedures for utilizing pip in the Development Environment are listed below.
- Open a command prompt or terminal – Depending on the operating system you are currently using, open a command prompt (Windows) or a terminal (MacOS/Linux).
- Verify pip’s installation – Enter the following command to see if pip is already installed on your computer.
syntax
pip-version
If pip is set up, the current version number will be shown. If not, you must first install the first pip on your own. If pip isn’t already set up, you can follow the directions in the official Python manual or on the internet.
- Install Packages – Run the command below to install packages on your machine.
syntax
pip install package_name
the replace package_name with the name of the package you wish to install. For instance, you would run to install the requests package.
- Verify the installation – Verify the installation by importing the package in your Program code when it has been completed. Example.
syntax
import package_name
If no errors with the software arise, the installation of the package was successful.
How to install package in python
The package manager pip, which is the default tool for installing Python packages, may be used to install a package.
How to install python
Depending on your operating system, Python programming must be installed.
The general procedures for installing Python on different systems are shown below.
Microsoft Windows.
- First, go to https://www.python.org/downloads/, the official website.
- To manually download the most recent stable version, click the “Download Python” button on the download page.
- Depending on your system architecture (32-bit or 64-bit), scroll down and choose the appropriate installer file.
- Now launch the computer’s downloaded installer.
- Make sure to check the “Add Python to PATH” box in the Python installation wizard before selecting the “Install now” option to begin the installation.
- Python will be manually installed by the installer, and the necessary Python environment variables will be configured.
- Once Python has been installed, you may check its version and installation status by launching a command line and entering the command Python –version.
Apple MacOS.
- Python programming is often pre-installed on the macOS operating system.
- Launch a Mac Terminal window.
- Type Python3 –version to see if Python is already installed on your current version of macOS. Continue to the next stage if it isn’t.
- Visit the download page at https://www.python.org/downloads.
- For the most recent stable version of Python, manually click the “Download Python” button on the download page.
- Select the macOS Python installer package by scrolling to the bottom.
- Run the Python installer for Mac that you downloaded.
- To finish the installation, comply to the installer’s instructions.
- To confirm that it is installed and to see the version, open a terminal when the installation is finished, and run python3 –version.
Linux Operating System.
- In a Linux operating system, open a terminal window.
- Type python3 –version to see if it is already installed. Continue to the next stage if it isn’t.
- Utilize the package manager for your particular Linux system to install Python. You may use the following command, for instance, on computers running Ubuntu or Debian.
- sudo apt-get install Python3
- The following commands can be used on computers running Fedora or Red Hat.
- sudo dnf install python3
- Depending on your Linux distribution, the actual command may be different.
- When the installation is finished, you can check Python’s version and installation status by typing python3 –version in the terminal.
- After installing it, you may use it by running scripts, entering commands into the interpreter, or developing with IDEs and text editors.
How to comment out multiple lines in python
Use multi-line comments or individual line comments to comment out numerous lines in an application. Here, there are basically two methods.
Multi-Line Comments – Unlike some other programming languages, it does not provide a built-in syntax for multi-line comments. A multi-line string can, however, be used as a comment, and the interpreter will disregard it up to that point. prior to being used in any form or assigned to a variable.
Here is one instance.
comment syntax
print(“\n Pythons is the best for development”) # this is the single line comment
# bellow text display clear example of multiline comment
“””
below text display some text
print text info
“””
print(“\n Welcome to vcanhelpsu”)
How to check python version
You may mostly follow these methods to determine which version of currently installed on your current operating system.
Open the Command Prompt (Windows) or Terminal (MacOS/Linux) program, depending on your operating system.
Enter the command by typing it in the box provided.
check pythons version.
python –version
How to print a list in Python
The print() method may be used to print a list.
These are several techniques for printing a list.
Print the entire list.
Syntax
python_list = [44, 66, 22, 99, 100]
print(“\n”,python_list)
How to reverse a string in python
To reverse a string, you can use string slicing.
this is an example.
Syntax
text = “vcanhelpsu”
rev_text = text[::-1]
print(“\n”,rev_text)
How to get the length of a string in python stack overflow
To get the length of a string, you can use the built-in len() function.
This is an example.
Syntax
text = “Welcome to Vcanhelpsu”
text_length = len(text) # length function
print(“\n”,text_length)
The length of the text string is determined in this example using the len() function. The variable length is then given the returned length, after which it is printed.
The len() method calculates a text’s total character count, including spaces and punctuation.
How to comment in python
The # symbol can be used to insert a single-line comment. The Program interpreter interprets any text on the same line that comes after the # sign as a comment and ignores it.
This is an example.
Syntax
# This is a single-line comment
print(“welcome to pythons\n”) # This is single line comment
Use triple quotes (“”” and ”’) to generate a multi-line string if you need to make a multi-line comment or comment out numerous lines of code. If the multi-line text is not set to a variable or utilized in any other manner, it considers it as a comment.
Here’s an illustration.
multiline
“””
this is an example of a multiline comment
you can continue to add info about any object with multi-line comment
“””
print(“welcome to vcanhelpsu\n”)
How to use python
If you wish to program in Python. So you must adhere to these fundamental guidelines.
- Python programming must first be installed.
- Create a development environment.
- Compose your first line of Program code.
- Learn how to code.
- Save the Program code you currently have.
- Run the program code for your running program.
- View the output of a program application.
What is parsing in python
Parsing is a programming term that describes the process of analyzing and deciphering structured data, such as text or files, to extract certain information or to carry out various programming operations based on the structure and content of the data. For this kind of activity, it offers a number of tools and modules that make parsing processes easier.
These are some typical Python programming use cases and associate parsing tools.
- The ability to parse HTML, XML, and JSON.
- CSV parsing.
- Text file and command-line parameter parsing.
How to import modules in python
you can import modules using the import statement.
Here are a few ways to import modules.
Importing an entire module.
python module import
import module_name
This syntax imports the entire module and allows you to access its contents using the module name.
Example
math.sqrt() or math.pi. # it import math module
How to update python
If you wish to switch from an older version of Python programming. Therefore, you may generally stick to these guidelines.
- Check the Python version you are using – Enter the following command into the command prompt or terminal that is open on your computer.
Check current python version
The version that is currently installed on your system will be shown by this command.
- Go to the Python website – Go to the Downloads area of the Python website at https://www.python.org.
- Download the most recent Python installation from this page – where you can also discover the installer for your operating system and download the most recent stable release of the Python programming language.
- Run the installer after downloading it – then follow the tutorial or instructions for installing that appear on the screen. Make sure to choose the option to upgrade or install right away rather than starting from scratch.
- Verify the installation – by entering the following command into a new command prompt or terminal window once Python has been successfully installed on your machine.
Check Active Installed Python Version
python-version
This will confirm that your computer’s Python installation is the most recent version.
How to round in python
The built-in round() method may be used to round integers to a certain number of decimal places.
This is an example.
Syntax
p = 10.9912
round_numbers = round(p, 3)
print(“\n”,round_numbers)
How to install python on mac
If you want your macOS operating system to support Python programming. In general, you can follow these instructions.
- Go to the Python website – First, go to the download part of the official Python website at https://www.python.org/.
- Download the Python installer – The various download versions are listed on the Downloads page. Choose the most recent Python 3.x stable release, such as Python 3.9. To obtain the installation package, click the link for the macOS installer.
- Run the installer – After downloading, double-click the installer package to launch it. A typical macOS Package Installer window will appear. To continue with the installation, adhere to the onscreen directions.
Make sure the “Install Python 3.x” box is checked (where x is the particular version you downloaded).
By selecting the “Customize Installation” button, you may elect to modify the installation. Although you may choose extra features or parts, most users should be OK with the basic installation.
To approve the installation, you might be prompted to provide your macOS user password.
- Check the installation – Launch the Terminal program on your Mac when the installation is finished. In the Terminal, enter the command Python3 –version or Python –version. The installed Python version will be shown. The presence of the version number indicates a successful installation of Python.
Always use Python3 to explicitly launch Python 3.x because Python 2.x may come pre-installed in more recent versions of macOS.
- PATH variable setting (optional) – During installation, Python should by default be added to the system’s PATH variable. This allows Python to be executed anywhere in the terminal. If you experience any issues when executing Python from the terminal. The Python installation directory may thus need to be explicitly added to your PATH. Open Terminal and enter the following command to accomplish this.
echo ‘export PATH=”/usr/local/bin:$PATH”‘ >> ~/.bash_profile
source ~/.bash_profile
This makes the executable available from the terminal by adding /usr/local/bin to the PATH variable.
How to reverse a list in python
you can reverse a list using the built-in reverse() method or by using slicing.
these are the ways to reverse a list.
use the reverse() method.
Syntax
dec_numbers = [20, 19, 18, 17, 16, 15, 14]
dec_numbers.reverse()
print(“\n Decending Reverse Numbers List – “,dec_numbers)
The reverse() method reverses the order of elements in the list in-place, meaning it modifies the original list directly.
How to make a list in python
you can create a list by enclosing comma-separated values inside square brackets [].
These are a few examples of how to make a list.
Creating an empty list.
Creating a list with values.
Syntax
integer = [22, 55, 90, 34, 75]
course = [‘Python’, ‘Java’, ‘Oracle’]
group_list = [‘A’, 100, ‘Java script’, False, 22.7]
print(“\n”)
print(“\n”,integer)
print(“\n”,course)
print(“\n”,group_list)
How to define a function in python
you can define a function using the def keyword followed by the function name, parentheses (), and a colon :.
These are the general syntax for defining a function.
Syntax
def function_name(parameter1, parameter2, …):
# Function body declared
# Statements to be executed when the function is called itself
# Optional return statement in function if any
Understand each component of a function definition in Pythons programming.
- Def – This keyword is used to declare a function definition.
- Function_name – This is the user-defined name you choose for your function. It should follow Python’s naming conventions (use lowercase with underscores for multiple words).
- Parameters – These are optional inputs to the function. You can specify zero or more parameters inside the function parentheses. which are separated by multiple commas. Parameters allow you to pass values to a function for processing.
- :- The colon now indicates the beginning of the function body.
- Function Body – It is the block of code that is executed when the function is called. It consists of one or more statements, which are indented below the def line.
- Return statement (optional) – You can include a return statement in any function body to specify the value to be returned by the function. If no return statement is used. So the function will not return any value as per the default value.
Here is an example of a simple function. which calculates the sum of two numbers.
Syntax
def mul_integer(p, q):
mul = p + q
return mul
In this example, the mul_integer function takes two parameters p and q. It calculates the multiply of p and q and returns the result using the return statement.
How to install python modules
To install modules, you can use the package manager called pip (pip stands for “pip installs packages”).
What is an integer in python
an integer is a numeric data type that represents whole numbers. It can be positive, negative, or zero. Integers have unlimited precision, meaning they can represent arbitrarily large numbers as long as there is enough memory available.
These are a few examples of integers.
integer declaration.
p = 234
q = -90
r = +10
print(“\n”,p)
print(“\n”,q)
print(“\n”,r)