StreamReader and StreamWriter
StreamReader and StreamWriter in C# Programming are built-in file handling operation classes in C#, these file classes are present inbuilt in System.IO namespace, C# programmers use these classes to read and write data and information from existing files or streams in C#. StreamReader and StreamWriter classes help C# programmers to manage read and write text data in file handling, where C# programmers can perform read and write operations from files in existing text and other file formats.
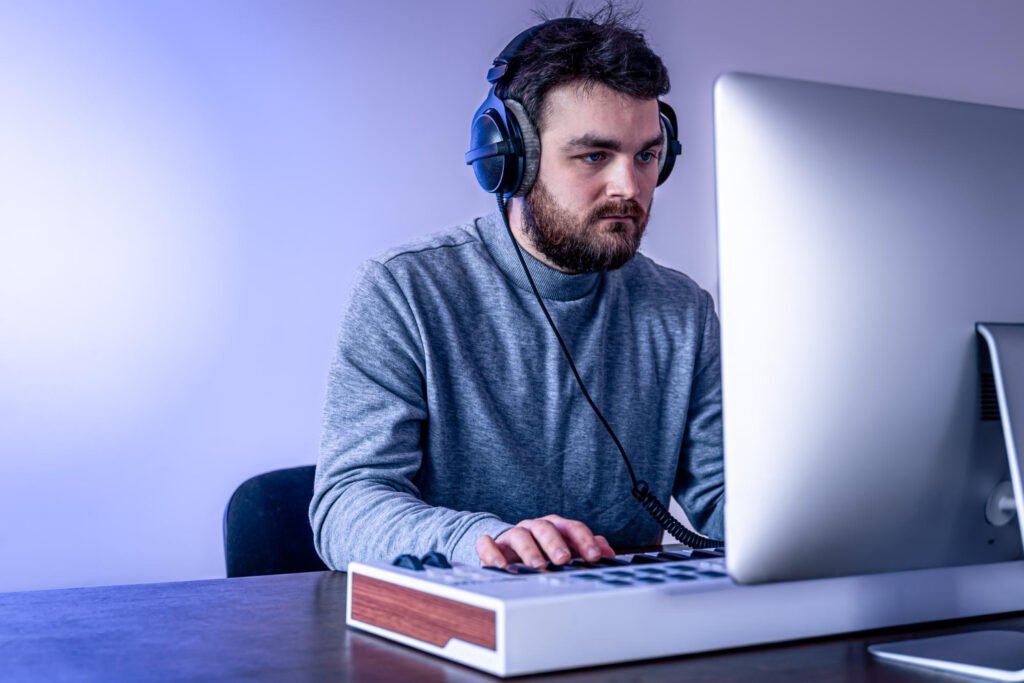
So let’s get to know better about StreamReader and StreamWriter built-in class function in file handling in C# programming.
StreamReader File Handling Function in C# Programming.
StreamReader class in C# Programming is used to read text information from existing file created in C# programming or other user input stream, such as memory location or network stream location. StreamReader class in C# programming reads text file character format in special character encoding format like UTF-8 or ASCII, and helps to efficiently read and customize large text files in C# programming as per the need.
Creating and using StreamReader in C# programming.
So, let’s read file with StreamReader class in C# programming.
using System;
using System.IO;
class Program
{
static void Main()
{
string filePath = @”C:\path\to\your\sample.txt”;
// let Use StreamReader class to read from a file
try
{
using (StreamReader reader = new StreamReader(filePath))
{
string line;
// it Reading line by line till null
while ((line = reader.ReadLine()) != null)
{
Console.WriteLine(line); // it produce Output each line in the file
}
}
}
catch (FileNotFoundException e)
{
Console.WriteLine(“\n Display File Read Error: File not found. ” + e.Message);
}
catch (Exception e)
{
Console.WriteLine(“File An error occurred: ” + e.Message);
}
}
}
Main methods of StreamReader file reading.
- ReadLine() – Reads a row of file text from the active or current location stream in C# program.
- ReadToEnd() – Reads all file characters from the current location to the end of the stream file in C# program.
- Read() – It reads the next character from the stream in the current C# program.
- Peek() – It returns the next character in the current C# program without moving the current location next, it works similar to LookAhead.
Reading all content with ReadToEnd in C# program example.
using System;
using System.IO;
class Program
{
static void Main()
{
string filePath = @”C:\path\to\your\sample.txt”;
try
{
using (StreamReader reader = new StreamReader(filePath))
{
// it will Read the entire content of the active file
string content = reader.ReadToEnd();
Console.WriteLine(content); // it will produce Output entire content of the file
}
}
catch (Exception e)
{
Console.WriteLine(“\n It display An error occurred ” + e.Message);
}
}
}
StreamWriter File Handling Function in C# Programming.
In C# programming, the Stream Writer class is used to write text information to a file or other output stream or file. The main use of the Stream Writer class in C# programming is to create new text files or overwrite existing files, along with this the Stream Writer class is also efficient in appending text information to the existing file or files.
Creating and using the StreamWriter class in C# programming.
So let’s take an example of using the Stream Writer class in C# programming.
using System;
using System.IO;
class Program
{
static void Main()
{
string filePath = @”C:\path\to\your\sample.txt”;
try
{
using (StreamWriter writer = new StreamWriter(filePath))
{
// it will Writing number of below lines to a sample.txt file
writer.WriteLine(“\n Hi, Vcanhelpsu.com”);
writer.WriteLine(“\n Lets Learn About Programming.”);
}
Console.WriteLine(“\n It write in file, when file is new or empty.”);
}
catch (Exception e)
{
Console.WriteLine(“\n file write operation An error occurred ” + e.Message);
}
}
}
Main methods of StreamWriter in C# programming.
- Write(string value) – Writes the particular indicated string to the stream in the C# program.
- WriteLine(string value) – Writes the indicated string to the stream in the C# program, later creates a new line character and writes it.
- Flush() – Clears the buffer memory in the current C# program, and writes any existing file data buffered data to the built-in stream.
- Close() – Closes the StreamWriter class in the current C# program, this file handling function closes all resources associated with the file.
Adding Text to File in C# Program Example.
using System;
using System.IO;
class Program
{
static void Main()
{
string filePath = @”C:\path\to\your\sample.txt”;
try
{
// below statement Open the file in append mode
using (StreamWriter writer = new StreamWriter(filePath, append: true))
{
writer.WriteLine(“\n it will append text of line in existing file.”);
}
Console.WriteLine(“\n The Text will be appended to the file.”);
}
catch (Exception e)
{
Console.WriteLine(“\n The file text append An error occurred ” + e.Message);
}
}
}
Comparison of StreamReader and StreamWriter c# function in file handling
Feature | StreamReader class function | StreamWriter class function |
Purpose | It Reads text from a file or user stream. | It Writes text to a file or user stream. |
Encoding | It will Reads text using specified or default encoding character format. | It will Writes text using specified or default encoding character format. |
Common Methods | ReadLine(), ReadToEnd(), Read() are common method to read from file. | Write(), WriteLine(), Flush() are common method to write in file. |
Access Type | Streamreader class provide only Read-only access | Streamwriter class function allow permission to Write-only access |
Error Handling | You Can apply throw FileNotFoundException or IOException | You Can use throw UnauthorizedAccessException or IOException |
File Operations with StreamReader and StreamWriter Classes in C# Programming.
Read and Write File with Specific Encoding in C# Program.
using System;
using System.IO;
using System.Text;
class Program
{
static void Main()
{
string filePath = @”C:\path\to\your\sample.txt”;
// it will Writing with steamwritee calss in UTF-8 encoding format
using (StreamWriter writer = new StreamWriter(filePath, false, Encoding.UTF8))
{
writer.WriteLine(“\n The line of text is written with UTF-8 encoding character format.”);
}
// it will Reading with UTF-8 encoding character format
using (StreamReader reader = new StreamReader(filePath, Encoding.UTF8))
{
string content = reader.ReadToEnd();
Console.WriteLine(content);
}
}
}
You can indicate the file character encoding while creating StreamReader and StreamWriter classes in your C# program, to ensure that the text is read or written in the correct encoding format.
Handling Exceptions in C# Program.
File Handling in C# Program While working with I/O operations, always manage program exceptions properly, as sometimes failures may occur while accessing the file due to various reasons. For example, while applying file operations, some common file related exceptions are file not found, no permission to access file, disk is full, etc.
FileNotFoundException
UnauthorizedAccessException
IOException
You can use try-catch block to manage the above program exceptions in C# program and check that the file program does not crash accidentally.
StreamReader and StreamWriter in C# Programming Conclusion.
StreamReader in C# program is a perfect class to read text from files, and it supports various class function methods like ReadLine() and ReadToEnd() to let you read text simultaneously in sequence in C# program.
StreamWriter class in C# program is designed to write text to files in file handling, which already has class methods like Write() and WriteLine() to provide write permission to data efficiently in C# program.
The StreamReader and StreamWriter classes in C# programs help programmers work with special character encodings and manage large files efficiently.