Primitive Data Types int, float, double, char, bool, etc.
There are different types of variable declaration method data types in C# programming, like you have learned in C language, C++ programming, Java, Python, etc. C# programming provides you with many types of primitive data types to manage different data types. Mainly integer data type, floating-point numeric data type, character data type and boolean data type are variable values in C# programming.
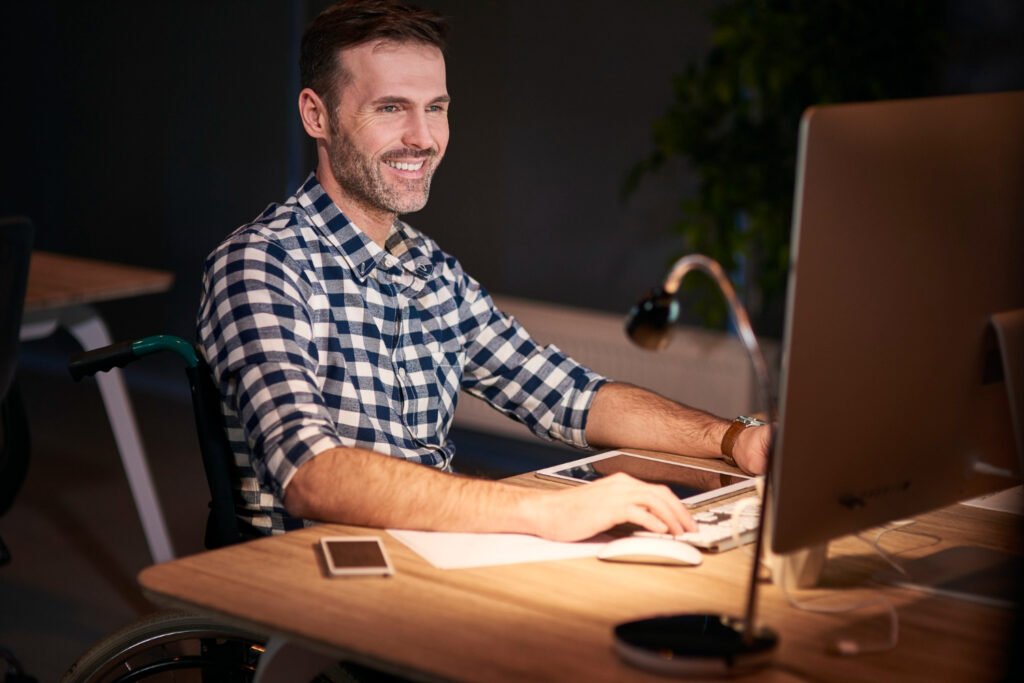
Integer data Types in C# Programming.
Integer data type in C# programming is used to store and process complete integer numeric variable integer values. Integer data type stores complete integer decimal number values.
Data Type | Data Type Size | Data Type Range | Data Type Default Value |
int | 32 bits | -2,147,483,648 to 2,147,483,647 | 0 |
long | 64 bits | -9 quintillion to +9 quintillion | 0L |
short | 16 bits | -32,768 to 32,767 | 0 |
byte | 8 bits | 0 to 255 | 0 |
sbyte | 8 bits | -128 to 127 | 0 |
uint | 32 bits | 0 to 4,294,967,295 | 0 |
ulong | 64 bits | 0 to 18 quintillion | 0L |
ushort | 16 bits | 0 to 65,535 | 0 |
Integer data type example in C# programming.
int contact = 9413;
long millinial = 9700000000L;
byte smallinteger = 255;
Floating-point data types in C# programming.
In C# programming, floating data types are used to store and process fractional parts along with integers and decimal numbers. Floating data type variables store fractional numeric values along with integers.
Data Type | Data Type Size | Precision | Default Value |
float | 32 bits | ~7 digits | 0.0f |
double | 64 bits | ~15 digits | 0.0d |
decimal | 128 bits | ~28-29 digits | 0.0m |
Floating data type examples in C# programming.
float pi_value = 3.14f;
double value = 4.432423423423;
decimal largeinteger = 899228162514264212121543950335m;
Character (char) data type in C# programming.
In C# programming, character data types are used to store and process string text information along with single character array. You can store multiple character arrays as variables in C# programming from single character.
Character Data Size – 16 bits character (Unicode Supports) format.
Char Range – ‘\u0000’ to ‘\uffff’
Char Default value – ‘\0’
Character examples in C# programming.
char text = ‘P’;
char unicodeChar = ‘\u0041’; // it representation of unicode char ‘A’
Boolean data type (bool) in C# programming.
In C# programming, Boolean data type is used to store and process true or false data type variable value. Where you can represent true and false values in C# programming.
Boolean examples in C# programming.
Size – 1 bit
Possible values - true or false
Default value – false
Boolean data type examples.
bool is_value = true;
bool is_result = false;
Other types of data types in C# programming.
Data Type | Data Type Description | Data Type Variable Example |
string | Sequence of characters | “Hi, Vcanhelpsu” |
object | Base type for all types | object value = 783; |