if, else, else if Javascript
In JavaScript programming, if, else, and else if are program control flow statements that are used to execute different blocks of program code based on single or multiple conditions. These control statements help programmers to make decisions in their program code. JavaScript programmers can apply single or multiple-choice control flow statements according to their program development needs.
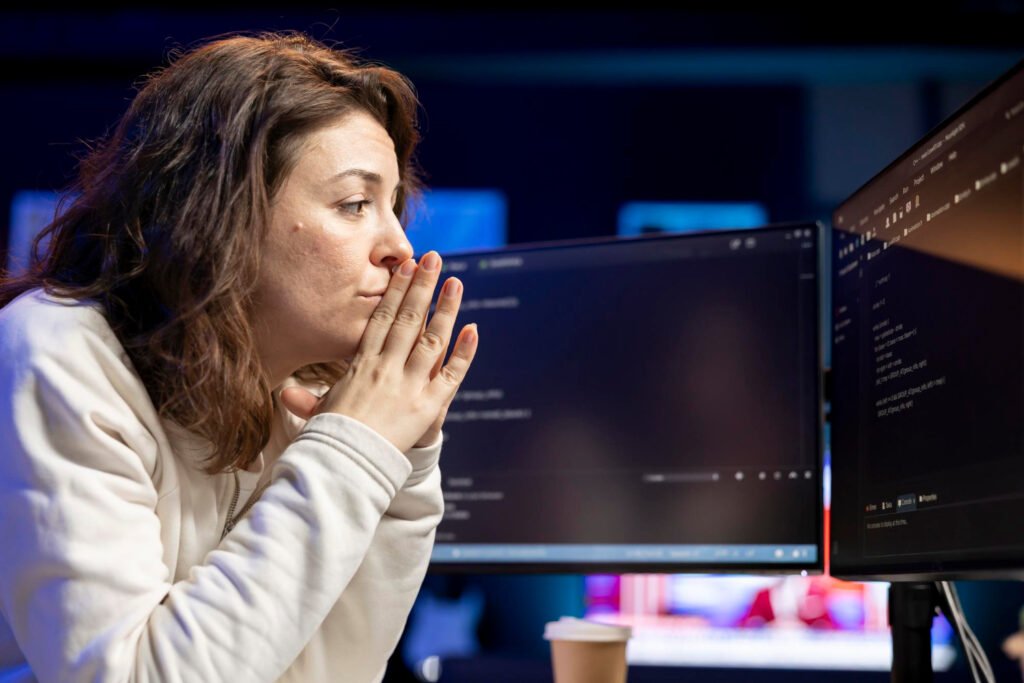
So let’s understand if, else, and else if program control flow statements in JavaScript programming.
If Statement JavaScript Statement.
In JavaScript programming, if the given if program statement condition is true, then if statement is used to execute a block of program code in the current program.
JavaScript if statement syntax.
if (condition) {
// if statement Code to be executed when if the condition is true
}
If statement JavaScript example.
let age = 21;
if (age >= 21) {
console.log(“He is a mature”);
}
// result is – “He is a mature”
Here, if the given condition age >= 21 is true, then the message “He is a mature” is printed.
else Statement in JavaScript.
If the if condition is false in the current JavaScript program, then the else statement is used to execute the block of program code. Here the else block is an alternative choice for the programmer, but programming development can apply it in the if statement block as per the requirement.
Syntax of else statement.
if (condition) {
// if program Code to be executed when if the condition is true
} else {
// else program Code to be executed when if the condition is false
}
Example of else statement.
let age = 14;
if (age >= 21) {
console.log(“He is a mature”);
} else {
console.log(“He is a minor”);
}
// result is – He is a minor
Since the age in the current JavaScript program is not greater than or equal to 21, so if the condition is false, then, here the else block executes the code, which prints the message “minor”.
else if Statement in JavaScript.
The else if statement in JavaScript programs is used to apply multiple new else if conditions in a single program, else if the initial condition is false or not. In the else if statement, you can use multiple else if statements to test multiple branch conditions in sequence or order. If the condition evolution in any one of the program statements here is true, then the corresponding else if block of code in the program will be executed.
else if statement syntax.
if (condition1) {
// if program Code to be executed when if condition1 is true
} else if (condition2) {
// else if program Code to be executed when if condition2 is true
} else {
// else program Code to be executed when if none of the above conditions are true
}
else if statement JavaScript example.
let percent = 94;
if (percent >= 90 && percent<=100) {
console.log(“Grade – A”);
} else if (percent >= 80) {
console.log(“Grade – B”);
} else if (percent >= 70) {
console.log(“Grade – C”);
} else {
console.log(“Grade – F”);
}
// result is – “Grade – A”
Here the first if condition is false (percent >= 94), so, it tests the next else if statement condition. Since the percentage is greater than or equal to 94, it prints the message “Grade – A”.
Example with multiple conditions in else if.
You can also use multiple else if control statements to evolve more than two or three program conditions in a JavaScript program.
Example of else if statement in JavaScript.
let temperature = 48;
if (temperature > 38) {
console.log(“It is hot day outside!”);
} else if (temperature > 27) {
console.log(“The weather cool.”);
} else if (temperature > 19) {
console.log(“wheter is firmly nice.”);
} else {
console.log(“too much cold weather outside!”);
}
// result is – It is hot day outside!
Here in this example above.
- Here the first if condition temperature > 38 is true, so it prints the message “It’s hot outside today!” Here if the given program condition was false, then this else if checks multiple conditions in the program manually one by one.
- if, else if, and else block Combination of multiple conditions.
- You can also combine multiple if, else if, and else if conditions using logical operators (such as && for AND, || for OR) in if, else if, and else block.
if, else if, and else block example.
let age = 21;
let isemployee = true;
if (age < 21) {
console.log(“You are a minor employee”);
} else if (age >= 18 && isemployee) {
console.log(“You are an mature employee”);
} else {
console.log(“you are not a mature employee”);
}
// result is – You are an mature employee
Here in this example above.
- Here if the condition age >= 18 && isemployee prints the message “You are an adult employee” if the condition is true.
Nested if Statement in JavaScript.
You can also test multiple program conditions by nesting if, else if, and else program statements within each other as per your program development requirement.
Nested if Statement JavaScript Example.
let age = 30;
let salary = 40000;
if (age >= 21) {
if (salary >= 27000) {
console.log(“yor are Eligible for services”);
} else {
console.log(“your salary too low for services”);
}
} else {
console.log(“Not eligible for services”);
}
Here in this example above.
- First, it tests in the if statement whether the person is an adult (age >= 21).
- If the person is an adult, then it checks whether the income is above a certain limit (salary >= 27000) or not.
- Here if both the conditions are true, then it prints the message “Not Eligible for Services”.
Here the key points for you to remember.
- if – Here it tests the if condition, and if the if condition is true, then the program executes the code block.
- else – Here if the previous condition is false, then the program executes the code block.
- else if – Here if the previous condition is false, then it is used to test multiple conditions.
- condition – The conditions inside if and else if in a JavaScript program are usually conditional expressions that evolve to a Boolean operator (true or false) value.
if else if statement example summary.
let activetime = 13;
if (activetime < 10) {
console.log(“its a morning day!”);
} else if (activetime < 15) {
console.log(“its a Good afternoon time!”);
} else {
console.log(“it a Good evening time!”);
}
// result is – its a Good afternoon time!
Here in this example.
- The first condition here tests if the active time is less than 10 (which it is), so it moves on to the else if control flow statement.
- The second condition here tests if the time is less than 15 (which it is), so it prints the message “It’s a good afternoon!”.
Conclusion of if, else, and else if statements in JavaScript.
The if, else, and else if control statements in JavaScript programs are essential control flow options choices in a program that allow the JavaScript programmer to make decisions based on the given logic code. Using these statements, you can execute different blocks of program code where the program code depends on whether particular conditions are met or not. Here the else if program statement allows the programmer to test multiple program conditions, and here the else block ensures that if none of the conditions are true, then the else statement prints the message.