Fetching data from APIs using fetch()
The fetch() function in the JavaScript programming language is an advanced JavaScript process or method for processing an online network request in a program. Such as collecting data information from an API from a network. Where fetch() is built into the web browser, where the fetch function is a built-in feature of the API. Where fetch() gives you a more powerful, flexible web browser client network request function, unlike the old XMLHttpRequest. Where fetch() returns a promise, making it easier to manage and control asynchronous program operations in JavaScript programs.
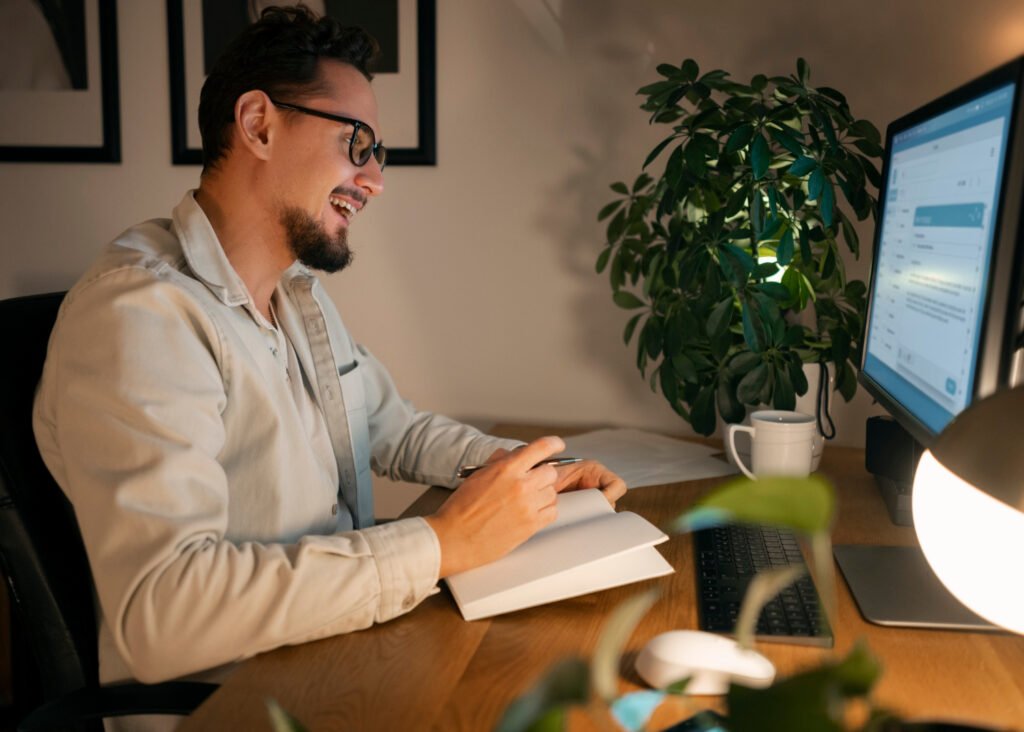
Basic syntax of fetch() in JavaScript.
The basic syntax of fetch() is as follows.
fetch(url, options)
.then(response => response.json()) // it Parse the JSON from the response location
.then(data => console.log(data)) // it use to Handle the data
.catch(error => console.error(‘Error:’, error)); // it use to Catch and log any request errors
- url – This is the URL resource location in the fetch function from which you want to receive data in the client request.
- options – Here options is a configuration object to customize an optional web request, such as, method, header, body, etc.
By default, the GET method is used to receive data from the fetch() function in a JavaScript program. However, JavaScript programmers can manually customize it to send data via POST, PUT, or other HTTP methods based on their web development needs.
Getting data from public API in JavaScript.
Here is a basic example of receiving data from a public API like JSONPlaceholder API in a JavaScript program. It provides programmers with sample JSON data for web request testing.
Example of getting user data from JSONPlaceholder API in a JavaScript program.
fetch(‘https://jsonplaceholder.typicode.com/users’)
.then(response => {
// here it Check if the response is successful
if (!response.ok) {
throw new Error(‘current Network response is not ok’);
}
return response.json(); // it use to Parse the JSON data from the response
})
.then(data => {
console.log(data); // it use to Handle the fetched data
})
.catch(error => {
console.error(‘it produce a problem with the fetch operation -‘, error);
});
Here in this example
- Here in this example we receive a user list from https://jsonplaceholder.typicode.com/users endpoint.
- Here we test the operation to see if the operation was successful using the response response.ok. If it is not, we throw an error.
- Programmers then parse the JSON data as needed using the response.json() function, which returns a promise.
- If all these operations are done properly, the programmer logs the data to the console.
- If any error is generated in this operation, such as network problems, invalid JSON, etc., it is caught in the catch() block.
Using fetch() with POST requests in JavaScript.
To send client data requests to an API in JavaScript, such as creating a new resource, JavaScript programmers can apply the POST method by passing an options object indicating the method, header, and body in the web request.
Example of sending data with a POST request.
const newUser = {
name: ‘David’,
email: ‘david@yahoo.com’,
username: ‘david’
};
fetch(‘https://jsonplaceholder.typicode.com/users’, {
method: ‘POST’, // it use t Specify the HTTP method
headers: {
‘Content-Type’: ‘application/json’ // it use to Specify that we are sending JSON
},
body: JSON.stringify(newUser) // it use to Convert the JavaScript object into JSON
})
.then(response => response.json()) // it use to Parse the JSON response
.then(data => {
console.log(‘New user record construct -‘, data); // it use to Log the response from the server
})
.catch(error => {
console.error(‘Error:’, error); // it use to handle any post method errors
});
Here in this example.
- Here we define an object newUser, which the programmer wants to send to the API.
- Here we indicate the POST method, and set the Content-Type header by moving it to application/json. Because here we are sending JSON data.
- Where the main portion of the web request is the JSON string version of the newUser object.
- Here the response is parsed as JSON, and we log the response to the console.
Handling response status codes in JavaScript.
In JavaScript programs, it is important to properly manage the HTTP response status codes in web requests to know that your application is behaving properly. For example, the API may return 404 (Not Found) or 500 (Internal Server Error), so you need to test any data before using it.
Handling HTTP status codes in JavaScript example.
fetch(‘https://jsonplaceholder.typicode.com/users’)
.then(response => {
if (!response.ok) {
throw new Error(`HTTP request error produce, check the Status: ${response.status}`);
}
return response.json(); // it Parse JSON where the response is successful
})
.then(data => {
console.log(data); // it use to Handle the data
})
.catch(error => {
console.error(‘it produce a problem with the fetch request data operation -‘, error); // it use to Handle any kind of request errors
});
Here in this example.
- Here in this program, we test whether the web https response.ok is true, which indicates status code 200-299.
- If the response is not successful, we throw an error with the status code for program debugging purpose.
- If the response is successful, we use the .json() function method to parse the response.
Async/Await syntax for fetch request in JavaScript.
Where the fetch() function in a JavaScript program returns a promise, JavaScript programmers can use async/await to write more readable program asynchronous code. Where async/await allows programmers to work with promises just like synchronous code.
Fetching data with async/await in JavaScript example.
async function getemployeeData() {
try {
const response = await fetch(‘https://jsonplaceholder.typicode.com/users’);
if (!response.ok) {
throw new Error(‘here Network response is not proper’);
}
const data = await response.json(); // it use to Parse the JSON response
console.log(data); // it use to Handle the data
} catch (error) {
console.error(‘it produce a problem with the fetch data operation -‘, error);
}
}
getemployeeData();
Here in this example.
- Here in this program, the getemployeeData() function is declared as async, which means it can use await inside.
- Here await is used with the call to the fetch() function, which halts the function until the promise operation is resolved.
- The .json() method is also awaited to ensure that we have the parsed JSON data before proceeding.
- This overview makes the program code more readable than .then() and .catch() chaining, especially when you have many asynchronous calls.
Handling errors in fetch() in JavaScript.
Here in fetch() operation, network errors like no connection or invalid responses like 404 are not automatically thrown as errors. So here programmer has to manually test the response status.
Example of handling errors.
fetch(‘https://jsonplaceholder.typicode.com/users’)
.then(response => {
if (!response.ok) {
throw new Error(‘it Failed to handle fetch data. and Status is – ‘ + response.status);
}
return response.json();
})
.then(data => {
console.log(data);
})
.catch(error => {
console.error(‘it display Error-‘, error);
});
Here in this example above.
- If the response status is not displayed in 200-299 range order, then we throw an error.
- Here catch() method catches any error that occurs during fetch process including network problems or invalid JSON parsing.
Sending headers with fetch() in JavaScript.
If you need to add additional headers authentication, content-type, etc., then you can indicate them in header option as per requirement.
Sending custom headers in JavaScript example.
fetch(‘https://jsonplaceholder.typicode.com/users’, {
method: ‘GET’,
headers: {
‘Authorization’: ‘Bearer myAccessToken’, // it use to Send an authorization token
‘Accept’: ‘application/json’ // it use to Tell the server we expect JSON in response order
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(‘display Error:’, error)); // it use to handle error
Here in this example, custom headers are present in the request. Headers are often used for request authentication, content communication, and other purposes.
Handling JSON and other data formats in JavaScript.
While the response.json() method is typically used for APIs that return JSON, you may need to manage and control other types of responses, such as text or binary data formats.
Handling text response in JavaScript example.
fetch(‘https://example.com/some-text’)
.then(response => response.text()) // it use to Parse the response as plain text file format
.then(data => console.log(data)) // it use to Handle the text file data
.catch(error => console.error(‘it display Error -‘, error));
Here in this example, the response.text() method is used to parse responses as simple text.
Summary of getting data using fetch() method function in JavaScript.
- API Basic Syntax – Where JavaScript programmers use fetch(url).then(response => response.json()) method to receive JSON data from an API.
- HTTP Methods – Here use these methods to send POST, PUT, DELETE, etc. in web request.
- Error Handling – Always test response.ok to manage HTTP errors in web request and catch network problems using .catch() function.
- Async/Await – Use async/await for more readable asynchronous program code in JavaScript program.
- Custom Headers – Pass headers like authentication in header options.
- Data Format – Use method functions like response.json(), response.text(), response.blob(), etc., depending on required response format.