Defining functions in python
In Python programming, functions are created for a specific programming purpose. After defining a function in a Python program, you can group these functions into reusable blocks of program code. Functions convert large Python program code blocks into small program modules. Finally, all these function modules are called one by one in the program.
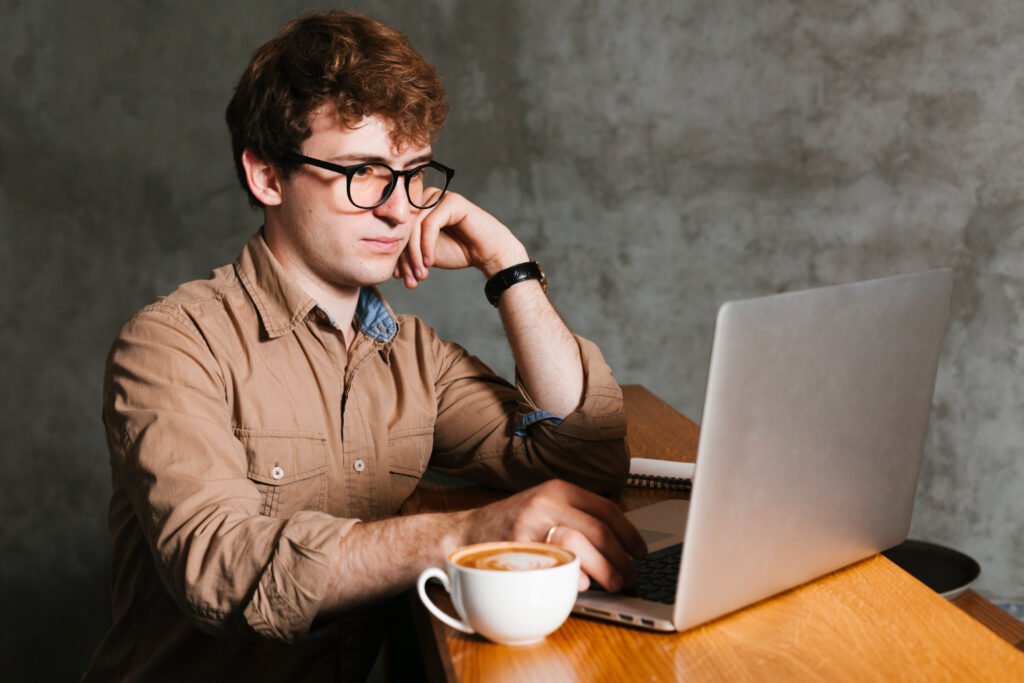
So let’s understand functions in Python programming in a better way.
Python function basic syntax.
def create_funcname(parameterst):
“”docstring”””
# basic function body
function return expression
def keyword – def keyword is used to define or start a user defined function definition.
create_funcname – Create function name is the name of the function to be created by the user.
Function parameters – Function parameters are the function arguments or parameter objects declared in the function, and are the input values (optional) accepted by the function.
function docstring – This is an optional document string element to explain the purpose of the function in the current function.
Function return type – If any function has a return value and you want to return the value returned by the function, then create a function return type.
Python function example.
def welcome(info):
“”display the name of website.”””
return f”welcome to the – {info}”
# it calling the above welcome function and print values
print(welcome(“vcanhelpsu.com”)) # the result is – welcome to the – vcanhelpsu.com
Function parameters.
# python Functions declare with p,q parameters/argument
def total_integer(p, q):
#display the total of p,q parameter
return p + q
print(total_integer (1, 2)) # the result is – 3
Python function return statement.
Python function programs can alternatively return user defined function values using function return statement. If the function you have created does not have any return values then by default this function returns non function value.
def sqrt(p):
# the sqrt function display the square root of any number
return p ** 4
output = sqrt(4)
print(output) # the result is – 256
Function default parameters.
You can set default values for function parameters/arguments as per your requirement in any Python function program.
def welcome(name, age=25, cont=9414):
# welcome function declare with three parameter argument
return f”{name}, {age}, {cont}”
print(welcome(“name is – jackie”)) # the result is – name is – jackie, 25, 9414
print(welcome(“contact is – 9414”)) # the result is – contact is – 9414, 25, 9414
print(welcome(“rock”, “welcome”)) # the result is – rock, welcome, 9414
Python function docstrings.
Use of docstrings (documentation strings) in Python function declare function purpose indicates what role the function you are using in the program. Where triple quotes (“”” “””) are used to write multi-line docstrings comments.
def substraction(p =4, q=2):
“”display the substraction of two integers.
Argument:
p (use integer or float) function p argument.
q (declare integer or float) function q argument.
Returns:
integer or float – substraction of p and q.
“”
return p – q
print(substraction)
Python Scope of Variables.
The variables defined inside a Python program function are by default of local scope nature for that function. And these local functions are automatically removed after Python program execution.
def local_function():
p = 9 # function Local parameter variable
print(f”this is a local function – {p}”)
local_function() # the result is – this is a local function – 9
#print(p) # if you tray print value p it display error because p variable define as local/inside function not outside/global function
Python Global Variables.
Use function variables declared outside any Python program function are of global nature, and can be accessed from any function in the current function program. If you want to declare global function in your Python program. Then global keyword is used to declare global variable inside the function.
p = 2 # declare Global variable outside function
def alter_globalvar(): # declare alter global varible function
global p
p = 7 # modify local variable value
print(p) # the result is – 2
alter_globalvar()
print(p) # the result is – 7
Python Lambda Function (Anonymous Function).
In any Python program, lambda functions are small anonymous function types. Which Python programmers declare with the reserved lambda keyword. After declaring the lambda function, the programmer can define the arguments as per his requirement, but a lambda function can have only one program expression.
Python Lambda Function example.
multiply = lambda p: p ** 3
print(multiply(9)) # the result is – 729