Method Syntax
In C# programming language, method syntax has similar features as other object-oriented programming languages, but C# programming has some basic rules and regulations to define class methods.
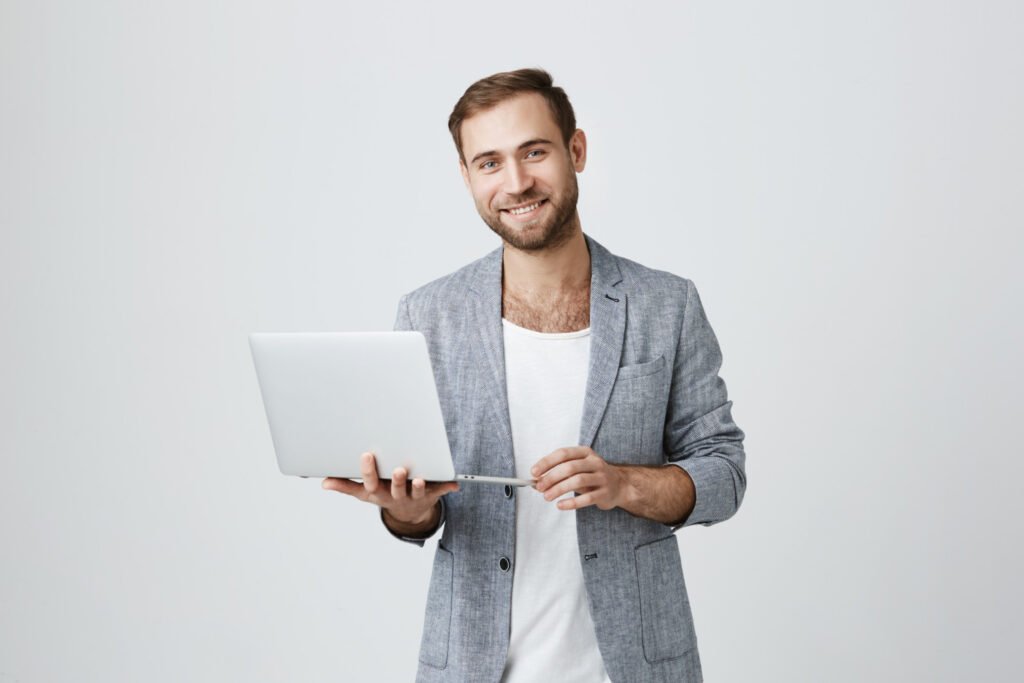
So let’s get to know more about method syntax features in C# programming.
Defining Method in C# Programming.
Method syntax in C# programming language can be defined with the following structure.
returnType MethodName(parameter1Type parameter1Name, parameter2Type parameter2Name)
{
// C# program Method body
return value; // it set return value for program
}
Example of method in class in C# program.
using System;
class TestClass
{
// Delare Method with a return data type
public int mulinteger(int p, int q)
{
return p * q;
}
// Declare Method without a return type (void method)
public void PrintMessage(string textmessage)
{
Console.WriteLine(textmessage);
}
}
class Program
{
static void Main(string[] args)
{
TestClass testClass = new TestClass();
// It Calling the multiply integer method
int multiply = testClass.mulinteger(4, 3);
Console.WriteLine(“\n the multiply is – ” + multiply); // the result is – 12
// It Calling the Print textMessage method
testClass.PrintMessage(“\n Hi, Vcanhelpsu Welcome To C#!”); // the result is – Hi, Vcanhelpsu Welcome To C#!
}
}
Method return types in C# program.
Class method in C# programming language can have multiple return types if the program has a return data type, or the program has no return data type.
- Void Return Data Type – If there is no return data type in C# program language, then this method does not return any value i.e. void data type value.
- Other C# Program Return Data Types – In this you can return any data type value from data types like int, string, double, etc. in C# program at the end of the program.
Example of a method returning value in C# program.
public int Mode(int p, int q)
{
return p %q;
}
Calling a method in C# program.
To call a method in C# program, you use the instance of the defined class (if the class method is not static) or the class name (if the class method is static) method.
Calling an instance method in C# program.
TestClass testClass = new TestClass();
testClass.PrintMessage(“This is the instance class method example”);
Calling static class method in C# program (without instance).
class SquareRoot
{
public static int Square(int p)
{
return p * p;
}
}
class Program
{
static void Main(string[] args)
{
int output = SquareRoot.Square(7);
Console.WriteLine(output); // The result is – 49
}
}
Method overloading in C# program.
C# programming supports method overloading, where you can have multiple different methods in a class program with the same name but different class parameter argument variables.
class Calculate
{
public int total(int p, int q)
{
return p + q;
}
public double total(double p, double q)
{
return p + q;
}
public string total(string p, string q)
{
return p + q;
}
}
C# Programming Method Modifiers.
You can use method modifiers to indicate the access level of a C# programming method.
For example,
- public – Public C# programming methods can be accessed or called from anywhere in the current program.
- private – Private C# programming methods can be accessed only from within the class or through defined class members.
- protected – Protected C# programming methods can be accessed only from within the class and derived classes.
- static – C# programming methods are related to the class itself, not the instance.
public void PrintMessage() // public method Can be accessed anywhere in program
{
Console.WriteLine(“This is a public class method”);
}
private void SecureMethod() // private class method Can only be accessed within this class
{
Console.WriteLine(” This is a private class method”);
}