FileReader, BufferedReader
In Java programming, FileReader and BufferedReader class functions are used in Java program file handling file operation to read data and information from created internal storage files character by character or a complete row of information at a time. But here FileReader and BufferedReader have different programming purposes and special features as per the Java developer requirement.
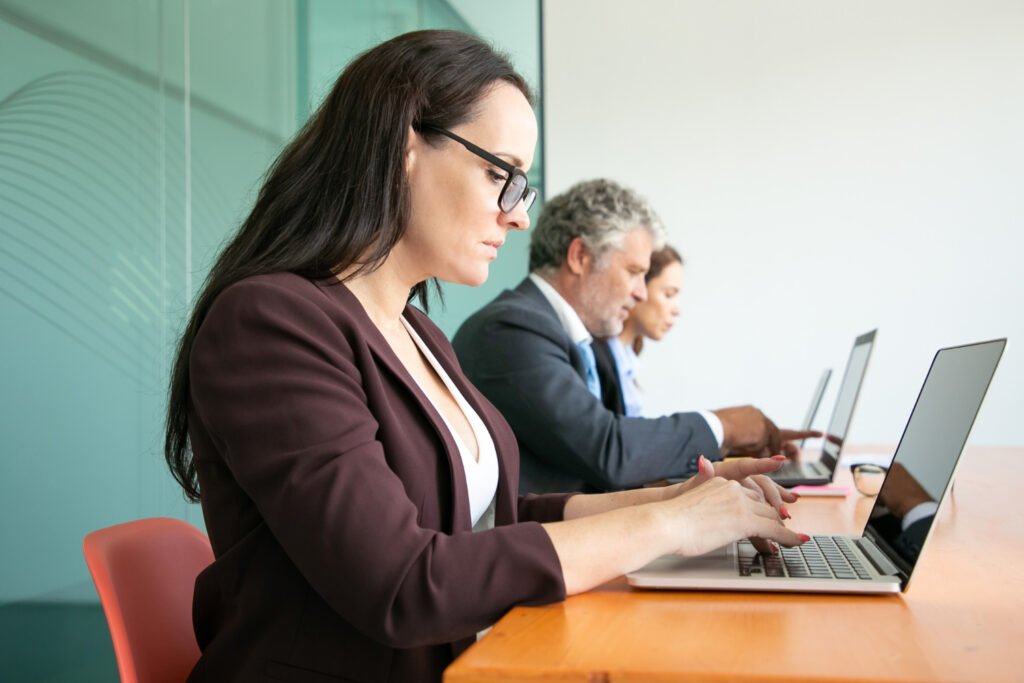
So, let’s understand both FileReader and BufferedReader classes and their usage in Java programming.
FileReader Class in Java Programming.
FileReader is a subclass of InputStreamReader in Java file handling, and it is used to read data and information from character files in Java program. File Reader is designed to read files in default character encoding which may vary depending on multiple platforms. But File Reader is usually used in UTF-8 or other file formats depending on the current system-hardware. Where the FileReader class reads one character at a time from a file in a Java program.
Key Features of FileReader Class in Java.
The File Reader class is used to read data and information from files as a stream of characters in the current Java program file.
In file handling, the File Reader class is designed to read data and information from files character by character. Where the File Reader class works best for text files.
If the file does not exist in the current program, it throws FileNotFoundException and IOException for input-output errors.
Basic usage of FileReader class in Java.
import java.io.FileReader;
import java.io.IOException;
public class FileReaderExample {
public static void main(String[] args) {
FileReader fileReader = null;
try {
fileReader = new FileReader(“test.txt”);
int character;
while ((character = fileReader.read()) != -1) {
System.out.print((char) character); // filereader class use convert int to char and print their value on screen
}
} catch (IOException e) {
System.out.println(“\n display Error when reading file – ” + e.getMessage());
} finally {
try {
if (fileReader != null) {
fileReader.close(); // it close the test.txt file after reading it
}
} catch (IOException e) {
System.out.println(“\n display Error closing file – ” + e.getMessage());
}
}
}
}
Java FileReader Class Explanation.
The FileReader class is used in the current program to open the “test.txt” file and read data and character information from the file.
Where the read() method in the current program reads only one character at a time. It returns the character as an integer (Unicode value) in the program, so we cast it to char data type to print it.
The while loop continues till the end of the current file, and read() returns the value -1.
After reading the file, we close the FileReader class to release the file resources.
BufferedReader Class in Java.
BufferedReader in Java file handling programs is a more efficient process of reading text data information from a file (or any other character-based input stream) because it reads large portions of data into the internal buffer at a time, reducing the number of file read operations. BufferedReader class in Java programs can be used to read text line by line instead of character by character, which enables Java programmers to be much more efficient when working with long large text files.
Key Features of Java BufferedReader Class.
Buffered Reader wraps around all sides of a Reader such as FileReader in a Java program, and adds buffering features to an existing file system, thereby improving file text information reading performance.
Buffered Reader provides Java programmers with the readLine() method, which reads an entire row of a file at a time.
The Buffered Reader class is ideal for reading large files or when you need to process text character by character.
Basic usage of BufferedReader class.
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class BufferedReaderExample {
public static void main(String[] args) {
BufferedReader bufferedReader = null;
try {
bufferedReader = new BufferedReader(new FileReader(“test.txt”));
String line;
while ((line = bufferedReader.readLine()) != null) {
System.out.println(line); // buffer reader lass function Print each line of the test file one row in single time
}
} catch (IOException e) {
System.out.println(“\n it display Error when reading file – ” + e.getMessage());
} finally {
try {
if (bufferedReader != null) {
bufferedReader.close(); // it Close the BufferedReader class after reading file all row
}
} catch (IOException e) {
System.out.println(“\n it display Error when closing file – ” + e.getMessage());
}
}
}
}
BufferedReader class explanation.
Here in a Java file handling program, BufferedReader is initialized with FileReader to read data and information from “test.txt”.
Whereas in this program, the readLine() method reads data and information from a row of text at a time, and returns the text line as a string.
In this program, the loop continues until the readLine() method returns zero, which indicates the end termination of the file.
After reading the file, the BufferedReader is closed to release the file resources.
Main Differences Between FileReader and BufferedReader class
Feature | FileReader | BufferedReader |
Purpose | Filereader class use to Reads a file character by character. | Buffer reader class use Buffers input for more efficient reading, and reads lines of text. |
Efficiency | Filereader class Not as efficient for large files because, it reads one character at a time in active file. | Bufferreader More efficient, as it reads larger chunks into memory and reduces the number of I/O file operations. |
Read Method | In filereader class read() – reads one character at a time in active file. | In bufferreader class readLine() – use to reads an entire line of text in active file. |
Use Case | Here filereader class Simple text reading tasks, for example, reading small files or individual characters in any file. | Buffrer reader class use to Reading larger files or text files line-by-line in active file. |
Common Use | FileReader is commonly used in combination with BufferedReader for file performance improvements. | Bufferreader class Best used when processing text files, especially when reading lines. |
Constructor | Where FileReader constructor accepts a file name or File object. | Where BufferedReader needs a Reader as input, commonly FileReader. |
FileReader and BufferedReader Classes in Java File Handling.
- For file efficiency – BufferedReader class is recommended when reading text files in Java file handling because it buffers the input and reduces file reading numbers in I/O operations.
- When you need to read the file line-by-line – BufferedReader class is a better class function for reading text line-by-line in Java file. While FileReader is limited to reading character-by-character text information only in Java file operations.
- When working with small files – If you are working with small files in Java file operations then only FileReader class function may be sufficient for you. But in most cases BufferedReader Java file class is preferred for better file handling performance.