Single Dimensional Arrays
In Java programming, a one-dimensional array is a continuous data storage array structure block that stores and processes data type array elements of the same type. These are stored in a continuous sequence in a linear order in a computer secondary storage location. Declaring an array in a Java program is an indexed list of data element values that are accessed and processed using their storage index locations to process the array.
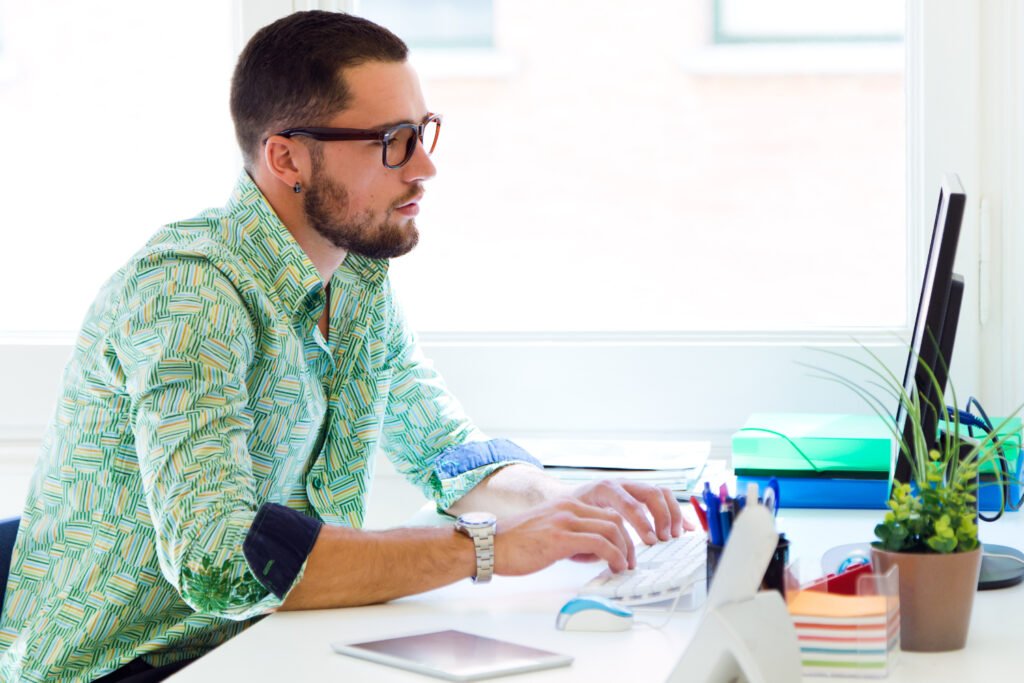
Syntax for declaring a single-dimensional array in Java programming.
Example of a basic array declaration.
data type[] arrayName;
Here datatype can be any valid Java data type data declaration type. For example, you can manually declare a single dimensional array with data types such as int, float, char, etc.
Here arrayName is the user generated array name that you assign to the array in the current program
Java Array Initialization – In Java programming, array can be initialized or started in two ways.
One-dimensional array declaration with values.
int[] values = {9, 21, 8, 0, 53};
One-dimensional array without values (specify custom size).
int[] values = new int[7]; // Here one dimensional size 7 array is created.
Example of single-dimensional array program in Java.
public class Main {
public static void main(String[] args) {
// here we Declaring and initializing an values name array
int[] values = {70, 90, 10, 34, 52};
// let Access elements of the values array with their index loation
System.out.println(“\n First index array element – ” + values[0]); // result is -70
System.out.println(“\n Second index array element – ” + values[1]); // result is -90
System.out.println(“\n 4th index array element – ” + values[4]); // result is – 52
// let modify the element in the values array
values[3] = 300; // let modify the 4th element of array(index 3)
// let Print and proess all values array elements with for loop
for (int p = 0; p < values.length; p++) {
System.out.println(“\n the array Element at index – ” + p + “: ” + values[p]);
}
}
}
Java Array Key Points.
- Array Indexing – Always in Java array program, array storage element starts from index 0 location. And continues to store the next number of array elements. Where, first array element is stored and processed at array index array[0] location, second array element is stored and processed at array[1], and so on in Java program.
- Length of an array – In a Java program, you can manage and control the length of the desired array by using array.length as per your programming requirement.
- Size of array – Remember that once an array is created in a Java program, its storage size is fixed. Here, if you need other array sizes in the same program, then you have to create a new array data type as per your requirement.
Java Array Operations.
- Accessing array elements – In a Java program, array elements are accessed and processed by using one-dimensional array index. For example, integer[0]) is the array 1st index location.
- Modifying array elements – As per the requirement, a Java programmer can assign a new value to a particular array index location. For example, values[3] = 79;), here the array value at the 4th index location in the values array has been modified by 79.
- Looping through an array – You can use for loop, while loop, do while loop, etc. to process or loop a One D array from start to end in a Java program.
Advantages of single-dimensional arrays in Java programming.
- One D Array Easy to use – One D array in Java program is easy to access and process array elements with index storage location.
- One D Array Fixed Size – Declaring array elements in Java program provides a structured, already stored values array data element collection.
Limitations of single-dimensional arrays in Java.
- One D Array Fixed size – Always remember that once the size of array is declared in One D array in Java program, you can never modify it.
- One D Array Flexibility – If you want to store more dynamic or multi-dimensional array elements. So you have to use other 2-D, and 3-D data types like arrays.