Enumerations in c
In C programming, enumeration data type (enums) is a user defined data types variable combination element collection like structure. Enumerations contain special constant elements. Enumerations in C program provide different data types values according to user provided values. This makes the enumeration based data type source code more readable and maintainable.
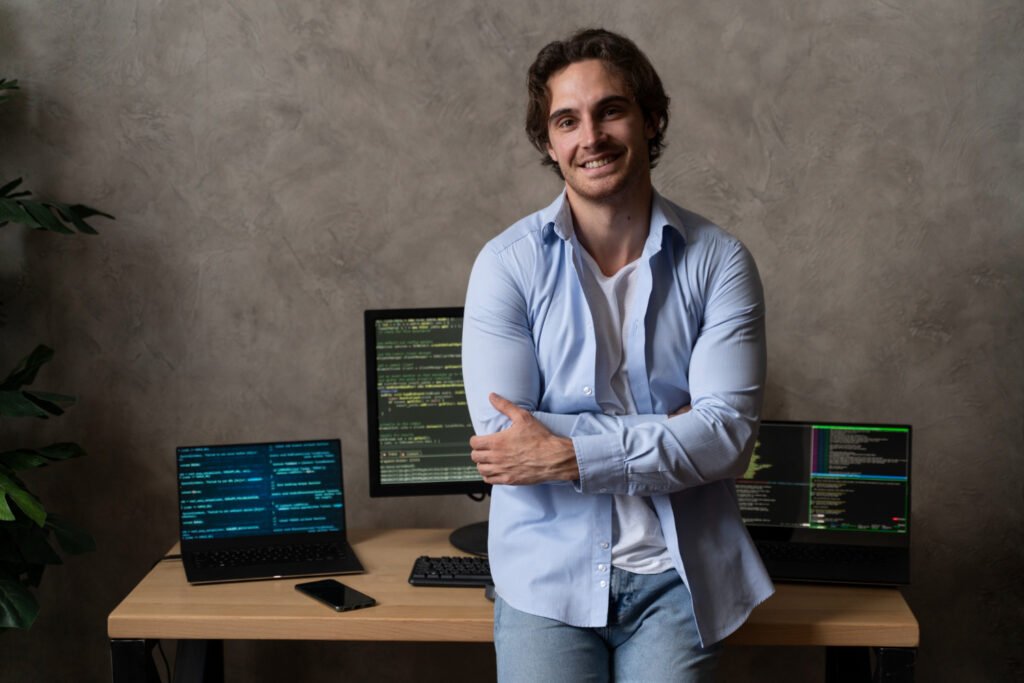
Defining Enumerations in C Programs.
An enumeration is declared using the enum keyword in any C program, followed by the name of the enum and the enumeration data type is a collection of integer constants.
Basic example of enumeration in C Program.
#include <stdio.h>
// HERE YOU Define an COURSE enumeration for COURSE SELECTION
enum Course {
C,
JAVA,
PYTHON,
HTML,
CSS,
Sql,
JAVASCRIPT
};
int main() {
// Declare a variable FOR your selection
enum Course ch = PYTHON;
// here you Print the selected enumeration course element
printf(“\n Today yo select this %d programming”, ch);
return 0;
}
In the above course enumeration program.
Here C language course is assigned the value 0.
Java programming course is assigned the value 1.
Python programming is assigned the value 2, and so on. Each programming course is assigned a different value.
Assigning Specific Values to C Enumeration.
You can provide unique integer values to constants in an enumeration program. Remember that if you do not explicitly provide the first value to the enumeration, it will default to 0, and every subsequent enumeration value will be incremented by 1.
#include <stdio.h>
// create test value enumeration for value test
enum TestValue {
VALUE = 0,
VALUE_TRUE = 1,
VALUE_FALSE = 0,
UNKNOWN_VALUE = -1
};
int main() {
// let here Declare a variable for test value
enum TestValue value = VALUE_TRUE;
// let Print the value of the test value enumeration
printf(“\n The Test Value Is – %d”, value);
return 0;
}
Using Enumeration in C Programs.
In C programs enumeration can be used for switch statements, comparisons and any place in programming source code where you can use integers.
C Program Switch Statement Enumeration Example.
#include <stdio.h>
// HERE YOU Define an healthstatusenumeration for health check
enum Healthstatus {
WELL,
UNWELL,
OK
};
void printHealthstatus(enum Healthstatus status) {
switch (status) {
case WELL:
printf(“\n your helth is well”);
break;
case UNWELL:
printf(“\n your health is unwell”);
break;
case OK:
printf(“\n your health is ok”);
break;
default:
printf(“\n yo select invalid option”);
break;
}
}
int main() {
enum Healthstatus currentstatus = WELL;
printHealthstatus(currentstatus);
return 0;
}
Enumeration and Typedef Data Type in C Program.
You can create aliases for enumeration data types using typedef data types to make program code more detailed in C programming.
Example of Typedef Data Type in C Language.
#include <stdio.h>
// let Define an enumeration for fruit variable
typedef enum {
MANGO = 1,
ORANGE,
PAPAYA,
CHIKU,
APPLE,
PEACH,
BANANA,
APRICOT,
PINEAPPLE,
WATERMELON,
MUSKMELON,
POMEGRANATE
} Fruit;
int main() {
// let Declare a variable of type Fruit
Fruit selectFruit = APPLE;
// let Print the value of the fruit enumeration
printf(“\n you select the fruit number is – %d”, selectFruit);
return 0;
}
Usage in C Enumeration Bit Fields.
C Program Enum with Bit Fields Example.
#include <stdio.h>
// Define an enumeration for file yes no invalid status
typedef enum {
YES = 1 << 0, // 1 bit
NO = 1 << 1, // 2 bit
INVALID = 1 << 2 // 4 bit
} EXECUTION;
// let Define a structure with bit fields
struct FileExecution {
unsigned int yes : 1;
unsigned int no : 1;
unsigned int invalid : 1;
};
int main() {
struct FileExecution file = {1, 0, 1}; // set Read and file Execute status
// let Print the file execution state
printf(“\n File selection mode is – %d%d%d\n”, file.yes, file.no, file.invalid);
return 0;
}
Enumeration Data Type in C Language.
In C programming language you can define a data type set of integer constants named enumeration, which greatly improves the readability and program maintenance of your enumeration program code.
Key points about Enumeration.
Enumeration Definition – To define enumeration in C program, use the built-in enum keyword.
Enumeration Unique Value – Enumeration constants are given unique values with the enum keyword.
Using Enums – You can use enumeration data type in switch statements, comparisons, operators, etc. in C programs.
Typedef Data Type Usage with Enums – You can create an alias data type for enumeration data type in C program.
Usage of Bit Fields – You can use enums keyword with bit fields to represent flags in C program.