Decision making (if-else, switch-case)
The process of making decisions based on the design program variables in C programming is facilitated and easy through conditional statements like if-else and switch-case. If-else statements help you to apply any programming logic in true or false order. In the same switch-case statement, you can print or select multiple switch-case conditions or statements. These statements let you execute different blocks of program code based on some programming condition logic.
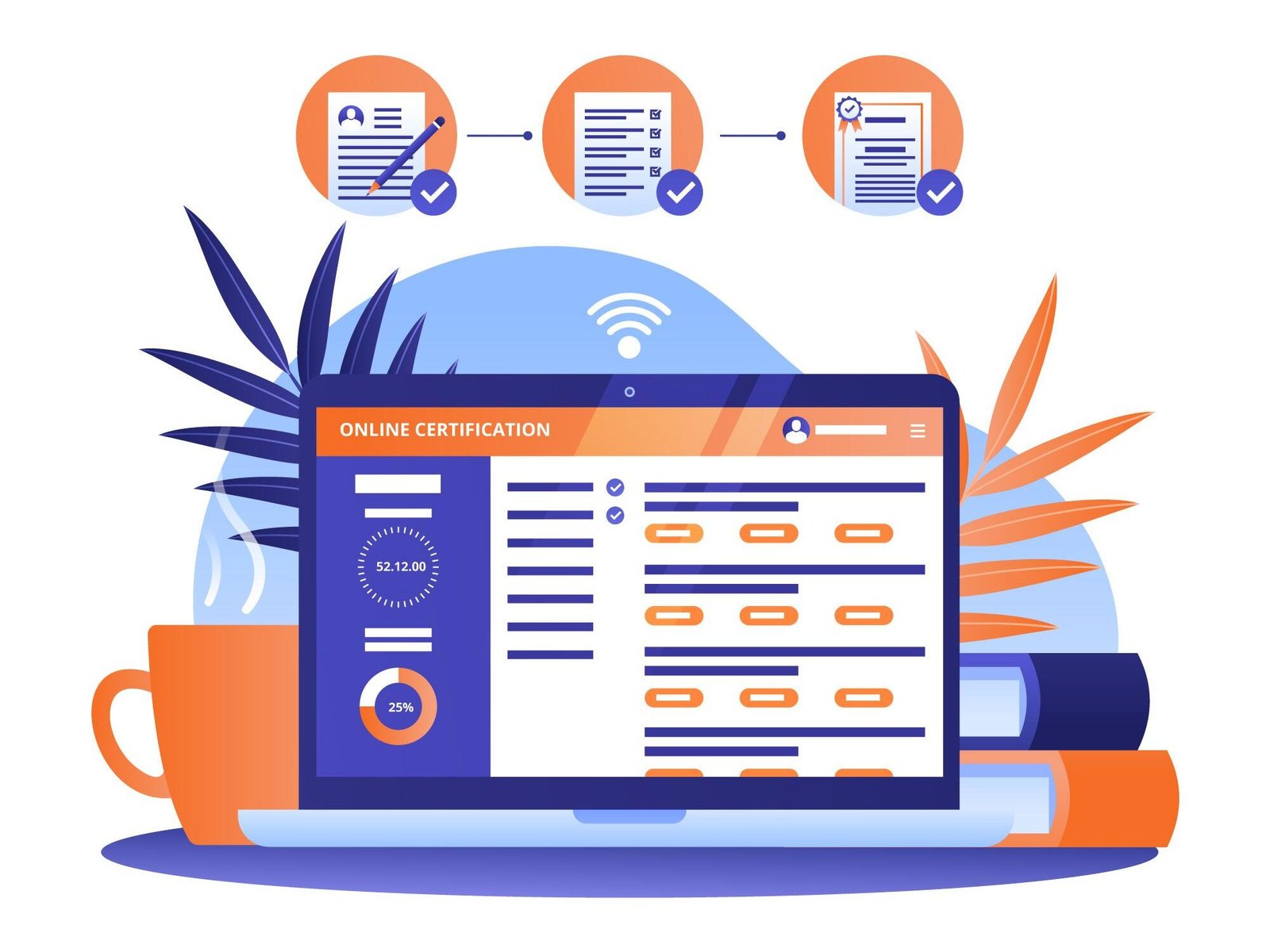
So let’s understand if-else and switch-case statements better in C language.
if-else statement in C language. The if-else logic statement evaluates a program condition, and immediately executes a block of the current program code if the program condition is true. Alternately, the else block information is displayed to execute the code if the program condition is false.
if else logic syntax in C language.
if (condition)
{
// if program Code to be executed if the given condition is true
}
else
{
// Code to be executed when the given if condition is false
}
if else Logic Syntax in C Language.
Basic if else Example in C.
int p = 3;
if (p > 1)
{
printf(“\n p is greater than 1”);
}
else
{
printf(“\n p is not less than 1\n”);
}
Switch-Case Statement in C Programming Language The switch-case statement allows you to select one of multiple blocks of program code to be executed based on multiple program expression values. Each switch-case statement case represents a different user-defined value information. Which can be based on the value given by the programmer in a switch-case expression.
switch-case Statement Syntax in c programming.
switch (expression)
{
case value1:
// the given program Code to be executed if expression found value1
break;
case value2:
// the given program Code to be executed if expression found value2
break;
case value3:
// the given program Code to be executed if expression found value3
break;
// display more case statements according to need…
default:
/ the default expression Code to be executed if expression doesn’t match any above given case
}
switch-case Statement Example in c programming.
char course = ‘c’;
switch (course)
{
case ‘P’:
printf(“\n Python”);
break;
case ‘C’:
printf(“\n C Programming”);
break;
case ‘J’:
printf(“\nJava Programming”);
break;
case ‘H’:
printf(“\n Html Development”);
break;
default:
printf(“\nInvalid Course Selection”);
}
About if-else/switch-case statements.
If-else logic statements are used in C programming when there are multiple program conditions to be evaluated by the C programmer, or when the logic or condition is in a more complex order. Where you can make either one of the if or else conditions true at a time.
Switch-case statement is used in C language when the C language programmer needs to test a single switch-case statement expression value against multiple values. In this, the break statement is used to exit the switch-case block after executing the respective switch-case condition. The default case is optional in switch-case statements and it executes when none of the case expressions given in the switch case is true.