Variables and constants in C
In C programming, variables and constants declared in the program are essential program components used to store different types of data values and manipulate program values. Variables and program constants in C programs serve different programming purposes, and variables and program constants are declared in slightly different ways depending on the proper program syntax.
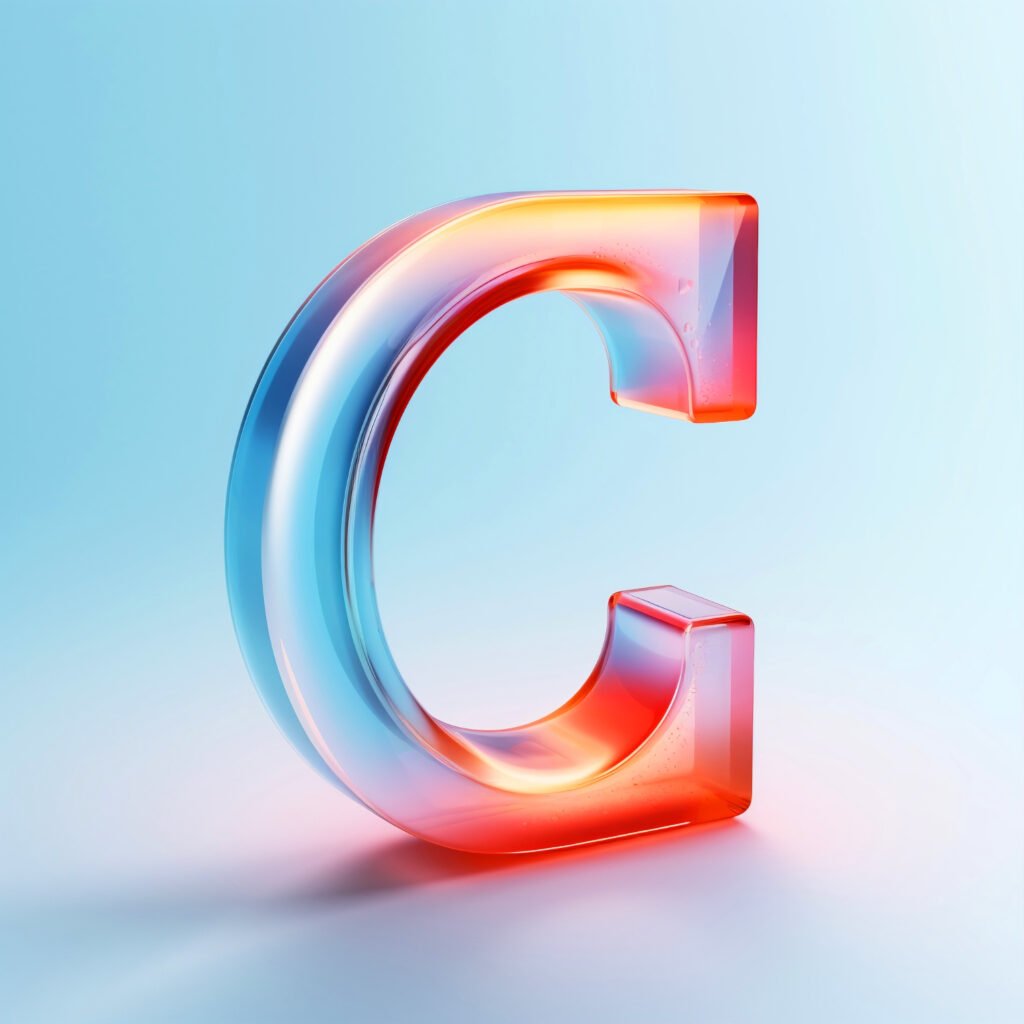
Variables in C Programming.
Variables are used in C programming to store many types of data and information that may change during the execution of a C program. Program variables in the C language are declared with a specific data type, which determines what type of data value the program variable you declare can hold. In C, variables are declared in the program before they are used.
// Declaration and Initialization of Variables in C Language
int k = 7;
float temperature = 100;
char text = ‘T’;
In the above example:
k is an integer variable that stores k variable values.
temperature is a floating-point data type variable that stores temperature values.
text is a character variable that stores a single text value.
Constants in C Language.
Constant data type is used to store the values that do not change the constant program value during the execution of a C program. They are manually declared using the const keyword in C language, and always remember that constant program value values can never be modified once they are assigned.
// Declaration of constants in C language
const int decimal = 230;
const float salary = 1789.45;
const char info = ‘a’;
In the above example:
decimal is a constant declared variable. which stores decimal value.
salary is a constant which stores the declared salary value.
a is a constant variable which stores a single a character variable value.
Constants are helpful in defining those values which should not be changed even by mistake during program execution, it means if you have declared a constant variable in C programming then it cannot be changed, constants in C language are to improve program code readability and facilitate program maintenance.
Major difference between variable and constant.
Initialization – A variable declared in a C program can be initialized or changed at the time of declaration or later in the program, whereas constants in C programs are to be initialized at the time of program declaration, and can never be modified in the program after that.
Declaration – In C language variables are declared using a specific data type (e.g., int, float, char). While constant variables are generally declared using the const keyword, in C you can declare constant data types and names in many ways (e.g., const int, const float, const char).
Mutability – In C language program variables can be changed during program execution. While the constant value declared in a C program cannot be changed. If a constant variable is given a value in C language, it remains the same throughout the execution of the program. It can never be changed.