Using the INSERT INTO Statement
The INSERT INTO command statement in SQL table is used to insert new table records (rows), data and information in an existing table. It is one of the most commonly used SQL commands to add new data and information in a SQL table database. You can use the INSERT INTO command statement in any existing table to add a single data row or multiple table rows simultaneously to your existing SQL table.
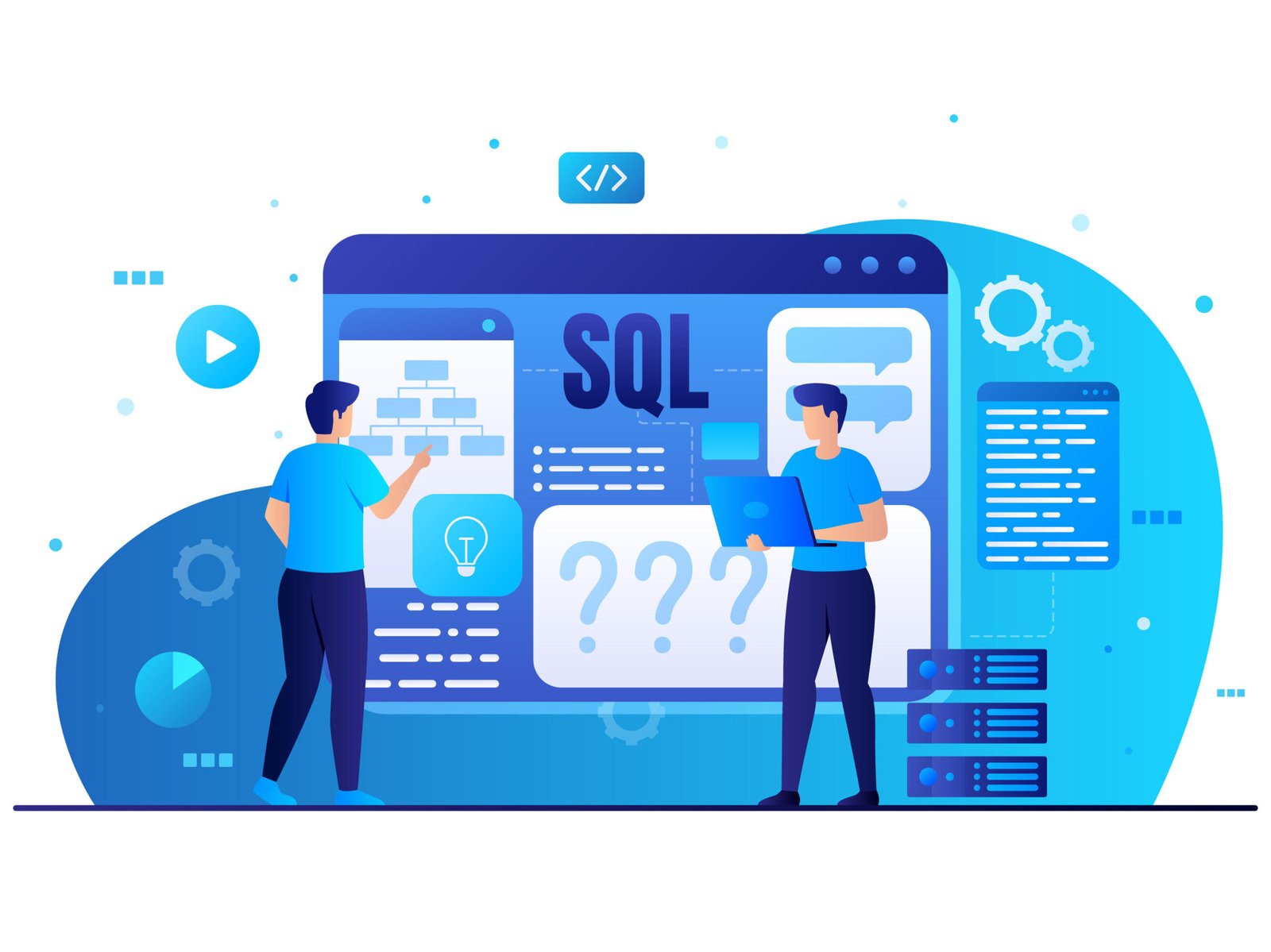
Syntax of INSERT INTO statement in SQL table.
You can use the INSERT INTO command statement in SQL table mainly for two purposes.
Inserting a single row in a SQL table.
To insert a single row of data in any SQL table, you can use this syntax.
INSERT INTO table_name (column1, column2, column3, …)
VALUES (value1, value2, value3, …);
- table_name – This is the name of the table in the SQL table where you want to insert the new data.
- column1, column2, ... – These are the names of the columns in the existing SQL table where the table column values will be inserted.
- value1, value2, … – These are the column corresponding values to be inserted into the columns in the existing inserted table.
Insert a single row into a SQL table Example.
INSERT INTO employe (first_name, last_name, age, department)
VALUES (‘Ajay, ‘Kumar’, 27, ‘IT’);
Here in this example above.
Here the INSERT INTO command statement inserts a new row of information into a table named employe.
Where ‘Ajay’, ‘Kumar’, 27, and ‘IT’ are the employee table values inserted into first_name, last_name, age, and department columns respectively.
Inserting multiple rows into a SQL table.
You can insert multiple rows of data and information into an existing SQL table at once.
The multiple table row data insert statement syntax is.
INSERT INTO table_name (column1, column2, column3, …)
VALUES
(value1x, value2x, value3x, …),
(value1y, value2y, value3y, …),
(value1z, value2z, value3z, …);
Inserting multiple rows into a SQL table example.
INSERT INTO employe (first_name, last_name, age, department)
VALUES
(‘Vinay’, ‘Sharma’, 21, ‘IT’),
(‘Kunal’, ‘Verma’, 37, ‘Management’),
(‘Lalit’, ‘Kumar’, 22, ‘Development’);
In the above example.
Here in this example above.
The INSERT INTO command statement assumes that the order of the table values properly matches the order of the columns in the employee table.
Inserting data into a SQL table without specifying column names.
If you are inserting values into all columns of a SQL table, and the table values properly match the column order in the schema, you can insert table data and information directly by omitting the column names.
Example of inserting data information directly into a SQL table.
INSERT INTO employe
VALUES (‘Jatin’, ‘Sukla’, 28, ‘Designer’);
Here in this example above.
The INSERT INTO command statement assumes that the order of the table values properly matches the order of the columns in the employee table.
Inserting data from another SQL table.
You can also insert data and information into a table based on the result of a SELECT query from another SQL table. You can use it as INSERT INTO … SELECT command statement.
Insert another SQL table syntax.
INSERT INTO table_name (column1, column2, …)
SELECT column1, column2, …
FROM another_table
WHERE condition;
Here in this example.
INSERT INTO employees_backup (first_name, last_name, age, department)
SELECT first_name, last_name, age, department
FROM employees
WHERE department = ‘Development’;
Here in this example above.
Here above data is copied or inserted from employe table to employees_backup table.
Where only employee records of Development department are inserted in backup table.
Inserting default values in SQL table.
If a column in a SQL table has default values defined, and you do not provide any values for that column, the default values will be automatically inserted in the existing table.
In this example.
If the employee table has a hire_date column with default values of the current date, you can insert a new employee column without indicating the hire_date column, and it will automatically use the default values.
INSERT INTO employe (first_name, last_name, age, department)
VALUES (‘Naveen’, ‘Jain’, 24, ‘Developer’);
In this case above here.
Here if hire_date is defined in the table schema, it will be automatically populated with default values (e.g., current date).
Inserting NULL values into SQL tables.
You can also insert NULL values into any column in SQL tables. This option is useful when you explicitly want to keep a table column empty.
Here in this example above.
INSERT INTO employe (first_name, last_name, age, department, hire_date)
VALUES (‘Lalit’, ‘Kumar’, 40, ‘Development’, NULL);
In this example above.
In this example here, the hire_date column is set to NULL values.
You can insert NULL column values into any column as per your table requirement, which allows you to insert null values.
Explanation of INSERT INTO statement.
Variation | Description |
Basic Insert (Single Row) | Use to Inserts a single row into a existing sql table. |
Insert Multiple Rows | Use to Inserts multiple rows in a single statement. |
Insert Without Column Names | Use to Inserts values assuming they match the order of the columns in the active SQL table. |
Insert from Another Table | Use to Inserts data into a table based on a SELECT query from another table. |
Insert Default Values | Use to insert Omits a column to use its default value. |
Insert NULL Values | Use to insert Inserts NULL values into columns that allow them. |
Insert with Duplicate Handling | Use to Handles duplicate keys by either ignoring or updating existing records. |
Insert into command conclusion in SQL table.
The INSERT INTO command statement in SQL table is the fundamental concept for inserting a new data row into a table database.
- Where you can insert one or more rows into a SQL table.
- Insert data into a SQL table directly or from another table.
- Insert table values with default values or NULL values into a SQL table.
- Use these methods to avoid duplicate values in a SQL table or to update existing table rows when necessary.