Syntax and examples of function declarations
Function declaration in JavaScript programming is a method to define and declare user defined custom functions. Where, the name of the function to be created by the programmer in JavaScript, the default parameter arguments in the function (if any declared) and the main block portion of the function are created. Where at the end of a program is the program code to be executed when the function is called.
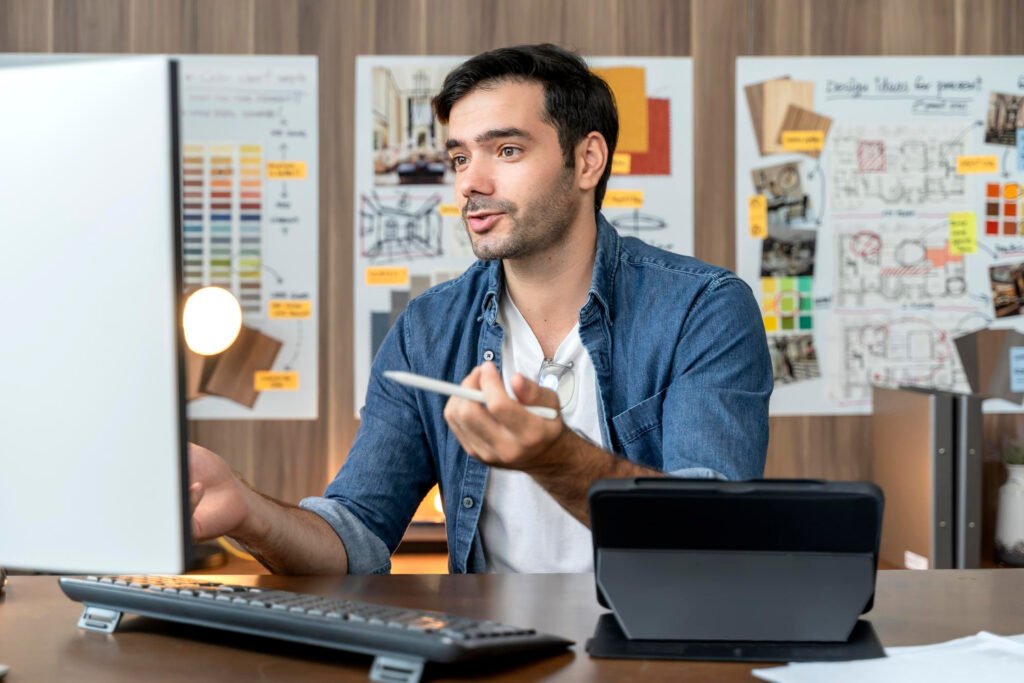
Syntax of function declaration in JavaScript.
function functionName(parameter1, parameter2, …) {
// write function code to execute
}
- function – This is a reserved keyword to define or create a function in any JavaScript program.
- functionName – This is the name of the custom user defined function in a program. Always user defined function must follow the rules and regulations of naming in JavaScript language. Like, do not create any empty space with a function, the function should not be started with any number.
- parameter1, parameter2, … – The function declared in JavaScript program can also be empty, if the function declaration has parameter arguments (optional). Which you can input as function input variables. These are the program variables of the function. Which pass the value with actual to formal arguments when calling this function.
- function body – This is the block of program source code inside the block {} in the current program, which will be executed later when the program function is called.
JavaScript basic function declaration example.
function vcanhelpsu() {
console.log(“welcome to vcanhelpsu!”);
}
vcanhelpsu(); // it Calling the vcanhelpsu function
Explanation of the above function.
- In the above custom function, the name of this function is vcanhelpsu, and this function does not take any parameter value input from the default user.
- When the vcanhelpsu function is called, it prints the message “welcome to vcanhelpsu!” on the console.
Example of function declaration with parameters in a JavaScript program.
function total(p, q) {
return p + q;
}
let output = total(1, 2); // it Calling the total function with arguments 1 and 2
console.log(output);
Explanation of the above function.
- Here the total function inputs two parameters p and q argument values by default, adds these two together in the function, and displays the result
- Where the total function is called with argument 1 and 2 as numeric values, and the function return value 3 as the result is displayed.
Function example with default parameters.
Here you can set the default value for the function parameter. If no value is passed while calling the function in the current program, then the default function value is used here.
function vcanhelpsu(text = “welcome”) {
console.log(“Hi, ” + text);
}
vcanhelpsu(“javascript”); // it Calling with vcanhelpsu function with an argument
vcanhelpsu(); // it Calling vcanhelpsu function without an argument
Explanation of default function parameters.
- Here vcanhelpsu function has a default value of “welcome” set for the name parameter.
- Here if you pass a text value as a function argument, then it uses that function value, otherwise, it defaults to “welcome”.
Function example returning a value in JavaScript.
In a JavaScript program, a function can return a value that can be used in other block portions of the current function program.
function product(p, q) {
return p * q;
}
let output = product(3, 4);
console.log(output); // it Calling product function and printing the return value
Explanation of function returning.
- Here the product function returns the product value of p and q variables.
- Where the output of calling product(4, 3) is 12, which is printed in the console screen.
Function with multiple parameters example in JavaScript.
function information(name, age) {
console.log(“Hello my name is ” + name + ” and my age is ” + age + ” year old.”);
}
information(“Siddhi”, 14); // it Calling the information function with arguments “Siddhi” and 14
Function with multiple parameters explanation.
- Where the information function takes two parameter value input by default. Which is indicated as name and age.
- It prints a text statement giving individual information along with the given function logic.
JavaScript function without return value (void function) example.
If you explicitly return a function value in a program, then the function returns undefined value by default.
function logout(name) {
console.log(“Now you Logout, ” + name + “?”);
}
let output = logout(“Bhavishi”); // it Calling the logout function and print statement
console.log(output); // here output will be the undefined
Explanation of function without return value.
- Here the logout function just prints a message on the console, without returning anything.
- When the result of the logout function call is stored in the result, it is undefined, then this function does not return any value.
JavaScript function expression vs function declaration example.
In a JavaScript program, the function declaration is hoisted, so it exists before it is defined in the program code, but there is no function expression.
Expression vs. Function Declaration.
JavaScript Function Declaration:
test(); // here test function Can be called before its declaration because it’s hoisted
function test() {
console.log(“This is, Test Function!”);
}
JavaScript Function Expression.
// This will throw an error because function expressions are not hoisted
testFunc(); // Display Error- testFunc is not a function
var testFunc = function() {
console.log(“this is test func expression!”);
};
Expression vs. Function Explanation.
- In a function declaration, the function is hoisted, so it can be called before the program code is executed.
- Whereas in a function expression, the function is assigned to a variable, and now it can be called only after the function is defined.
Summary of Functions in JavaScript.
A function declaration in JavaScript programming is a method of defining a user-defined custom function that can be called later in any program if needed in the JavaScript program.
The JavaScript function syntax is.
function functionName(parameters) {
// create custom function code to execute
}
A JavaScript function can have parameters and function return values.
Function hoisting: JavaScript program function declarations are hoisted, which means they can be called before they appear in the program source code.
When to use function declarations in JavaScript programs.
Use function declarations in a JavaScript program when you want to define a reusable function program code block in a program. Which can be called many times in a program.
JavaScript program function declarations are useful for creating such user-defined functions. Which is easy for the programmer to analyse, understand and test as per the need.