Python Sets
Sets in Python programming are an unordered storage collection of unique elements. Set data types declared in Python programs can be modified at any time, which means you can add new set elements or remove old set elements from previously declared set data type elements. Additionally, you can perform tasks like testing membership in set elements, removing duplicate elements in an existing set sequence, and unioning set elements, intersecting unique set elements, as well as applying set mathematical operations like difference and symmetric difference.
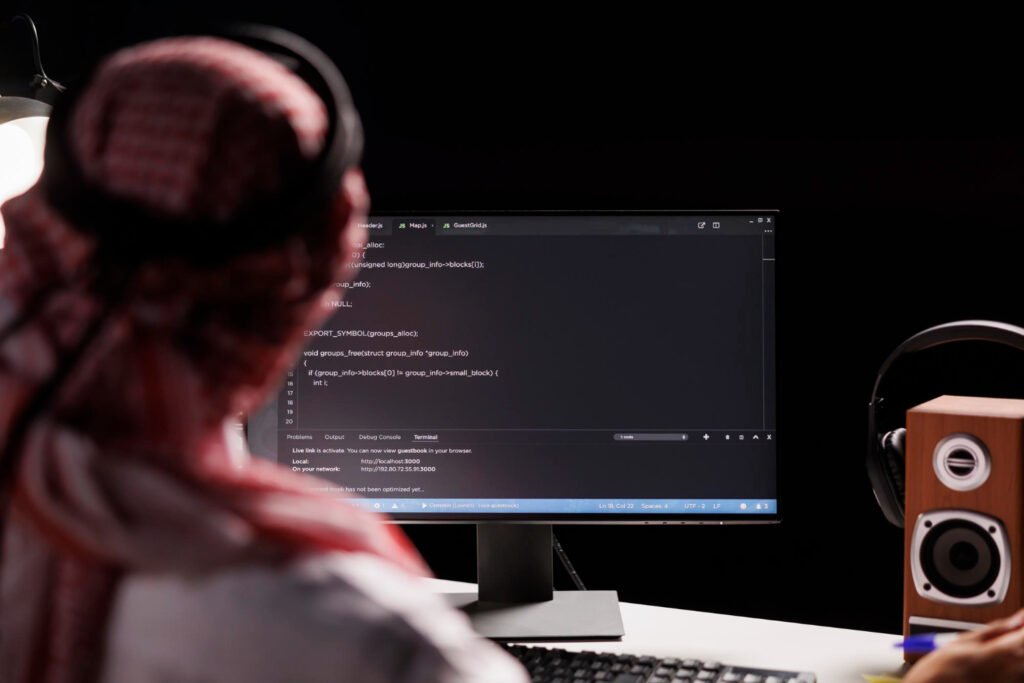
Creating a New Set in Python.
Python programmers can create a set element in a program using left and right curly braces {} with comma-operator separated set element values or using the set() constructor with a repetition (such as a list or tuple).
# Declaring a set using curly braces in Python program
test_set = {7, 9, 1, 5, 8, 3, 0 }
# Creating a new set using set() constructor in Python
sample_set = set([4, 7, 3, 9, 10, 1])
Basic operations on sets in Python programs.
Adding elements to a set – The add() method is used to add a new element to a set in a Python program, and the update() Python method is used to add multiple set elements (any other repetition) at once.
test_set.add(2)
print(test_set) # the result is – {7, 9, 1, 5, 8, 3, 0 }
sample_set.update([11, 12, 15])
print(test_set) # the result is – {7, 9, 1, 5, 8, 3, 0, 11, 12, 15 }
Deleting Set Elements in Python – In Python programming, Python methods like remove(), discard(), or pop() are used to remove elements from a set.
test_set.remove(0)
print(my_set) # the result is – {7, 9, 1, 5, 8, 3, 0 }
test_set.discard(15)
print(test_set) # the result is – {7, 9, 1, 5, 8, 3, 11, 12}
popped_element = test_set.pop()
print(test_set) # the result is – { 9, 1, 5, 8, 3, 11, 12}
print(popped_element) # the result is – { 9, 1, 5, 8, 3, 11, 12} (randomnly remove set pop element 7)
Operations on Python Sets – Apply set operations like set union (|), intersection (&), difference (-), and symmetric difference (^) in Python programs We can do it.
setgroup1 = {10, 9, 8, 7, 6}
setgroup2 = {5, 4, 3, 2, 1}
# let print two different set union element
print(setgroup1 | setgroup2) # the result is – {1, 2, 3, 4, 5, 6, 7, 8, 9, 10}
# Python set intersection setgroup1 = {10, 9, 8, 7, 6,5} 2 = {5, 4, 3, 2, 1,10}
# let print two set difference element
setgroup1 = {10, 9, 8, 7, 6,5}
setgroup2 = {5, 4, 3, 2, 1,10}
print(setgroup1 & setgroup2) # the result is – {10, 5}
# let print two set difference element
setgroup1 = {10, 9, 8, 7, 6,5}
setgroup2 = {5, 4, 3, 2, 1,10}
print(setgroup1 – setgroup2) # the result is – {8, 9, 6, 7}
# let print two set symmetric difference element
print(setgroup1 ^ setgroup2) # the result is – {1, 2, 3, 4, 6, 7, 8, 9}
python set membership testing – Use in keyword in set to check whether any set element is present in the declared set in Python program.
print(10 in setgroup1) # the result is – True
print(21 in setgroup2) # the result is – false
Different Python Set Methods.
Sets in Python program support various types of methods for general operations. So let’s get to know some commonly used sets methods in Python programming.
python sets methods.
add() – Using the add() method adds a new set element to an existing Python set.
remove() – The remove() set element method removes an existing element from a Python set. Here if the set element does not exist, it displays KeyError.
discard() – Discard() removes an existing set element from a Python set, if the set element exists in the set. So,
pop() – Removes a desired set element from the existing Python set, and displays the new setlist.
clear() – Removes all the set elements from the Python program set.
copy() – Displays a duplicate copy of the existing Python set.
union() – Displays a new set by combining the elements of both the sets in the Python program.
intersection() – Displays a new set of values with the elements common to both Python sets.
difference() – Displays a new set of values with the elements in the existing Python set that are not present in the other set.
symmetric_difference() – Displays a new set of values with the elements in one of the Python sets that are not present in both sets.
Python Frozen Set.
In Python programming you get a new set type called frozenset which is not to be modified in Python programs and can be hashed. Frozenset behaves by default like a set element in Python but after frozenset creation in Python programs it cannot be modified again. It can be used as a key in dictionaries or as an element of another set.
test_frozenset = frozenset([7, 9, 8, 3, 2, 1]) # test name frezenset created
sample = frozenset([7, 9, 8, 1])
print(“\n”,test_frozenset) # the result is – frozenset({1, 2, 3, 7, 8, 9})
print(“\n”,sample) # the result is – frozenset({8, 9, 1, 7})