Python in Lists
List data type is a universal and fundamental data structure storage element in Python programming language. It allows Python programmers to store and manipulate the storage of data items in a sequential order. Remember that list items declared in Python program are modifiable at any time, which means you can modify the list elements anytime after declaration of list elements in Python program.
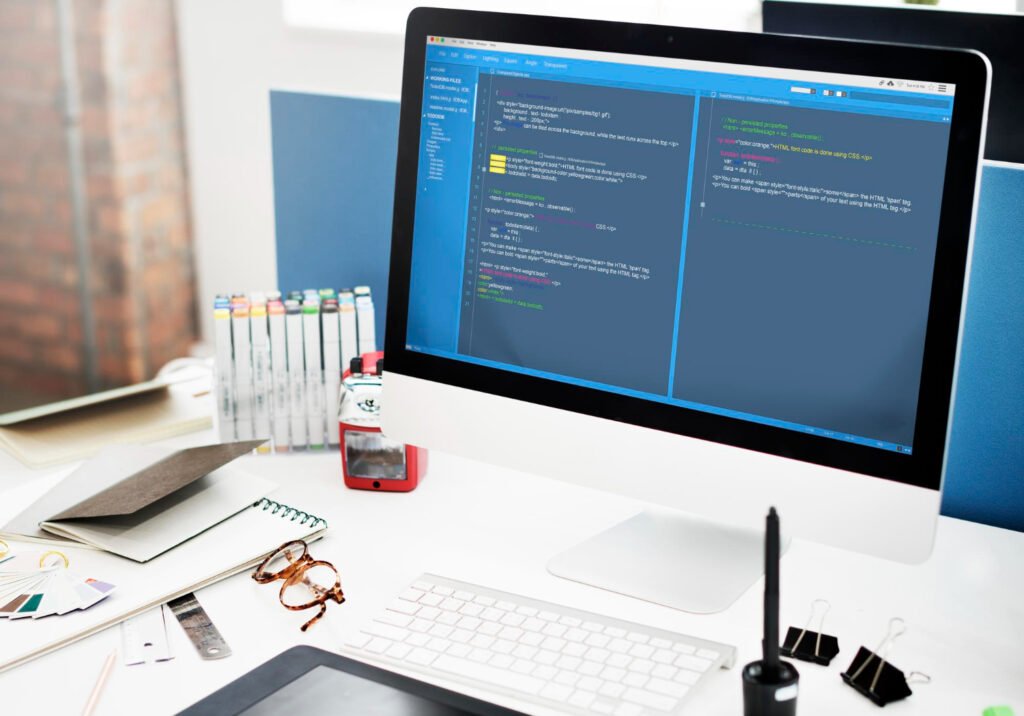
So let’s understand list elements in Python programming in a better way.
Creating Lists in Python.
In any Python program, you represent list elements one by one with comma operator inside square brackets []. All list elements represent different list element values in the list.
# Creating a list of integers in Python
integer = [9, 11, 55, 98, 76, 88, 90 ]
# Creating a list of strings in Python
laptop = [“apple”, “hp”, “dell”, “lenovo”, “acer”, “asus”]
# Creating a mixed-type list element in Python program
group_lists = [9, “vcanhelpsu”, 999.89, false]
Accessing list elements in Python.
You can access individual storage elements of existing lists in your current program by using square brackets [] and the index storage location of the list element (where each list index location sequence will start from 0).
courses = [“python”, “java”, “css”, “html”]
print(courses[0]) # result is – “python”
print(courses[1]) # result is – “java”
print(courses[-1]) # result is – “html” (display last element with negative indexing)
Slicing lists in Python program.
In Python program, you can extract an element or part of declared lists using slicing method. Using string slicing, you can slice and display any particular element between list 0 (zero element) and last list index location.
decimal = [55, 66, 77, 88, 89, 99, 100, 44, 33, 22]
print(decimal[2:7]) # result is – [77, 88, 89, 99, 100]
print(decimal[:4]) # result is – [55, 66, 77, 88]
print(decimal[4:]) # result is – [89, 99, 100, 44, 33, 22]
print(decimal[::3]) # result is – [55, 88, 100, 22]
print(decimal[::-2]) # result is – [22, 44, 99, 88, 66]
Modifying Python Lists.
Python lists are modifiable at any time, so you can modify list elements at any time, add new list elements, insert new list elements, delete existing list elements, and apply many other list operations.
courses = [“java”, “python”, “html”]
# it is used to pdate an element in the list with css
courses[1] = “css”
print(courses) # result is – [‘java’, ‘css’, ‘html’]
# it is used to Add an element to the list with javascript
courses.append(“javascript”)
print(courses) # the result is – [‘java’, ‘css’, ‘html’, ‘javascript’]
# it is used to Insert an element at a specific index in the list
courses.insert(1, “c”)
print(courses) # the result is – [‘java’, ‘c’, ‘css’, ‘html’, ‘javascript’]
# it used to Remove an element based on value in the list
courses.remove(“java”)
print(courses) # the result is – [‘java’, ‘c’, ‘css’, ‘html’, ‘javascript’]
# it is uded to Remove an element based on index in the list
del courses[0]
print(courses) # the result is – [‘css’, ‘html’, ‘javascript’]
Python List Operations.
You can apply various operations on declared list elements in Python program. Such as list element addition (+), list iteration (*), checking list members (in), and finding list length (len()).
lista = [99, 88, 77, 66]
listb = [34, 56, 90, 100]
# it used to join two list elements in Python
join = lista + listb
print(join) # the result is – [99, 88, 77, 66, 34, 56, 90, 100]
# it use to mutate the list elements
mutate = lista * 4
print(mutate) # the result is – [99, 88, 77, 66, 99, 88, 77, 66, 99, 88, 77, 66, 99, 88, 77, 66]
# it used to existing List member checking
print(88 in lista) # result is – true
print(100 in listb) # result is – true
# it used to Finding length of Python element
print(len(listb))
Python list comprehensions.
Python list comprehensions provide a concise method of creating new lists based on existing lists or other iterable objects.
# below square used to display square root of number between 2 to 10 with for loop range
sqrt = [p**2 for p in range(2, 10)]
print(sqrt) # the result is – [4, 9, 16, 25, 36, 49, 64, 81
# here creating an even number with if statement or for loop range
evennum = [x for x in range(2, 16) if x % 2 == 0]
print(evennum) # the result is – [2, 4, 6, 8, 10, 12, 14]
Nested Lists in Python Program.
Python lists can contain lists inside other lists as elements. This allows you to use lists to create multi-dimensional data structures.
table = [[100, 300, 200], [700, 800, 900], [400, 1000, 1100]]
print(table[0][1]) # the result is – 300 (reach the element at row 0, and column 1)
print(table[1][2]) # the result is – 900 (reach the element at row 1, and column 2)
Lists are an incredibly universal data structure method in Python programming, and are a fundamental part of processing data in list order in Python programming. Python lists are used extensively in data manipulation, program algorithm development, and various application development.