Polymorphism in pythons
Polymorphism features is a fundamental programming concept in object-oriented programming (OOP) and Python. It uses multiple class objects as a common superclass objects. Here Python polymorphism features makes the class code more accessible and flexible by defining methods in the superclass and overriding them in the subclasses.
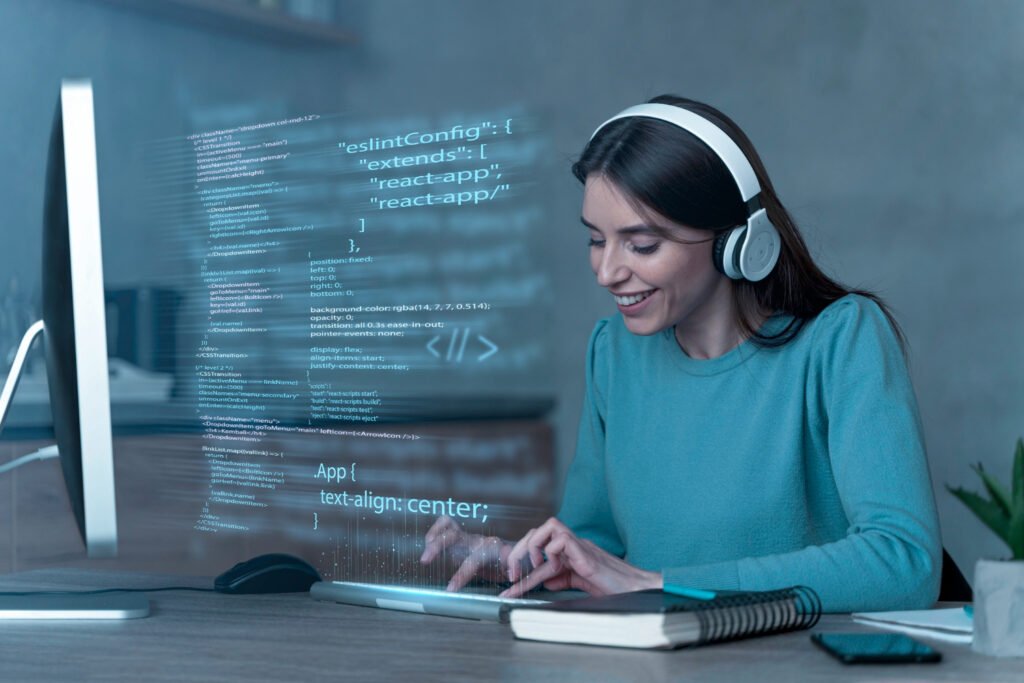
Let us understand polymorphism in Python programming better.
Understanding Polymorphism in Python.
The term polymorphism in computer programming is derived from the Greek words “poly” (many) and “morph” (form). It represents the ability to use different similar class objects as instances of the same class through a common programming interface (method names).
Example of Polymorphism in Python.
Consider a simple example using base classes Python and subclasses Python, Java, and HTML, each of which overrides a common method.
class programming:
def select(course):
raise NotImplementedError(“\n raise error when class polymorphism execute”)
class python(programming):
def select(course):
return “python!”
class java(programming):
def select(course):
return “java!”
class html(programming):
def select(course):
return “html!”
# use function to show polymorphism
def select_course(programming):
return programming.select()
# Creating instances of python, java and html
python = python()
java = java()
html=html()
# use function to display polymorphism class result
print(select_course(python)) # result is – python
print(select_course(java)) # result is – java
print(select_course(html)) # result is – html
In the above polymorphism example, Program is the base main/super class with an abstract select() method.
Python and Java, HTML are subclasses of the Python class that override the select() method with outputs (“Python!” in Python and “Java!” in Java) upon their specific execution.
The function select_course() exhibits polymorphism by accepting any object of the class Python (or its subclasses) and calling its select() method, which produces different class results depending on the realtime type of the object (Python, Java, or HTML) depending on the class Python.
Advantages of Polymorphism Programming in Python.
Program flexibility – Polymorphism in Python programming allows such program code. Which allows Python programmers to work with multiple types of objects at a time, and use them uniformly through a common program interface.
Program code reusability – Polymorphism in Python allows methods to be reused across different classes, reducing source code duplication in your program, and increasing large complex program maintenance.
Program extensibility – Polymorphism enables you to create new classes that conform to existing interfaces (methods), without modifying existing Python program code.
Polymorphism in Python programming.
Python programming naturally supports polymorphism features through its dynamic programming nature typing and the ability to resolve method calls at program runtime based on the realtime type of the object.
Key points of polymorphism.
Method overriding – Subclasses in a Python program can override methods of their superclasses to provide special behavior while maintaining a common method signature.
Duck typing – Python’s philosophy of “duck typing” in Python forces the behavior of an object on its type, meaning that as long as a class object enables a required method, it can be used with other class objects that have the same class method signature.
Example of duck typing in Python.
class course:
def select(choice):
return “Select course”
class programming:
def select(choice):
return “Select Programming”
# use function use with select method
def choose_method(method):
return method.select()
course = course()
programming = programming()
print(choose_method(course)) # result is – select course
print(choose_method(programming)) # result is – select programming
In the program example above.
Both the course and programming classes have a select() method declared.
The function choose_method() accepts any object (course or programming) that has a select() method, and calls it in the program, demonstrating polymorphism through duck typing.