Pointer to functions
A pointer to function in C program is a type of pointer memory address location variable. Which stores the memory address of the function argument/parameter instead of a program variable declared in the C program. Pointer to function is especially useful in programming conditions where you want to pass an argument or parameter in the current function program as an argument to another function through call by reference. When you want to return a function from a function in a C program. In C language, a function runs a large program code by dividing it into small modules. Using a pointer to function makes function calling fast and error free.
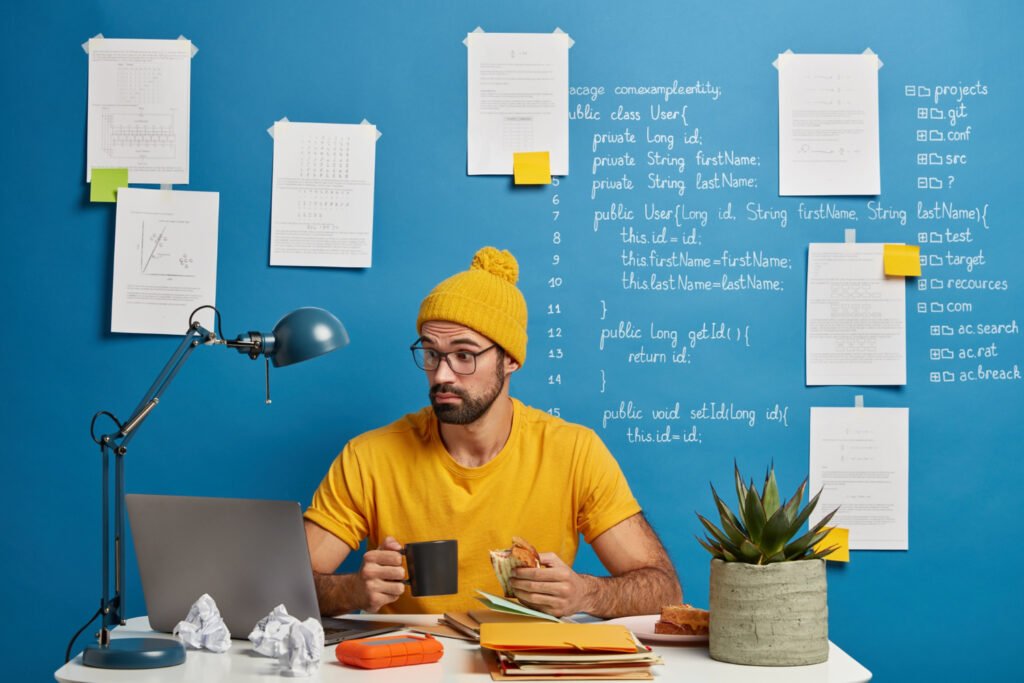
Declaring function pointers in C.
To declare a function pointer in a C program, the function to pointer syntax consists of declaring the function return type, function argument/parameter type and pointer name in the appropriate brackets.
// Example – below function pointer to declare function with two integer and their returns type as int data type
int (*testPtr)(int, int);
Assigning a function to a pointer in C.
In a C program, programmer can declare a function by using the name of the function without brackets and assigning a pointer to the function.
int total(int p, int q) {
return p + q;
}
int (*testPtr)(int, int) = total; // This code assigns ‘testPtr’ to ‘total’ function
Calling function through pointer in C.
You can call function arguments/parameters declared through pointer variable in C program just like you call normal function directly in C program.
int addition = testPtr(1, 2); // Calls ‘addition(1, 2)’, outputs the result – 3
Function pointer as function parameter in C.
You can pass function pointers as argument parameters to other functions in a C program. This allows you to operate on a function with flexible function callbacks or operations.
void test(int (*total)(int, int), int p, int q) {
int output = total(p, q);
printf(“\n the output is – %d”, output);
}
test(total, 1, 2); // This calls ‘add(1, 2)’ using the ‘total’ pointer
Returning pointers from functions in C.
Functions in C can return function pointer arguments. Which can be used to create pointers to functions in a function program or for different function operations.
int minus(int p, int q) {
return p – q;
}
Array of function pointers in C.
You can create an array of function pointers to represent a list of functions in a C program. Pointer variables can be used to create user defined functions by running the array elements in a loop.
int mul(int p, int q) {
return p * q;
}
int (*process[5])(int, int) = { multiply };
for (int p = 0; p < 5; p++) {
printf(“\n %d”, process[p](3, 2)); // This calls each function in the array
}