new and delete operators c++
In C++ programming, new and delete operators are used for program memory management in the form of dynamic memory allocation and memory deallocation. Where new and delete operators perform memory management process in the heap opposite to the program data storage stack, and play an important role in creating objects and arrays and deleting old array elements during the execution of any C++ program.
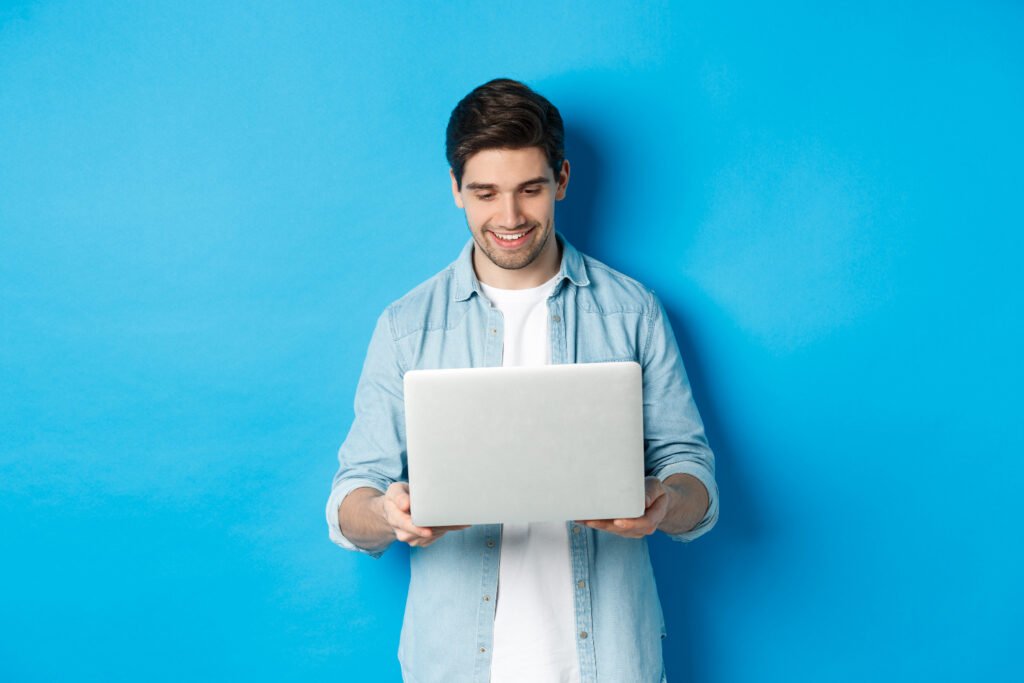
new operator in C++ programming.
In any C++ program, new operator allocates or creates memory from the heap for the current program, and moves it to a user generated pointer position. In C++ programs, new operator can be used to allocate the required memory byte location for a single object or an array of objects.
new operator syntax in C++ programming.
single objet:
P* ptr = new P; // it Allocates memory with new operator for object of type P
Array of Objects.
P* ptr = new P[n]; // It Allocates memory with new for an array of n objects of type P
Example of new operator in C++ programming.
#include <iostream>
class TestClass {
public:
TestClass() { std::cout << “\n Constructor operation perform”; }
~TestClass() { std::cout << “\n Destructor operation perform”; }
};
int main() {
// it allot memory for single object
TestClass* delobj = new TestClass(); // Constructor operation perform
// it allot memory for array of objects
TestClass* array = new TestClass[4]; // here Constructor call each element
// it deallocate memory using ‘delete’ operator
delete delobj; // here Destructor is call single obj
delete[] array; // here Destructor is call all object in the array loation
return 0;
}
delete operator in C++ programming.
In any C++ program, delete operator is used to free the heap memory space location allotted by the new operator. Here you can see that when you use delete operator in C++ program, the destructor of the object automatically calls it.
Delete operator syntax in C++ programming.
Single Object:
delete ptr; // it Deallocates memory with delete operator for the single object
Array of Objects.
delete[] ptr; // it Deallocates or delete memory with delete for the array of ob jects
Example of delete operator in C++ programming.
#include <iostream>
class TestClass {
public:
TestClass() { std::cout << “\n Constructor process done”; }
~TestClass() { std::cout << “\n Destructor process done”; }
};
int main() {
TestClass* delobj = new TestClass(); //here the new operator allot Memory dynamically
delete delobj; // here use delete operator as Destructor to called and memory and free them
TestClass* array = new TestClass[3]; // create new operator Memory with 3 array objects element
delete[] array; // here delete operator Destructor call with each object array and memory will be free
return 0;
}
Main points of delete operator.
Facts about memory allocation and deallocation in C++ program.
- The new operator in C++ program allocates memory for any program from the heap, and returns it at the pointer location.
- The delete operator in C++ program provides memory deallocation features for a single program object, it frees the previously allocated memory location space.
- The new[] operator allocates memory for object array in the current C++ program.
- The delete[] operator frees the memory deallocation for object array or previously allocated array block memory element location space in the current C++ program.
Memory error management in C++ programs.
If the new operator is unable to allocate memory in your current program, then it throws std::bad_alloc exception in that program. Unless you use it with the nothrow version of the new operator, such as new(std::nothrow).
If the pointer in the current C++ program is nullptr, then the delete operator does not perform memory loss. So, you do not need to test before calling delete operator in that program.
Double free error in C++ program.
Calling delete operator more than once on a pointer in a C++ program or calling delete[] operator on a non-array pointer can cause abnormal array program behaviour. First of all, you should make sure that you delete each object or array element in the current array program exactly once.
Memory leak in C++ program.
When you allocate new memory location space with new operator in the current C++ program, while you do not deallocate that allocated memory location with delete operator, then you can cause allocated memory leak in that program. So, you should avoid such errors in your current program. To avoid causing memory leaks, you must deallocate the dynamically allocated memory in your program.
Example of memory leak in C++ program.
#include <iostream>
class TestClass {
public:
TestClass() { std::cout << “\n Constructor process done”; }
~TestClass() { std::cout << “\n Destructor process done”; }
};
int main() {
TestClass* delobj = new TestClass(); //here the new operator allot Memory dynamically
delete delobj; // here use delete operator as Destructor to called and memory and free them
TestClass* array = new TestClass[3]; // create new operator Memory with 3 array objects element
delete[] array; // here delete operator Destructor call with each object array and memory will be free
return 0;
}
Here in this program example given above, the memory allocated with the new operator is never freed. This will result in memory leak in the current program.
Using new and delete Safely in C++ program.
To avoid causing memory leaks in any C++ program, you must ensure that every memory allocated with the new operator has a corresponding add or delete operation applied. You can apply smart pointers, such as std::unique_ptr and std::shared_ptr, features from the built-in C++ standard library to automatically manage dynamic memory in your program and avoid the problems of manual memory management.