Macros in c
Macros are a programming art in C programming through the built-in preprocessor. Generally macros are used to define constant data types, program functions and code snippet elements in detail in C language, any macros code is defined and interpreted by the preprocessor in C language. If you want to use macros in C language, you must first define it using the #define preprocessor directive.
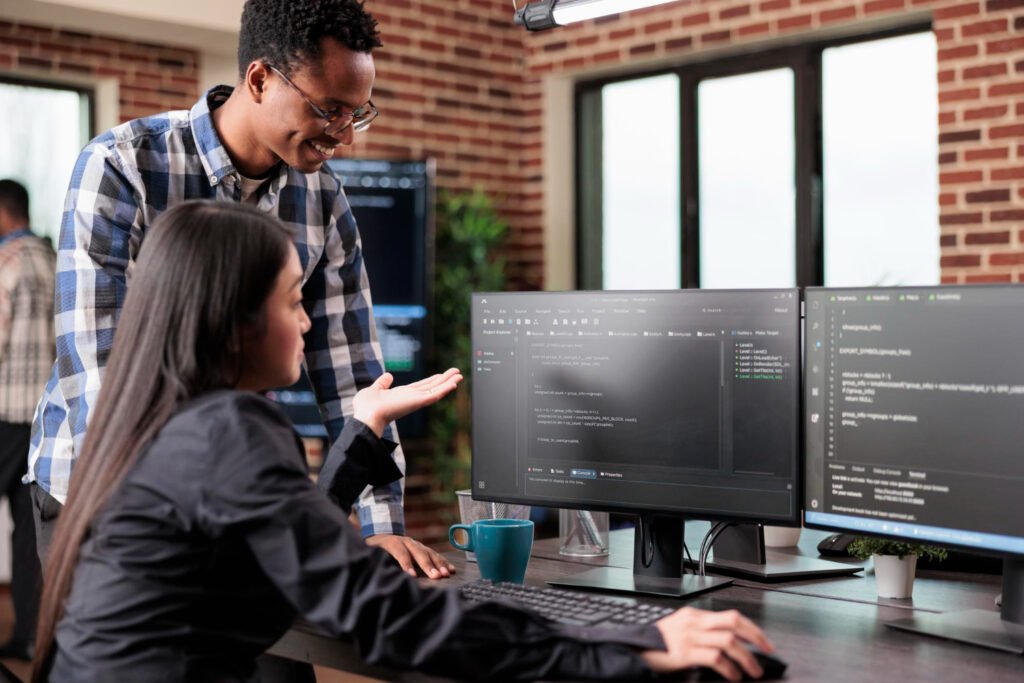
Basic Macros in C Language.
Defining Constant Macros in C Language.
Here you can use the macros data type to define constant elements in a C program. Remember that macros elements are typed in upper case characters to differentiate them from variables.
#include <stdio.h>
#define PI 3.14
#define MAXimumSIZE 1500
int main() {
printf(“\n Value of PI elements is – %f”, PI);
printf(“\n the Maximum size is – %d”, MAXimumSIZE);
return 0;
}
Defining Function Macros in C Language.
In a C program, if you need to make macros function-like, you can make macros inline functions. So you declare arguments or parameters for the macros, and define the function macros in place during preprocessing.
#include <stdio.h>
#define SQRT(p) ((p) * (p))
int main() {
int sqvalue = 9;
printf(“\n The Square root of %d is %d “, sqvalue, SQRT(sqvalue));
return 0;
}
Advanced Macros in C Language.
Macros Conditional compilation.
Macros in C programs can be used for conditional program compilation, where you control or manage the macros according to some particular condition in the program source code.
#include <stdio.h>
#define DEBUG
int main() {
int p = 2, q = 3;
#ifdef DEBUG
printf(“\n The Debug is – p = %d, q = %d\n”, p, q);
#endif
int count = p + q;
printf(“\n the count is – %d\n”, count);
return 0;
}
Include guards in C macros.
Include guards in C macros programs prevent multiple inclusions in the same header file, which can lead to macro program compilation errors.
#ifndef Type header file name in capital letter
#define Type header file name in capital letter
// display current Header file contents name
#endif // Type header file name in capital letter
Variadic Macros in C Programs.
Variadic macros in C programs allow you to define macros that accept a variable number of program argument parameters.
#include <stdio.h>
#define PRINTF(format, …) printf(format, __VA_ARGS__)
int main() {
PRINTF(“\n Single digit – %d”, 1);
PRINTF(“\n Two digit number – %d, %d”, 1, 2);
return 0;
}
Disadvantages of Macros.
Macros Security Type – Macros declared in C programs are not type tested.
Unnecessary side effects – Sometimes the unexpected behavior of macros in C programs can cause many problems in the program. If macros are not used carefully in C programs, especially when parameters or arguments are declared with function-like macros.
Modification Difficulty – Sometimes it becomes more complicated to extend, debug or make an error free macro in an existing C program.
Macros Advantage.
Always Use Macros Parentheses – Always remember to declare macro arguments and the entire macro definition in parentheses to avoid macro precedence problems.
#define SQRT(p) ((p) * (p))
Limit the use of macros in C – Always use macros carefully in C programs and prefer inline functions over macros when macro type security and program code debugging is important.
inline int sqrt(int p) {
return p * p;
}
Macros Consistent Naming – Always remember to use uppercase characters for declaring macro names in C programs. So that they can be easily distinguished from variables and functions.
#define MAXIMUMSIZE 1500
Macro usage examples widely used in C programs.
So let’s look at a C program macros here. In which various uses of macros are explained including common macros constants, function-like macros and program condition compilation.
#include <stdio.h>
// define Macro definitions
#define PI 3.14
#define MIN(p, q) ((p) < (q) ? (p) : (q))
#define DEBUG
// you can see Conditional compilation
#ifdef DEBUG
#define LOG(msg) printf(“DEBUG: %s\n”, msg)
#else
#define LOG(msg)
#endif
int main() {
// let Using a constant macro element
printf(“\n the Value of PI is – %f”, PI);
// here Using a function-like macro
int p = 1, q = 2;
printf(“\n The Minimum value of %d and %d is %d\n”, p, q, MIN(p, q));
// let Using a conditional compilation macro
LOG(“\n This is a debug message”);
return 0;
}