Introduction to Delegates
Declare delegate in C# programming language is a secured program function pointer. Delegates help programmers to store and access program methods in a dynamic order on program execution in C# programming. When you declare or define a delegate in C# programming language, you are defining a manual delegate type that can hold references to existing methods in C# programming language that have a special delegate method signature.
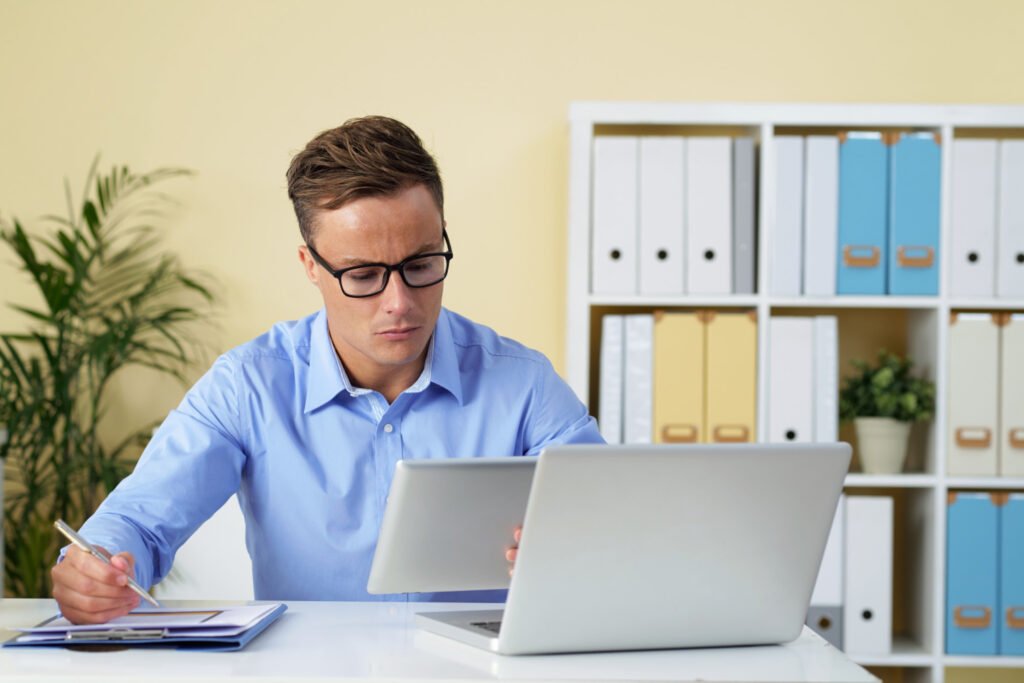
Why use delegates in C# programming language?
- Event handling – Delegates in C# programming language are commonly used to manage and control multiple individual events in a C# program.
- For example, event handlers in GUI(Graphical User Interface) frameworks like Forms in Windows environment and WPF are commonly executed using delegates.
- Callback methods – Delegates in C# programming language allow passing methods as program arguments, thereby enabling callback capability in a program structure.
- Flexibility – Delegates in C# programming language are calls to particular methods at program runtime, you can also modify it if needed at the time of program parameter calling.
Declaring delegates in C# programming language.
To declare a delegate in C# programming language, you need to indicate the signature of the class methods that the delegate will use as reference in a program. Such as (class method return type and class parameters or arguments).
Syntax of declaring a delegate in C# programming language.
public delegate returnType DelegateName(parameter/argument List);
For example here, the current program has a delegate declaration that indicates the methods in the active program that return void values and take input of a specific data type as a parameter or argument.
public delegate void TestDelegate(int numeric);
- delegate returnType – Defines the data type of the value to be returned by the delegate method.
- DelegateName – The current delegate type and its name in C# programming language.
- delagate parameterList – These are the program parameters (if any class parameters) that the delegate will accept in the current program.
Using delegates in C# programming language.
Once the delegate data type is defined in C# programming language, you can immediately create the delegate as per the requirement and bind it to a method. Next, the delegate can be used to call the bound methods in the current program.
Declaring and using delegate example.
using System;
public delegate void TestDelegate(int numeric); // Here testdelegate Declare as a delegate
public class sample
{
// Here We Declare Method use to matches the delegate signature
public static void DisplayNumeric(int numeric)
{
Console.WriteLine(“\n The Numeric value is – ” + numeric);
}
static void Main()
{
// Here we Instantiate delegate and bind it to displaynumeric
TestDelegate del = new TestDelegate(DisplayNumeric);
// Here it Call the method with delegate
del(79); // the result is – 79
}
}
del TestDelegate Here is a basic example of a delegate data type.
- PrintNumeric Here, if the existing method matches the signature of the delegate, we can now assign it to the delegate
- We invoke a method through a delegate using del(79) numeric.
Multicast delegate in C# programming language.
A multicast delegate declared in C# programming language can hold references to multiple methods. When the delegate is invoked in a program, it calls all the methods in a multicast delegate list in the current program.
Multicast delegate example.
using System;
public delegate void DemoDelegate(int numeric); // Demodalagate Declare as a delegate
public class Sample
{
public static void Displaynumeric(int numeric)
{
Console.WriteLine(“\n the default Numeric is – ” + numeric);
}
public static void Printadd(int numeric)
{
Console.WriteLine(“\n the addition is – ” + (numeric + 10));
}
static void Main()
{
// Declare Instantiate a delegate and bind multiple methods
DemoDelegate del = Displaynumeric;
del += Printadd; // Display Add another method
// Here it Call both methods with delegate
del(79);
// the result is –
// the default Numeric is – 79
// the addition is – 89
}
}
In the multicast delegate example.
- Here, the program is bound to both the delegate del PrintNumeric and Printadd.
- When del(79) is called, both the methods are executed in sequence order.
Important Notes in C# Programming Language.
- Here if one of the methods in multicast delegate in C# programming language throws an exception, then the remaining methods in the active program sequence will not be executed.
- Multicast delegate in C# programming language is used multiple times in the event handling process to indicate multiple listeners.
Summary of Delegate in C# Programming Language.
- Delegate in C# programming language is a program type that references program methods with a special signature.
- Multicast delegate in C# programming language can reference multiple methods and invoke them in sequence order in a program.
- Delegate in C# programming language is used in program callback methods, program event handling, and dynamic method invocation.
- C# provides built-in delegate data types such as Action, Func, and Predicate to programmers for a general overview of the programming language.