Error handling
In C Programming File Handling Error Management, particular file errors occurring during file operation in a C program are managed and controlled. Error management detects errors occurring during file operation. Many times, while opening a file, we can manage the errors of failures in file open, read, write, append operations.
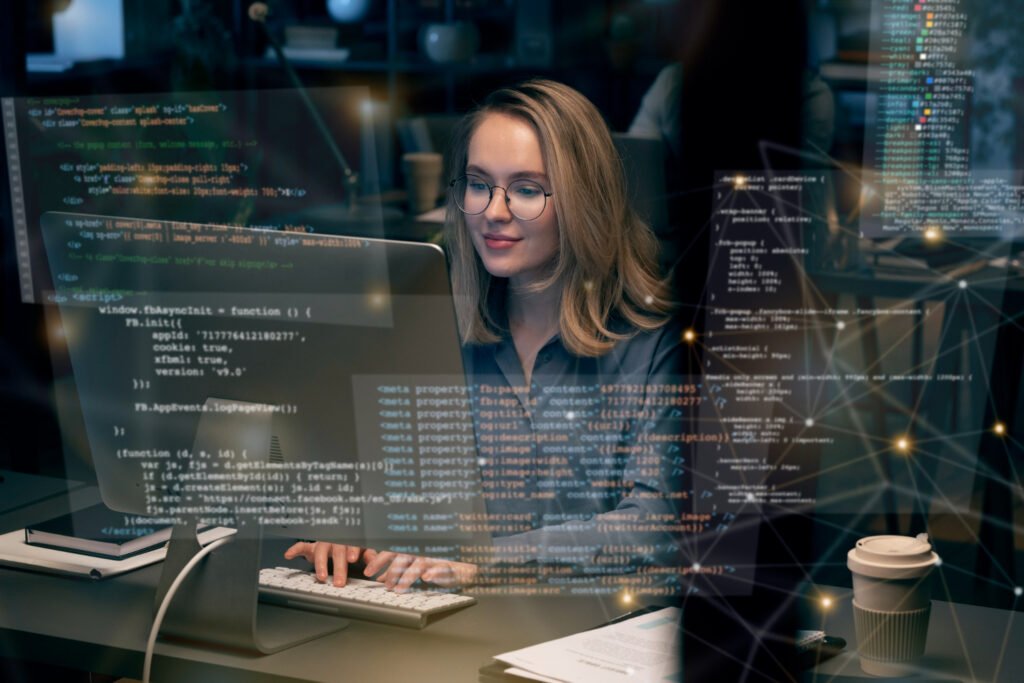
So let’s understand error management in C Programming File Handling in a better way.
Most of the time, when we have to take care while performing file handling operations, we should test the return value of the file tasks you use such as fopen(), fclose(), fread(), and fwrite() functions to see if they have succeeded or failed in the file operation.
File opening error example in C program.
If you open a file in read mode in the current program and due to some reason you cannot open the file, then in this condition the fopen() function returns NULL value.
FILE *file = fopen(“test.txt”, “r”); // open file in read mode purpose
if (file == NULL) {
perror(“you face Error opening file operation “);
return 1;
}
Closing the file in file handling.
Always remember that when you open a file in a file handling program. And when the file operation is completed, then fclose() function returns 0 if successful and EOF(end of file) value if unsuccessful.
if (fclose(file) != 0) {
perror(“you face Error when closing file”);
return 1;
}
Reading data from file in file handling.
In file handling, fread() function is used to read data from a file. fread() reads data and information from the file. If any false value is produced in reading this file, then this condition may result in error or file close condition.
size_t output = fread(buffer, sizeof(char), sizeof(buffer), file);
if (result != sizeof(buffer)) {
if (feof(file)) {
printf(“the cursor move End of file reached.\n”);
} else if (ferror(file)) {
perror(“otherwise Error reading file process”);
}
}
Writing to file in file handling.
If you want to write some text or information in the existing file, then use fwrite() function, this function returns the existing number of character value information in the file. Here if this command/code returns a value less than the requested values, then an error is generated in the file writing process.
size_t output = fwrite(buffer, sizeof(char), sizeof(buffer), file);
if (output != sizeof(buffer)) {
perror(“you face Error when writing to the file”);
return 1;
}
Error Handling Example of File Handling in C Programming.
Here you get a file handling example. Which demonstrates the error management code used in file handling for opening a file, reading a file, writing to a file and closing the file.
#include <stdio.h>
#include <stdlib.h>
int main() {
FILE *file;
// lets Open a file for writing operation
file = fopen(“test.txt”, “w”);
if (file == NULL) {
perror(“you can face Error opening file for writing operation”);
return 1;
} // lets Write to the content of the file
const char *info = “file, handling \n lets test the file operation\n”;
size_t output = fwrite(text, sizeof(char), strlen(info), file);
if (output != strlen(info)) { perror(“it caused Error writing to the file”);
fclose(file);
return 1;
} // Close the file if (fclose(file) != 0) {
perror(“if any Error closing file after writing to file”);
return 1;
} // lets Open the file for reading operation
file = fopen(“test.txt”, “r”);
if (file == NULL) {
perror(“you see the Error when opening file for reading purpose”);
return 1;
} // lets finally Read and print the content of the test file char value[256];
while (fgets(value, sizeof(value), file) != NULL) {
printf(“%s”, value);
} if (error(file)) {
perror(“you see the error reading from file”);
fclose(file);
return 1;
} // lets Close the file if (fclose(file) != 0) {
perror(“you see the error closing file after reading the file”);
return 1;
} return 0;
}
File handling library functions used in error management.
Opening a file – The fopen() function is used in file operations to write and read files and to open a file. If the fopen() operation fails, perror() prints an error message, and the program exits immediately.
Writing text to a file – The fwrite() function writes data and information to a file during a file operation. If it writes fewer bytes of information than the user expects, it prints an error message, the file writing process stops, and the program exits immediately.
Reading data from a file – The fgets() function in the file handling process reads text lines from a file. If an error occurs in reading file lines, ferror() prints an error message, and the program exits immediately.
Closing a file – The fclose() function is used in file handling to close a file. If this file operation fails, it prints an error message and the program exits immediately.