Conditional compilation in c
Conditional compilation in C allows you to add or remove features from macros or parts of program code based on certain conditions. This macros program is especially useful for computer platform-specific code, program debugging, and customizing code. Here are some preprocessor directives used for conditional compilation.
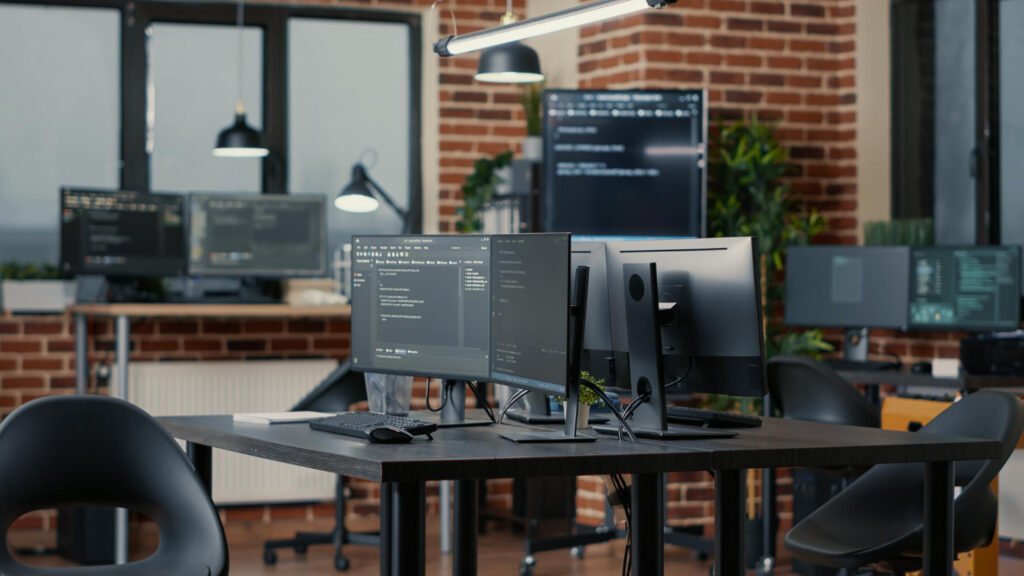
These are some popular conditional preprocessor directives.
#ifdef and #ifndef
#define and #undef
#if, #elif, #else, and #endif
Basic conditional compilation in macros.
Using #ifdef and #ifndef Preprocessor Directives
The #ifdef preprocessor directive checks whether a macro is defined.
The #ifndef preprocessor directive checks whether a macro is not defined.
#include <stdio.h>
#define DEBUG
int main() {
int p = 4, q = 7;
#ifdef DEBUG
printf(“\n The Debug is – p = %d, q = %d\n”, p, q);
#endif
int total = p + q;
printf(“\n the total is – %d\n”, total);
return 0;
}
Here in this example, the debug message is printed only if DEBUG is defined.
Using #if, #elif, #else, and #endif Preprocessor Directives in C Macros
The #if preprocessor directive checks if a condition is true or false.
The #elif preprocessor directive is the second condition test if the previous #if or #elif condition was false.
#else This gives you an option if none of the previous program conditions were true.
#endif This marks the end of the conditional block.
#include <stdio.h>
#define TEST 3
int main() {
#if TEST == 1
printf(“\n TEST 1\n”);
#elif TEST == 2
printf(“\n Test 2\n”);
#elif TEST == 3
printf(“\n Test 3\n”);
#else
printf(“Test other\n”);
#endif
return 0;
}
In the above example the output of the program depends on the value of test.
Macros Platform-specific code example.
Conditional compilation is often used in C macros programs to add platform-specific program code.
#include <stdio.h>
int main() {
#ifdef C
printf(“\n test on c Windows”);
#elif JAVA
printf(“\n text on java”);
#elif PYTHON
printf(“\n test on python”);
#else
printf(“\n no above platform supportd”);
#endif
return 0;
}
C macros debugging example.
You can use conditional program compilation to add debugging program code to a C program that may not be present in the release version.
#include <stdio.h>
void debugLog(const char *msg) {
#ifdef DEBUG
printf(” the DEBUG – %s\n”, msg);
#endif
}
int main() {
debugLog(“begain the program”);
int p = 4, q = 3;
int total = p + q;
debugLog(“the total count is”);
printf(“\n the total is – %d\n”, total);
debugLog(“let terminate the program”);
return 0;
}
C macros debugging example.
C macros can also use conditional compilation to add or exclude code for program optimization.
#include <stdio.h>
#define Let_OPTIMIZE
int main() {
#if LET_OPTIMIZE
printf(“\n This is Optimized code\n”);
// use Optimized algorithm
#else
printf(“\n This is the Non-optimized code”);
// Simple optimize algorithm declaration
#endif
return 0;
}
Use of #define and #undef in C macros.
#define creates a user defined macro.
#undef removes the macro definition.
#include <stdio.h>
#define DEBUG
int main() {
#ifdef DEBUG
printf(“\n program Debug mode is on”);
#endif
#undef DEBUG
#ifdef DEBUG
printf(“\n This statement no printed”);
#endif
return 0;
}
C Macros Include Guard Example.
If you want to prevent multiple inclusion of the same header file in a macros then use this.
#ifndef HEADER_FILE_NAME_H
#define HEADER_FILE_NAME_H
// Macros header file content
#endif // HEADER_FILE_NAME_H