Classes and Objects
Class and Object in C++ Programming.
In C++ programming, class and object are basic classes design and development programming fundamental concepts in object-oriented programming (OOP). A class in a C++ program is defined by developing base and multiple subclasses for an object, where an object in C++ is an instance of that class. Using classes in C++, OOP principles such as C++ class encapsulation features, abstraction, class inheritance, and class polymorphism are explored in basic and advanced C++ programming.
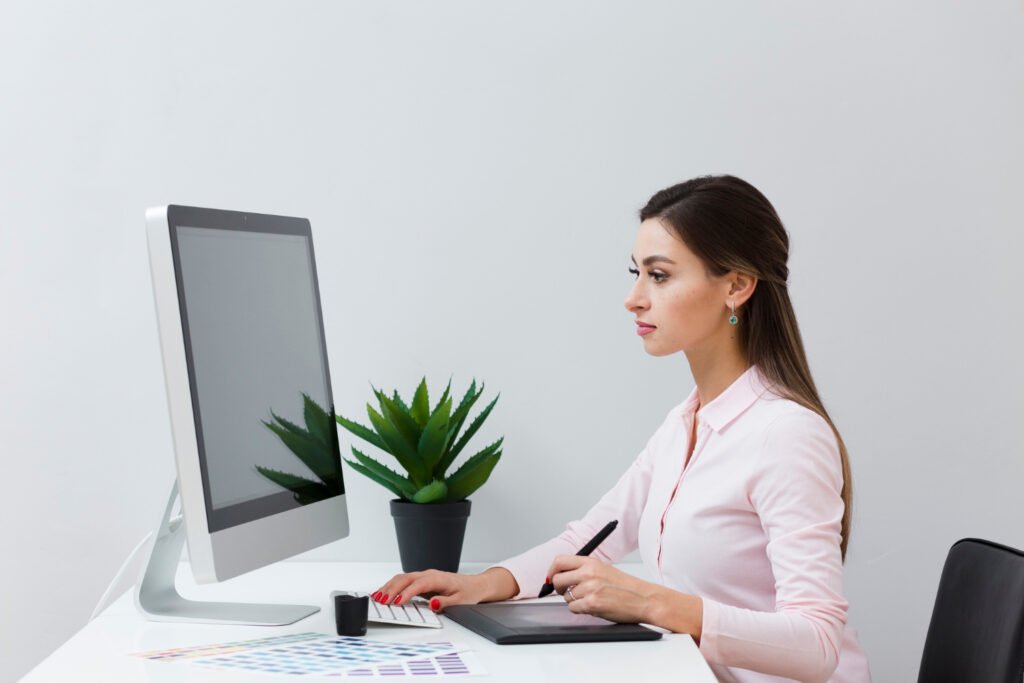
So, let’s understand class and object in C++ programming better.
Defining a C++ Programming Class.
A class in C++ programming is a user defined data type declaration method that contains custom user defined class variables, known as class data type members, and class functions, known as class member functions or class methods. Classes in C++ are created by grouping data variable types and class functions into a program. Which operate as a single entity group in the class data in the existing class program.
Syntax for defining a class in C++ programming.
class ClassName {
public:
// class Data members
type memberName;
// class Member functions
returnType methodName(parameters /argument)
{
// class function body
}
private:
// remember class Private members are only accessible inside cass
};
Class attributes in C++ programming.
- In C++ programming, public class members are accessed and processed externally in the class.
- In C++ programming, private class members are accessed and processed only from within the existing class. Where, by default, the existing class accesses private members.
- In C++ programming, protected class members are accessed and processed from within the class and through derived classes.
Creating objects in C++ programming.
Once you create a user-defined class data type in a C++ program, you can create the desired class object for that class. Where the object is an instance of the class, and each class object has its own data set condition.
Syntax for creating object in C++ program.
ClassName objectName;
Here you can create dynamic class object by using new class keyword in your existing class.
ClassName* objectPointer = new ClassName;
Defining class and creating object example in C++ programming.
Here you have an example of class member function to define class, create class object and access active condition of object in this program.
#include <iostream>
#include <string>
class employee {
public:
// Class Data members
std::string name;
int contact;
// Class Member function (method)
void empInfo() {
std::cout << “\n ========= Employee Detailed =========== ” << std::endl;
std::cout << “\n Employee name – ” << name << “, Employee contact – ” << contact << std::endl;
}
// Class Member function with parameters argument
void empInfo(std::string p, int q) {
name = p;
contact = q;
}
};
int main() {
// here we Create objects of the class
employee emp1;
employee emp2;
// apply values using member functions
emp1.empInfo(“Amit”, 94130);
emp2.empInfo(“Jay”, 98280);
// let Access and print data value using member functions
emp1.empInfo();
emp2.empInfo();
return 0;
}
Explanation in C++ programming.
- Class Definition – Here Employee class has two data members (employee name and employee contact) and two class member functions (empInfo and empInfo).
- Class object creation – Where in class main() function, two objects emp1 and emp2 are created from Employee class.
- Class function call – We use empInfo function to assign values to data members of emp1 and emp2 objects, and use empInfo object to print object values.