Character arrays vs. string literals
Character arrays and string literals are used in C programming to fulfill different programming tasks, and they have different string array properties. Especially they are used to solve some specific string operations.
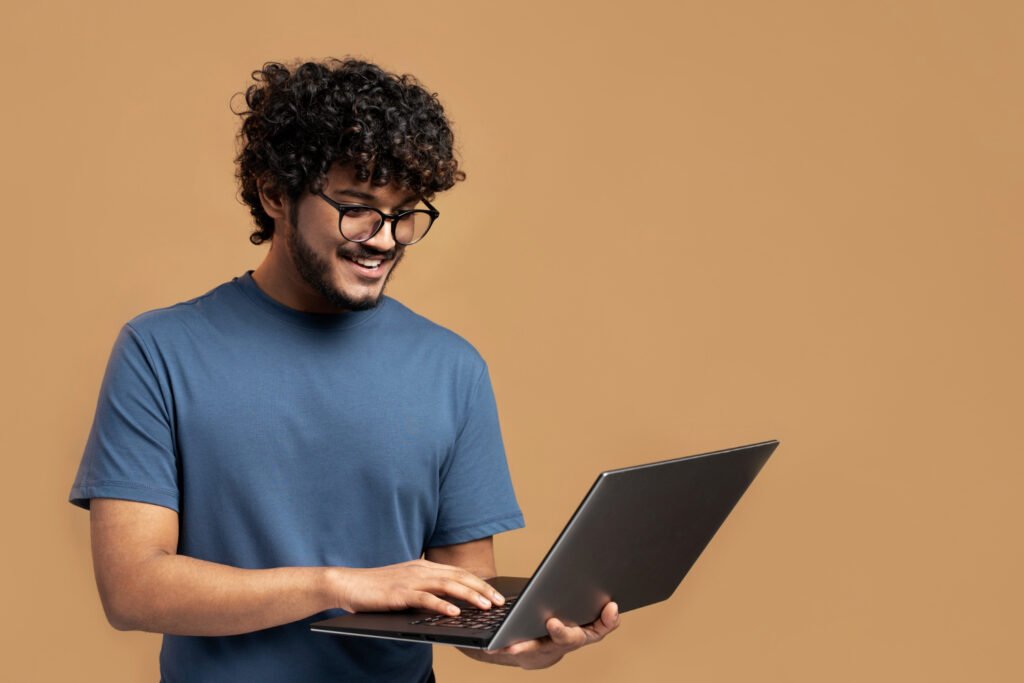
Character array declaration in C.
Character array declaration and initialization.
char text[50]; // 50 Character array declaration named string text of size 50.
str[0] = ‘V’;
str[1] = ‘c’;
str[2] = ‘a’;
str[3] = ‘n’;
str[4] = ‘h’;
str[5] = ‘e’;
str[6] = ‘l’;
str[7] = ‘p’;
str[8] = ‘s’;
str[9] = ‘u’;
str[10] = ‘\0’; // Null pointer indicates the end of array string. That means no array character is stored beyond this. And this character array is the string end point.
Character array char[] empty array is used in C program to store string of characters by indexing them sequentially in order.
Array Initialization – You can initialize character array with a sequence of characters. Values can be assigned to each array element manually.
String Null Terminator – While manually initializing character array to represent string in C program, you should put a null terminator (‘\0’) at the end of the string to mark the end point of the string.
Array Character Initialization.
char text[30];
str[0] = ‘w’;
str[1] = ‘e’;
str[2] = ‘l’;
str[3] = ‘c’;
str[4] = ‘o’;
str[5] = ‘m’;
str[6] = ‘e’;
str[7] = ‘\0’; // Null terminator is added to indicate the end of the string.
printf(“\n the array string is – %s”, text); //result – string array is – welcome
In the above program text is a character array which stores the string “welcome” with an explicit null terminator.
String Literals in C.
String Literals in C Program.
const char *text = “welcome”;
String literal data type in C is a sequence of characters enclosed in double quotes (“).
constant – String literals in C are constant in nature, and usually stored in memory for reading in C program.
Null Terminator – String literals are automatically terminated by the C program compiler at null-pointer location.
String Literal Example in C.
const char *text = “welcome”;
printf(“\n String literal is – %s”, text); // String literal is – welcome
In this program, text displays a string literal “welcome” text, which is automatically terminated at null pointer end.
Difference between character array and string literals.
Mutability – Character array in C can be modified after being introduced in the program, which allows dynamic changes in declared string content. In C, declare string Due to the nature of literals being constant, they can never be modified in a C program.
Storage – Character arrays in C are stored in stack or heap order, whereas string literals declared in a C program are usually stored in primary memory for read-only purposes.
Initialization – Character arrays in C are explicitly started and ended with a null character (‘\0’). String literals declared in a C program are automatically terminated with a null pointer by the program compiler.
Uses of Character Arrays and Literals in C.
Character arrays – When you ever need to dynamically modify string content in a C program, such as modifying string characters or adding, deleting, etc., then use character arrays.
String Literals – Use string literals constant data type when you want to use already fixed constant strings and the string is immutable and does not need to be modified during program execution. Sometimes you will find it helpful to use string literal constants or fixed string data in your program.
String Arrays and String Literals Important Points.
When you declare string array in C program, make sure that character array is properly null terminator pointer to avoid problems with string functions that depend on null terminator.
String literals in C program initialize string and provide fixed constant character values but they cannot be modified at program compile time, thus creating a read-only environment for string literals at program compile time.