Setting Up .NET Core Projects
.NET Core is a cross-platform operating system, open-source development framework for graphical software development to create and develop modern advanced applications. .NET Core allows programmers to create multi-platform applications. It includes many universal platforms, including Windows, Linux, Mac OS, and create, test, and execute these applications on operating systems.
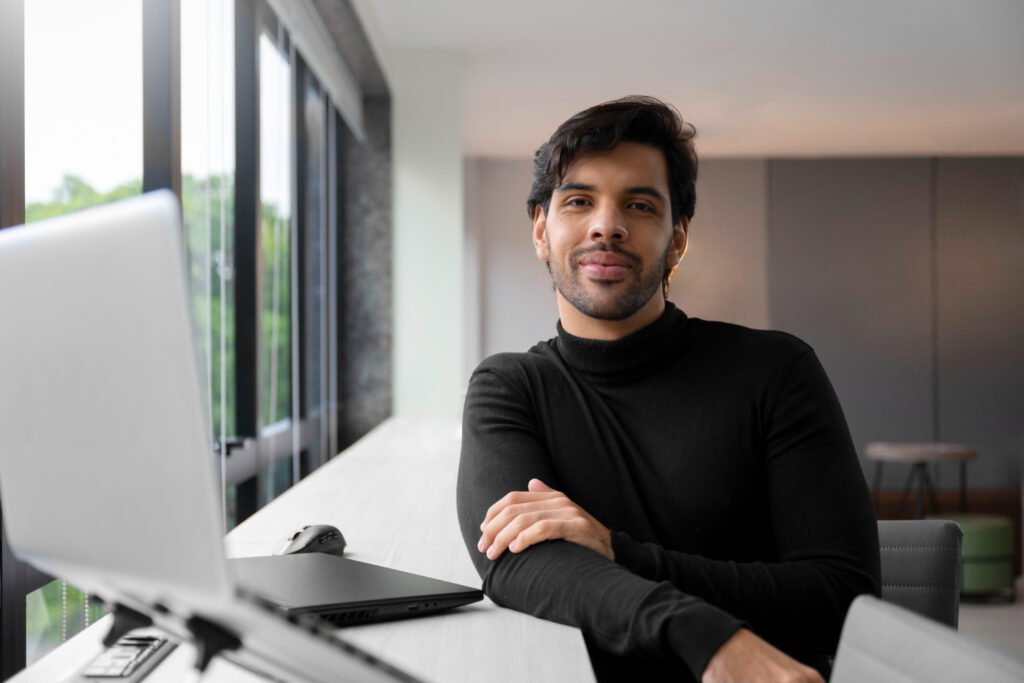
From basic to advanced programs To set up a .NET Core project, you need to follow some steps.
So, let’s know them in a better way.
Here are the steps to set up a .NET Core project, which includes installing important development tools, creating a new project, and executing it.
First, install the .NET Core SDK on your computer.
To start the .NET Core framework on your desktop, laptop, you need to manually install the .NET Core SDK version. Where everything is present in the SDK toolkit. Which helps programmer developers from .NET Core application design development testing to execution.
Just visit the official .NET website.
To download the .NET SDK for your existing desktop, laptop operating system including Windows, Max OS, Linux, move to https://dotnet.microsoft.com/download.
Install the SDK version on your computer.
Follow the SDK installation instructions properly for your existing desktop laptop computer platform. You can open the terminal or command prompt application in your computer and confirm the installation by executing the command given below.
dotnet –version
This command displays the .NET Core SDK version information installed in your computer.
Create a new .NET Core project.
After installing the .NET Core SDK version in your desktop laptop computer, developers can develop different projects or software applications of multiple types such as console applications, web applications, or libraries.
Creating a .NET Core console application.
- Some basic steps have to be followed to create a new .NET Core project in the console terminal screen.
- First of all open your terminal application in your computer. In which start Command Prompt or PowerShell on Windows.
- In that terminal or shell, go to the directory where you want to develop your project.
To create a new console application in your computer, apply the command given below.
dotnet new console -n MyFirstApp
console – This defines the type of an application console app in your project.
-n TestApp– Here you set the name of your project folder to TestApp.
Here this command creates a new folder named TestApp with the structure given below.
TestApp/
├── Program.cs
├── TestApp.csproj
└── bin/
└── Debug/
└── netcoreapp3.1/
└── TestApp.dll
- Program.cs – This is the main entry point of the developed application.
- TestApp.csproj – This is the project file containing the project configuration.
- bin/ – This is the build output in your program.
Now go to your project stored directories location.
cd TestApp
Write some basic program code for .NET Core project development.
On your desktop or laptop computer, open the program.cs file in your favorite text editor, code editor software like Visual Studio Code, Visual Studio, or Notepad and then you will get the following application default code.
using System;
class Program
{
static void Main(string[] args)
{
Console.WriteLine(“Hi, Vanhelpsu!”);
}
}
This prints the message Hi, Vanhelpsu! in the console when a basic program is executed.
Create the projects you need and run them.
Create a new project – To create a new project in .NET Core, run the following command in your computer.
dotnet build
This command compiles your existing program application, and produces the required program output in the bin/ folder in .NET Core.
Run an existing project – To run the .NET Core application you developed, apply the following command.
dotnet run
After executing this command, you should see this in the output screen.
Hi, Vanhelpsu!
Adding Dependencies (NuGet Packages) in .NET Core
You can add external libraries or required packages to your existing project using the NuGet .NET package manager. For example, if you want to add a JSON library file like Newtonsoft.Json to your project.
Run the following command to install the package in your project.
dotnet add package Newtonsoft.Json
Update the Program.cs file – After adding the required package to your existing project, you can use it in your source code. Update the Test.cs file to add the JSON file serialization.
using System;
using Newtonsoft.Json;
class test
{
static void Main(string[] args)
{
var employee = new { Name = “Ajay”, Age = 40 };
string json = JsonConvert.SerializeObject(employee);
Console.WriteLine(json);
}
}
Rerun the project – After storing your existing project file in the computer, execute dotnet run again, and the output should display the JSON string.
{“Name”:”Ajay”,”Age”:40}
Publishing a .NET Core application.
When you are ready to publish your developed .NET Core application, you can publish it anytime. Publishing compiles and packages your application in a folder along with all the required dependencies.
Run the following command to publish a .NET Core application.
dotnet publish -c Release
This will create a release build file in the bin/Release/netcoreappX.Y/publish/ folder.
Run the published application – Here you move to the publish/ folder directory and run the executable build file directly. You can also deploy this project on a server, or share it with other users.
Creating other .NET Core CLI project types.
Apart from console application development, you can create projects of other types of software, web applications, console applications with .NET Core CLI.
Create and execute a web application ASP.NET Core Web API.
dotnet new webapi -n TestApiApp
Class Library – To create a class library that you can reference from other projects, and execute it as needed.
dotnet new classlib -n MyLibrary
Unit Test Project – Create and execute a unit test project in your development project.
dotnet new xunit -n TestApp
Blazor WebAssembly Application – To make your development project an interactive client-side web application, run this code.
dotnet new blazorwasm -n TestBlazorApp
You can get a list of available project templates by running the following command on your computer.
dotnet new –list
Use Visual Studio or Visual Studio Code (optional) software.
For a more graphical rich set programming development environment experience, you can use Visual Studio Code or Visual Studio Graphical Source Code Development Application.
Visual Studio – Software Development Project is an integrated development environment application software with advanced features such as debugging, IntelliSense, and GUI-based design for web applications.
Download it from here.
Visual Studio Code – Visual Studio Code is a lightweight, cross-platform application development code editor software. You can use it with the C# extension for .NET development.
Download it from here.
Where you install the C# extension on your computer through the extension marketplace.
.NET Core Conclusion in C# Programming Language.
Generally, now you have learned how to set up a .NET Core project on your computer, and how to write some basic basic program code, how to add the necessary dependencies to the application and how to develop and execute your application. You can further explore .NET Core for more complex application development such as your advanced web application API, mobile apps, or desktop applications.