Single-Dimensional and Multi-Dimensional Arrays
Like other programming, arrays are a basic concept of programming in C# programming language, which allows C# programmers to store and process homogenous multiple array values of the same type in a program by declaring arrays. In C# programming language, arrays can be stored and processed in single-dimensional (1D) arrays or multi-dimensional 2D dimensional arrays, 3D dimensional arrays, etc. In C# programming language, desired data type variables can be stored and manipulated in a continuous order.
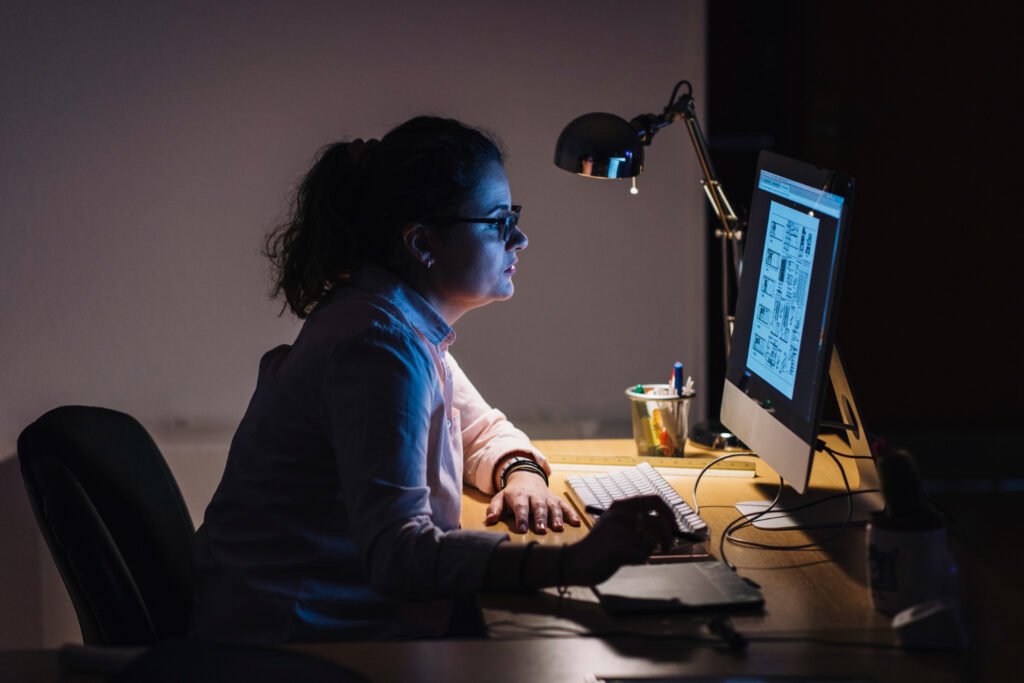
Single-dimensional array in C# programming language.
A single-dimensional array is a storage collection of elements in which all array elements in the declared array are of the same type and are accessed by the same index.
Single-dimensional array declaration syntax in C#.
/Declaring an array of integers in C#
int[] decimal = new int[7]; // declare a list array of size 7
// we can initialize a single dimensional array with default values
int[] decimal = { 9, 1, 7, 0, 3, 4, 6 };
Here in the first step a single dimensional array with fixed size of size 7 is declared.
Similarly in the second method a single dimensional array with fixed value is declared with specific fixed value decimal = { 9, 1, 7, 0, 3, 4, 6 };
Accessing the elements of a single-dimensional array in C#.
C# programmers can access the array element using the array index location, where in this storage array the first array store element is stored at index 0, and so on and array elements are stored in continuous order.
using System;
class Program
{
static void Main()
{
// let Declare and initialize one dimensional array
int[] deimal = { 9, 1, 4, 7, 8 };
// let Access and print one dimensional array
Console.WriteLine(deimal[1]); // the result is – 1
Console.WriteLine(deimal[4]); // the result is – 8
// let Modify an array element
deimal[3] = 24;
Console.WriteLine(deimal[3]); // the result is – 24
}
}
Important points about single-dimensional array in C#.
In C# language, array elements are always stored from zero-indexed location. Where, first array element is stored at index 0 location.
User declared array in C# language has a fixed size, remember, once the array is declared in C# language, its size cannot be changed.
Multi-dimensional array in C# language.
Multi-dimensional array in C# language stores data values in row and column order. Generally, multi-dimensional array in C# language stores and processes data in matrix or tabular format.
Declaring multi-dimensional array (2D) in C# language.
In C# language, a 2D array is generally used to represent data and information in grid or matrix order.
/// Declaring 2D array with 4 rows and 3 columns in C# language.
int[,] table = new int[4, 3]; // here table array declare 4 rows, 3 columns
As per the requirement, you can initialize a multi-dimensional array with array values at the time of multi-dimensional array declaration in C# programming language.
// Declaring 2D array with fixed values in C# language
int[,] table =
{
{9, 4},
{1, 7},
{8, 2}
};
Accessing elements in multi-dimensional array in C# language.
You can access multi-dimensional array elements by indicating 2 dimensional array (row & column) in the current C# program.
using System;
class Program
{
static void Main()
{
// here we Declare and initialize a 2D array with 3 rows, 2 columns
int[,] table =
{
{9, 8},
{1, 2},
{7, 6}
};
// let Access and print the individual 2d array elements
Console.WriteLine(table[0, 1]); // the result is – 8
Console.WriteLine(table[1, 1]); // the result is – 2
// let Modify above array element
table[0, 0] = 27;
Console.WriteLine(table[0, 0]); // the result is – 27
}
}
Declaring and Initializing 3D array in C# language.
A 3-dimensional array in C# programming language is an array table of arrays, variable data in a 3-dimensional array is stored and processed in a cube format.
// Declaring a 3D array with 3x3x3 dimensions in C# programming language
int[,,] cube = new int[3, 3, 3]; // Here the 3d cube array has 3 “slices”, 3 rows and 3 columns in each slice
You can declare and initialize a 3D array with specific values in C# programming language.
int[,,] cube =
{
{
{9, 8},
{1, 2}
},
{
{4, 6},
{0, 5}
}
};
Accessing elements in a 3D array in C# programming language.
In the C# 3D array program, you are provided with a 3d matrix array variable value containing three index elements (for cube slices, rows and columns).
using System;
class Program
{
static void Main()
{
// let Declare and initialize 3D array with (2 slices, 2 rows, 2 columns) structure
int[,,] cube =
{
{
{24, 71},
{34, 27}
},
{
{11, 18},
{19, 20}
}
};
// let Access and print the element from the 3D array
Console.WriteLine(cube[0, 1, 0]); // the result is – 34
Console.WriteLine(cube[1, 0, 1]); // the result is – 18
}
}
Jagged array in C# programming language.
Apart from multi-dimensional array in C# programming language, C# also supports jagged array. Jagged array is an array element in a C# program, where the length of each internal array can be different as per the requirement.
Declaring and Initializing jagged array in C# programming language.
// Declaring 4 rows jagged array in C# program
int[][] jaggedArray = new int[4][];
// Initializing jagged array in C# programming language.
jaggedArray[0] = new int[] { 1, 0, 2 };
jaggedArray[1] = new int[] { 1, 4, 5 };
jaggedArray[2] = new int[] { 9, 3, 2, 1 };
jaggedArray[3] = new int[] { 1, 0, 1 };
You can initialize the jagged array with fixed values as per your requirement.
int[][] jaggedArray =
{
new int[] { 9, 1, 4 },
new int[] { 1, 2 },
new int[] { 1, 0, 7, 2 }
};
Accessing elements in a jagged array in C# programming language.
In C# programming language you can access the jagged array elements using two index arrays (row and column), you will be able to access it from the index location like a multi-dimensional array. While in C# programmer jagged array, the length of the declared internal array can be different.
using System;
class Program
{
static void Main()
{
// let Declare and initialize a jagged array in c#
int[][] jaggedArray =
{
new int[] { 22, 44, 88, 99 },
new int[] { 90, 100, 23 },
new int[] { 36, 87, 18, 09 }
};
// let acess and print individual jagged array elements
Console.WriteLine(jaggedArray[1][0]); // the result is – 90
Console.WriteLine(jaggedArray[2][1]); // he result is – 87
Console.WriteLine(jaggedArray[2][2]); // he result is – 18
}
}
Differences Between Single, multi-dimensional and jagged Arrays
Feature | Single-Dimensional Array | Multi-Dimensional Array | Jagged Array |
Array Structure | It stores A single list of array elements | It store A grid of (2D, 3D, etc.) array | Collection of group multiple Array of arrays |
Array Declaration | int[] value = new value[7]; | int[,] matarray = new int[3,4]; | int[][] jagarray = new int[2][2]; |
Array Indexing | Single index (e.g., array[0]) | Multiple array indices (e.g., arr[1, 2]) | Multiple array indices (e.g., arr[2][3]) |
Array Size | Fixed size | Fixed size | It may Varying sizes for inner arrays |
Array Memory Allocation | Contiguous memory block of array | It store Contiguous memory block | It is a Non-contiguous memory array blocks (each row is a separate array from other) |
Array Access Speed | 1d array Faster | Multi-dimensional array Slower (due to multiple array dimensions structure) | It is Similar to 2D array, but here it depends on the row sizes |
Multiple Array and Jagged Array Conclusion in C# programming language.
- Single-dimensional arrays in C# language are used to store and process homogeneous data of the same type where you can access a single dimensional array element using a single index.
- Multi-dimensional arrays in C# language represent array elements in table grids, matrices order where programmer has to access multi-dimensional array elements using multiple index locations.
- Jagged arrays in C# language provide programmer flexibility in reference declaration of different row sizes making them ideal for program conditions where you need to group different types of single arrays and declare and use them in jagged array format.