Syntax of Lambda Expressions
Lambda expressions in Java programming were first introduced in Java 8 version as a detailed program syntax method in the Functional Programming Interface (an interface with a single abstract method). Lambda expressions enable functional programming concepts in Java programming. This makes the source code written in Java programming programs more readable, easier to use, and more conditional parameter expression compatible.
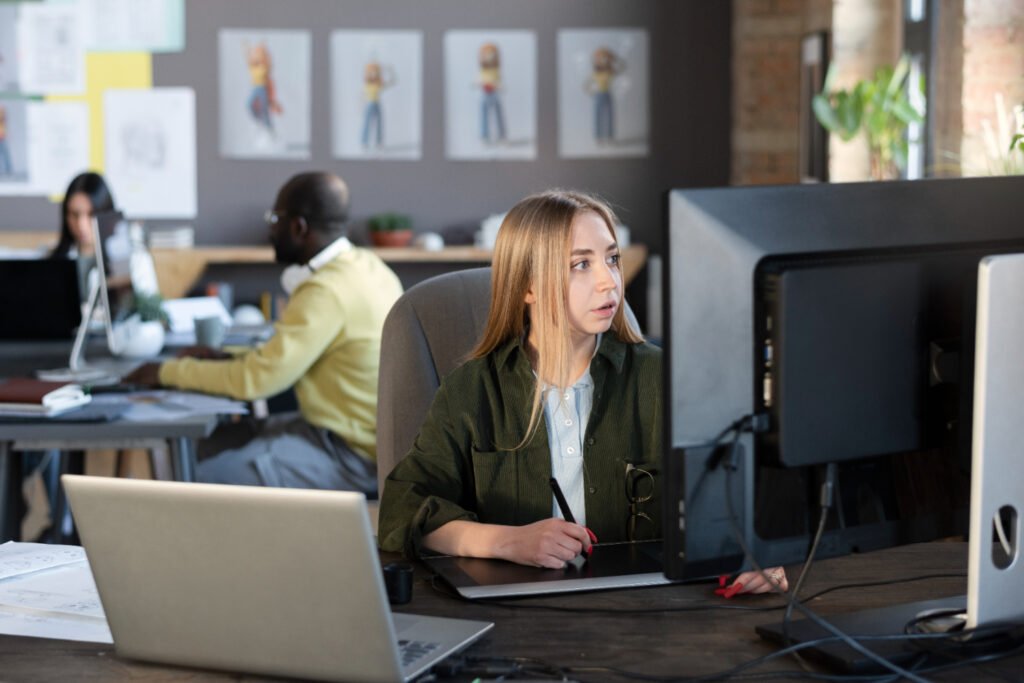
Basic syntax of lambda expression in Java.
The general syntax of a Java program lambda expression is.
(lambda parameters) -> expression
If there is more than one statement in the lambda expression, then.
(lambda parameters) -> { statement }
Components of lambda expression in Java program.
- Lambda parameters – Lambda parameter arguments are displayed in parentheses (), and preview the active input variable argument of the lambda expression. If a lambda expression has only one parameter variable, then you can create other parameters with desired data type as per requirement. So here the brackets for lambda parameter can be omitted as per requirement.
- Arrow (->) – Here the arrow symbol previews the lambda parameter argument list by separating it from the main part of the lambda expression.
- Body – The lambda body portion contains the program logic code expressions to be executed in the main part of the lambda expression. Here it can be either a single lambda expression, or a lambda block expression of multiple statements as per the programmer requirement.
Example of Lambda Expression in Java Program.
No lambda expression parameters in java.
() -> System.out.println(“welcome to vcanhelpsu!”);
In the above lambda expression there is no parameter argument variable declared, and it simply prints the text message “welcome to vcanhelpsu!” on the computer console screen.
Lambda one parameter (implicitly typed) expression.
p -> p * p
Here the lambda expression takes one parameter p argument variable value input from the user, and returns the square value of the p parameter.
Lambda one parameter (Explicitly Typed) expression.
(int q) -> q * q
This is the same lambda expression as the previous example, but in this the data type of the parameter of the lambda expression is explicitly defined to be int data type.
Multiple parameters in lambda expression.
(int p, int q) -> p + q
This above lambda expression takes two integer parameter values p and q argument input, and returns the total value of both of them.
Multiple statements in lambda expression body.
(int p, int q) -> {
int total = p + q;
System.out.println(“\n the total is – ” + ; total);
return total;
}
In the above example, the lambda expression has more than one parameter argument statement. So the lambda body is combined in curly braces {}. This expression prints the total of the p and q parameters, and then returns the total value at the end.
Returning a lambda value.
If the given lambda expression has a clear return data type, you can use the return keyword inside the lambda body.
(int p, int q) -> { return p + q; }
Here, the lambda expression takes two parameter arguments input from the user, and returns the total value of both those parameters.
Lambda Expression with Collections in Java Programming.
You can custom use many types of lambda expressions with data types such as List, Set, etc. in Java’s built-in collections framework to perform programming operations such as filtering, transforming, or iteration in Java programming.
List<Integer> integer = Arrays.asList(9, 7, 8, 2, 1);
integer.forEach(i -> System.out.println(i * 2));
In the above example, the forEach loop iterates over the existing lambda array list, and prints the double value of each element of the lambda expression.
Lambda functional interface.
To use a lambda expression in a Java program, it must be assigned to a variable of a functional interface data type. A functional interface in Java lambda expression is an interface that has exactly one detailed lambda expression summary method. But it can also have multiple default or static lambda methods.
Java Programming Lambda Functional Interface Example.
@FunctionalInterface
public interface testFunction {
int count(int p, int q);
}
Java Lambda expression that implements the calculate method.
testFunction count = (p, q) -> p + q;
Common use cases for lambda expressions in Java.
We can use lambda expressions in Java programs in the collection framework.
We can repeat from start to end in a loop using the forEach Java Programming loop method.
In an existing Java program, lambda filters, maps and other operations on the stream can execute lambda expressions as needed.
Java Lambda event handling.
In GUI (Graphical User Interface) programming, lambda expressions are commonly used to program event handling tasks. Which replaces anonymous internal classes in Java programming.
Lambda thread creation.
Using lambda expressions to create threads in Java programming.
Example of a lambda with the functional interface.
@FunctionalInterface
interface Calculator {
int total(int p, int q);
}
public class Lambda {
public static void main(String[] args) {
// here Lambda expression implementing the total method
Calculator calc = (p, q) -> p + q;
System.out.println(“\n the total is – ” + calc.total(1, 2));
}
}
Lambda Expression Summary in Java Programming.
- Lambda basic syntax – This is the default basic (parameters) -> expression or (parameters) -> { statement } lambda expression syntax.
- No lambda parameters – This indicates empty no lambda () -> expression in lambda expression.
- One lambda parameter – p -> This indicates the data type of the p parameter in the current lambda expression which can be inferred.
- Multiple lambda parameters – This indicates the return type of (p, q) -> multiple lambda expressions in the current lambda program expression.
- Lambda with multiple statements – This indicates the return type of lambda data type with multiple lambda expressions in the current (p, q) -> { statement1; statement2; return result; }.
- Functional Lambda Interface – This is a functional lambda interface to the target type for the current lambda expression. Which contains a detailed methods for lambda expression.
- Lambda Common Use Cases – Lambda expressions in Java programming are used in functional programming, gui event handling, and data collection processing.