Introduction to lambdas in c++
Lambda expressions are a powerful programming attribute or feature introduced in C++11 programming that allows C++ programmers to directly define anonymous functions or function objects inside their program code. Lambda expressions help you define small, temporary functions or program operations in your existing program. C++ programmers often need to program algorithms, callbacks, or other programming conditions where a small lambda function expression is needed. Lambda expressions are fundamental features for modern C++ programming, enabling C++ programmers to develop advanced expression-based, clear, and readable program source code.
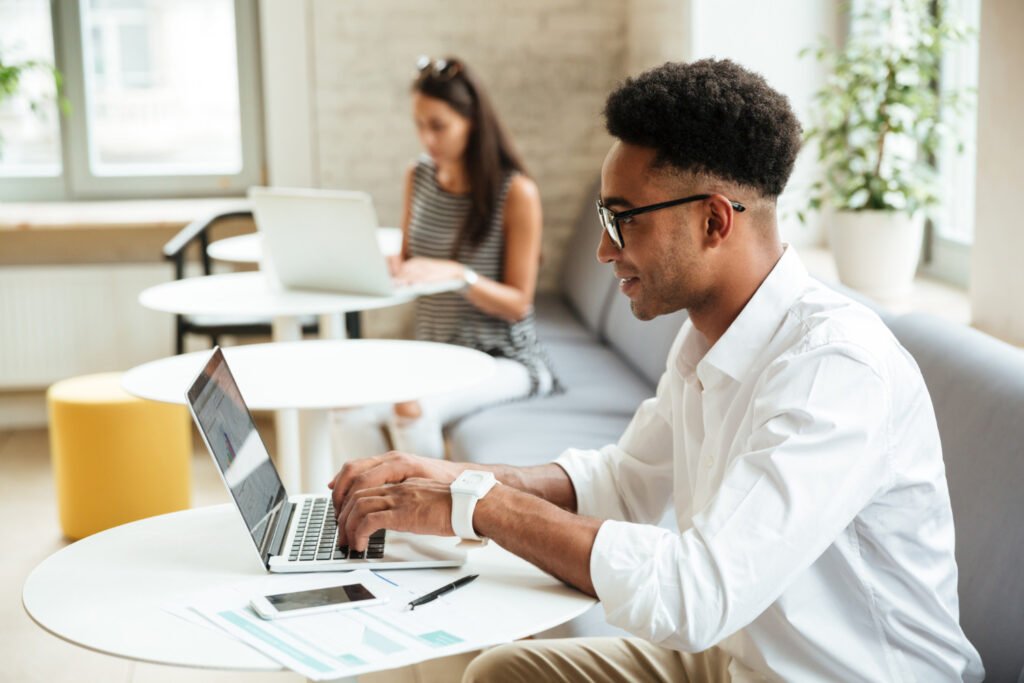
So let’s get to know lambda function expressions better in C++11 programming.
Basic syntax of lambda in C++11 programming.
[ capture ] ( parameter/argument_list ) -> lambda return_type { function_body }
- Lambda capture clause [captures] – Lambda capture clause indicates which variables are to be captured by the lambda expression from the nearest scope in the current program. In this process, local variables may also be present in the current program, where C++11 programmers can capture these lambda expressions through values and program references.
- Lambda parameter list ( parameter_list ) – Lambda parameter list is the list that the lambda expression processes as input arguments or parameter variables. Here if a lambda expression does not display parameter arguments, then this lambda expression may be an empty expression.
- Lambda return type -> return_type (optional) – It indicates the return data type of the lambda expression. If the lambda expression is empty, then the C++11 compiler will automatically find the lambda return type expression.
- Lambda function body {function_body} – It contains the body code of the lambda expression, which contains the main lambda function expression logic or code to create it.
Examples of Simple Lambda in C++ Programming.
Lambda Expression Example without Parameters.
#include <iostream>
int main() {
// create basic lambda expression use to prints “welcome to vanhelpsu!”
auto vcanhelpsu = []() {
std::cout << “\n welcome to vanhelpsu! ” << std::endl;
};
vcanhelpsu (); // it Call the above vcanhelpsu() lambda function
return 0;
}
In this above example.
In this program above, the lambda expression []() {std::cout << “welcome to vanhelpsu!”; } does not input any parameter variable value from the programmer, and when the vcanhelpsu function is called at the time of program execution, it simply prints the text message “welcome to vanhelpsu!” on the console screen.
Lambda Example with Parameters in C++.
#include <iostream>
int main() {
// hre count Lambda funtion that takes two integers parameter p & q and returns their count total
auto count = [](int p, int q) -> int {
return p + q;
};
std::cout << “\n Total is – ” << count(1, 2) << std::endl; // the total count is = 3
return 0;
}
Here in this lambda example above.
In this above lambda expression [](int p, int q) -> int {return p + q; } Accepts two integer parameter variables as input, and returns the total value of these two variables.
In this program, the lambda function return type is indicated as -> int data type.
Capturing a lambda variable in C++ programming.
One of the special attributes of lambda expression in C++ program is capture clause []. Where capture clause captures parameter variables from the scope surrounding the lambda in the current program, so that they can be used anywhere inside the lambda body as per the need.
Lambda variable capture example by value.
#include <iostream>
int main() {
int p = 1;
int q = 2;
// here Lambda expression captures p and q parameter value
auto total = [p, q]() {
return p + q; // here p and q used to captured by value
};
std::cout << “\n the total is – ” << total() << std::endl; // the total is -3
return 0;
}
Here in this lambda example.
In this lambda program expression, p and q are indicated by the value [p, q ], which captures the input variables in the program.
Where the p and q lambda parameter values are copied into the lambda, which means that modifications made to the p and q parameter variables outside the lambda expression do not affect the values used in the lambda.