try, catch, and throw c++
Exception Handling in C++ Programming with: try, catch, and throw.
In C++ programming, exception handling provides a good mechanism to manage and control C++ program runtime errors and unconditional programming conditions in a proper structured order. Instead of manually testing the program error code after each operation in the existing C++ program, exception handling helps you to find program errors during C++ program execution and manage the program source code in a more better order and control them in readability order.
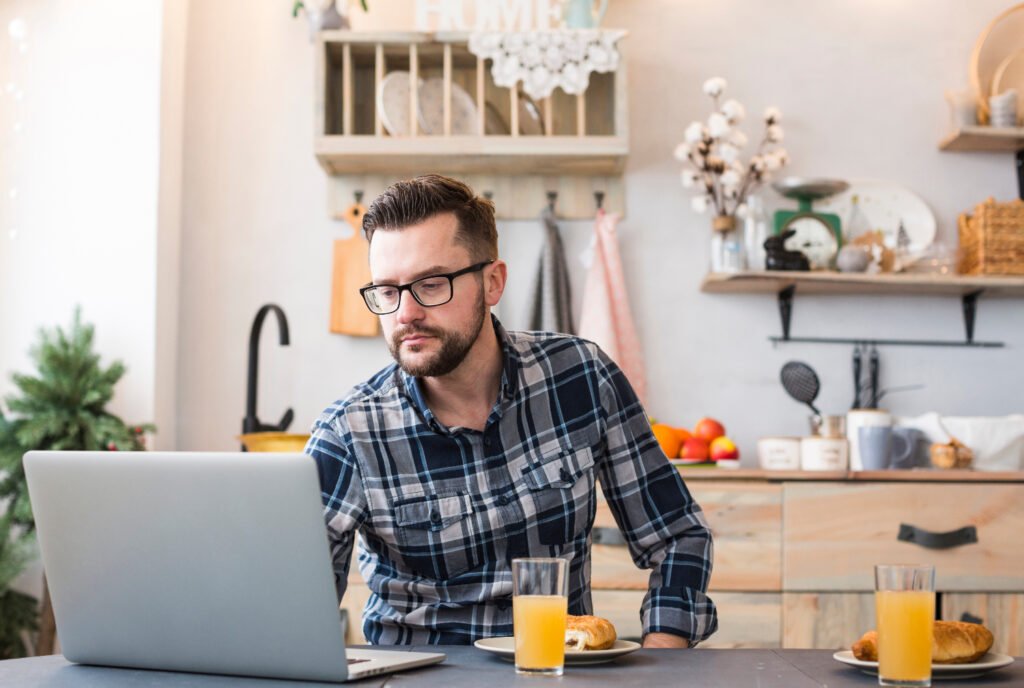
Let’s get to know the try, catch, and throw keywords better to handle program error exceptions in C++ programming.
throw keyword in C++ programming.
In any C++ program, throw keyword is used to throw exceptions in C++ program. When you throw an exception in a C++ program, it will stop the function code execution in the active program, and the corresponding catch block in the current program will automatically move to it if it exists.
Syntax of throw keyword in C++ programming.
throw expression;
- Throw expression – It is the value or object in the current program that you want to throw in case of an exception in your program. Where usually it is a program exception object. Which can be a built-in data type in the current program such as an integer data type or string data type or a user defined custom class object of an individual class. Which operates with legacy std::exception in C++ programs.
Example of throw keyword in C++ programming.
#include <iostream>
using namespace std;
void testvalue(int value) {
if (value < 0)
{
throw “Let tray value will be negative”; // it Throw an exception when value is negative value
}
cout << “\n value is valid – ” << value << endl;
}
int main()
{
try
{
testvalue(-1); // it will throw an program exception when it found
}
catch (const char* textmsg)
{
cout << “\n display exception – ” << textmsg << endl; // it Catch the thrown exception and output error message
}
return 0;
}
- Throw keyword Explanation – In the above program example, the testvalue() function throws an exception in the program when the value is negative. The catch block in the current program catches the program exception, and prints the programmer generated text message to the screen output.
try block in C++ programming.
In C++ programming, the try block is used to wrap the code in any program that throws exceptions in the active program code. Here, if any manual exception is thrown inside the try block, it will immediately transfer the control to the catch block in the related program as per the current program.
Syntax of try in C++ programming.
try {
// type program Code use to throw an exception
}
Remember that the program source code written inside the try block is executed normally. If any program exception is present in it, it is thrown during program execution, and the program source code remaining in the try block is left as it is.
Example with try block in C++ programming.
#include <iostream>
using namespace std;
int division(int p, int q)
{
if (p == 0)
{
throw “\n the p value divide by zero error”; // it Throw an exception if the value is zero
}
return p / q;
}
int main()
{
try
{
int output = division(7, 0); // it will output throw an exception
cout << “\n the output is – ” << output << endl;
}
catch (const char* textmsg)
{
cout << “the outpur is Error – ” << textmsg << endl; // it catch block code exception and display output message
}
return 0;
}
try block Explanation in C++ Programming – Here in the above program, the try block manually calls the division() function. As soon as an attempt is made to divide the value by zero in this program, an exception is thrown in the code. Similarly, it transfers the control in the program to the catch block, which controls the exception as per the program requirement.
Catch Block in C++ Programming.
The catch block in C++ programming is used to catch the program code exception thrown in the try block. Which indicates in the current program that what type of program code exception it can manage and control in the current program. Here you can have multiple catch blocks provided to manage and control multiple types of program exceptions in a C++ program.
Syntax of catch block in C++ programming.
catch (exception_type parameter) {
// write Code to handle program exception
}
- exception_type – It defines the type of program exception in the current program, which is caught during program execution. For example, it can be int, std::exception or custom exception class.
- Parameter – The parameter argument is an object method in the program exception type in the current program. Which holds the exception data and information for the program.
You can catch any program code exception type using catch-all block in C++ programming as per your programming need.
catch (…) {
// type the program block to catches all program exceptions
}
Example with multiple catch blocks in C++ programming.
#include <iostream>
using namespace std;
void trayFunction(int p)
{
if (p == 0)
{
throw ” Zero value is not allow”; // it Throw a program exception type const char* data type
}
else if (p < 0)
{
throw -1; // it Throw a program exception of datatype int
}
else
{
cout << “you enter Valid value – ” << p << endl;
}
}
int main() {
try {
trayFunction(0); // it will throw a const char* program exception
} catch (const char* textmsg)
{
cout << “it Caught program exception – ” << textmsg << endl;
}
try
{
trayFunction(-7); // it will throw a int program exception
}
catch (int errorCode)
{
cout << “\n it display Caught program error code ” << errorCode << endl;
}
return 0;
}
- Catch Block Explanation – Here in the above program you get to know how it controls and manages multiple types of program exceptions in the program.
- When trayFunction(0) is called in the current program function, it throws a const char* code exception which is caught and controlled by the first catch block in the current program.
- When trayFunction(-7) is called in the active program, it throws an int exception in the current program which is caught by another catch block.