What is typescript language
Microsoft created and maintains the free and open-source programming language known as typescript. Because it is a superset of javascript, all legitimate javascript code is also valid typescript code. Javascript gains optional static typing, additional capabilities, and other benefits from typescript, making it a more robust and versatile language for creating complex applications.
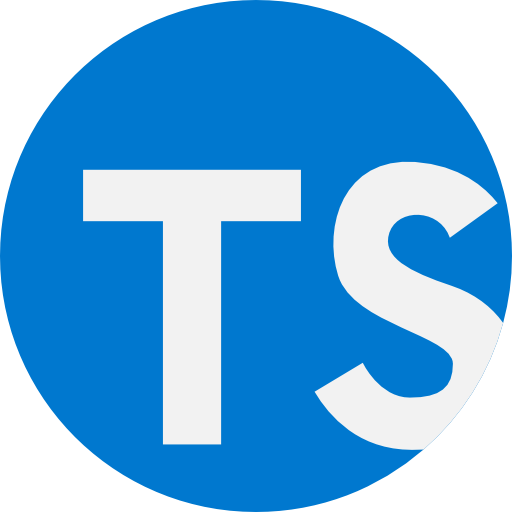
Support for static typing is one of typescript’s core features. Static typing enables it compiler to detect type problems at compile-time rather than at runtime by allowing you to declare types for variables, function arguments, and return values. This improves the robustness and maintainability of your code and helps to prevent problems.
Typescript also includes other functionality.
- Definitions of classes and interfaces for writing object-oriented programming
- Assistance with modern javascript technologies like async/await and generators
- A module approach for grouping reusable parts into code
- Type inference, which in certain situations lets you skip type annotations
- A wide range of built-in types and methods for typical programming tasks
- Support for well-known editors and ides that offer code completion and error highlighting based on typescript types, such as visual studio code.
Last but not least, it is a strong and adaptable language that blends javascript’s greatest traits with contemporary programming languages ideas like static typing and object-oriented programming. Large-scale applications are frequently built using it, especially in the web development industry.
Basic of typescript language
Here are some these language fundamentals.
Static typing is a feature that typescript adds that enables developers to declare types for variables, function arguments, and return values. This makes the code more dependable and simpler to maintain by catching type problems at compile-time.
- Object-oriented programming – concepts from object-oriented programming (oop), including classes, interfaces, and inheritance, are supported by it. This enables programmers to create well-structured, reusable code.
- Es6 features – it is compatible with many of the new es6 features, including arrow functions, the let and const keywords, and destructuring assignments.
- Modules – a technique to organize code into reusable components, it supports modules. With the ability to import and export modules, a modular design enables developers to create complex applications.
- Interfaces – interfaces are a way to specify the shape of objects. Without mentioning an implementation, they offer a contract for the attributes and operations that an object should possess.
- Tooling – a command-line compiler, a language service for code editors, and support for popular development tools like visual studio code and webpack are all included with it.
Pros and cons of typescript language
Here are some advantages and disadvantages of the programming language typescript.
Pros.
- Static typing – typescript’s static typing feature enables compile-time error detection, improving the reliability and maintainability of the code.
- Object-oriented programming (oop) – is supported by typescrpt, which makes it simpler to organize and reuse code by supporting oop concepts like classes, interfaces, and inheritance.
- Es6 capabilities – it supports many of the ecmascript 6 (es6) capabilities, making it simpler to develop effective and contemporary code.
- Type inference – in some circumstances, typescript may infer types, eliminating the requirement for explicit type annotations and streamlining the code.
- Tooling – it has a robust collection of tools and integrations that make it simpler to use and integrate into projects that already exist.
Cons.
- Learning curve – compared to normal javascript, it has a higher learning curve, especially for those who are unfamiliar with static typing or oop.
- Overhead – it adds additional code overhead and compilation time, which might impede development.
- Limited adoption – compared to ordinary javascript, it has had less adoption, therefore there may be fewer tools and libraries available.
- Strictness – some developers may find typescript’s static typing to be overly severe, making it more challenging to build code that deviates from the anticipated type system.
How to download typescript in windows
Follow these instructions to get it.
- Visit https://www.typescriptlang.org, the typescript language’s official website.
- In the top-right portion of the page, click the “download” button.
- Select the bundle that is right for your platform. Node.js, npm, visual studio, visual studio code, and other tools can be used to install typescript.
- To install typescript, follow the instructions for the platform you’ve chosen.
- You may launch a command prompt or terminal window and use the following command to install typescript using node.js and npm.
Install typescript with npm
Your system will then have typescript installed globally so that it may be used in any project. After that, you may use the tsc command at the terminal to compile typescript files.
How to install typescript
- You must have node.js and npm (node package manager) installed on your machine in order to install it. The steps to install typescript are shown below.
- Get node.js and install it. Visit https://nodejs.org/en/, the official node.js website, and download the version that is compatible with your operating system. Install node.js by following the installation instructions.
- After node.js has been installed, you can start your computer by opening a command prompt or terminal window.
In the search box on windows, type “cmd” and choose “command prompt” to accomplish this. Ctrl + alt + t on a mac or linux keyboard will launch a terminal window.
Use the command prompt or terminal window to enter the following command to install typescript using npm.
Install typescript with npm
- By doing this, it will be installed globally on your machine and made accessible for usage in all projects.
- Check the installation – type the following command and press enter to make sure it was installed successfully.
Tsc –version
When installed properly, this ought to show the ative compiler’s version number.
Once it has been installed, you can use the tsc command to compile typescripts code into javascript and begin writing typescripts code.
Typescript-language server
A tool that offers typescripts language services including code completion, formatting, and syntax checking is referred to as the typescripts language server. Any editor that supports the language server protocol can utilize it because it is included within the typescripts compiler.
Installing a plugin or extension that supports the language server protocol will be necessary if you want to utilize the typescripts language server with your editor. The typescripts language server in visual studio code may be installed using the following methods.
- Download visual studio code – install visual studio code if you haven’t already by downloading it from the website at https://code.visualstudio.com.
- Install the typescript extension – by opening visual studio code and selecting the extensions view using the ctrl+shift+x keyboard shortcut or by clicking the extension’s icon in the left-hand sidebar. Click “install” after finding the “typescript” extension.
- Configure the typescript language server – open the user settings by selecting “file” > “preferences” > “settings” or by pressing ctrl+, to configure the typescript language server. Select “typescript: path” after typing “typescript: path” into the search bar. Put the path to your typescript installation in the value (for example, “c:\users\yourusername\appdata\roaming\npm\node_modules\typescript”).
- Enable the typescript language server – open a typescript file in visual studio code and wait for the extension to fully load before enabling the typescript language server. The typescript language server should provide a notification at the bottom of the screen reading “typescript language server is listening on…” this indicates that your typescript code’s language server is now activated and offering language services.
You may now take use of these language server’s services, such code completion and syntax checking, to enhance your typescripts development experience.
Version of typescript programming
Programming language typescripts is continuously expanding, and new versions are often published to provide new features, repair bugs, and enhance speed.
The most recent stable release of typescripts as of april 2023 is version 4.5.5, which was made available on january 19, 2023. This version has a number of new features, including support for decorators on enum members, improved type inference for conditional types, and more flexible tuple types.
You may launch a command window or terminal window and enter the following command to determine the version of the typescripts that is currently installed on your system.
tsc –version
This should provide information like as “version 4.5.5” regarding the version of the compiler that is now active on your computer system.