Pattern matching with re module in python
String Programming Expression Pattern Matching with re Module in Python Programming You can search and manipulate Python text strings based on regular condition expressions (regex). The re module in Python provides a simple and easy way to search text, match text strings, and manipulate string text operations using regular string expressions.
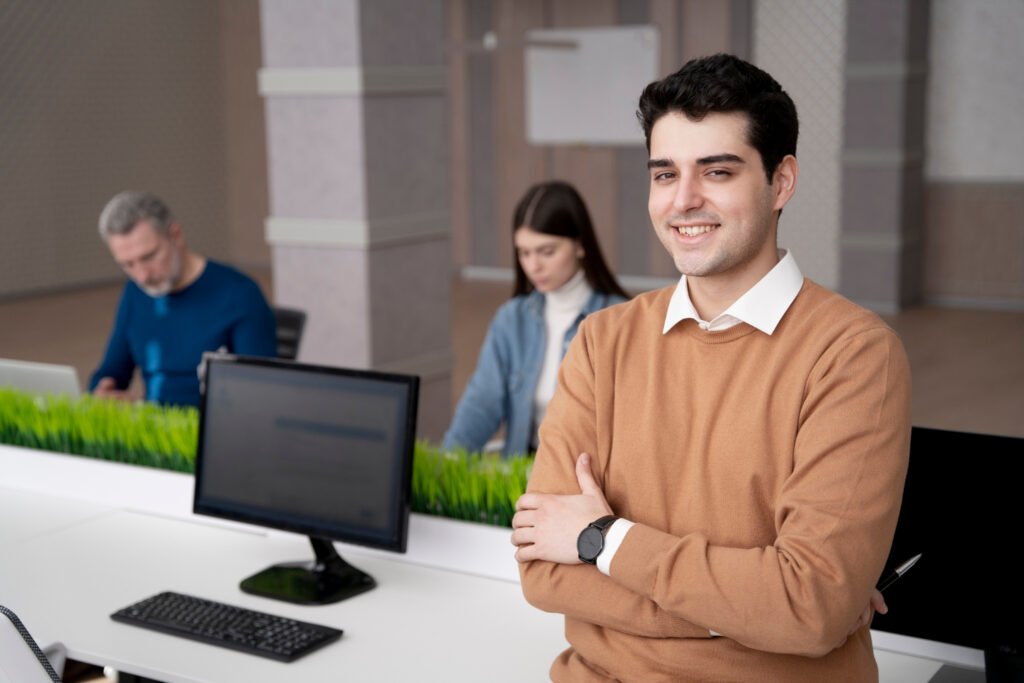
Using re Module for Pattern Matching in Python Programming.
Basic Functions in re Module in Python
`re.search(pattern, string, flags=0)
Pattern search – Regular expression pattern for search string in Python program.
String expression – This is the string text search pattern in which regular string search is executed.
Flags (optional) – These are additional flags in the find string that modify the way text patterns are matched in the search string (for example, string text case sensitive search etc.)
Returns a match search string object if the regular string text is present in the search pattern, otherwise returns the None value.
import re
# let Search fix string/text word ‘vcanhelpsu’ in the given string
output = re.search(r’vcanhelpsu’, ‘hi, vcanhelpsu’)
if output:
print(‘\n search string Pattern found -‘, output.group()) # the result is world – vcanhelpsu
else:
print(‘\n search string Pattern not found’)
`re.match(pattern, string, flags=0)
Similar to the re.search() Python function above, the re.match function only tests the match of string text at the beginning of the current string.
import re
# let try Match ‘python’ in the start of string
display = re.match(r’python’, ‘python, programming’)
if display:
print(‘\n the search string Pattern is found -‘, display.group()) # the result is – python
else:
print(‘\n the search string Pattern not found -‘)
`re.findall(pattern, string, flags=0)
The re.findall() function in a Python program searches for all search occurrences of the pattern in a string, and previews them as a list of strings.
import re
# let tray to Find all integer in the given string
integer = re.findall(r’\d’, ‘vcanhelpsu is 1 the programming 7 website 11’)
print(‘\n search integer found -‘, integer) # the result is – [‘1’, ‘7’, ‘1’, ‘1’]
`re.finditer(pattern, string, flags=0)
re.finditer() returns an iterator value for the test object for all occurrences of the pattern in the string in a Python program.
import re
# let repeat over all occurrences of ‘vcanhelpsu’ in the string
for find in re.finditer(r’vcanhelpsu’, ‘learn programming at vcanhelpsu programming’):
print(‘\n the search string Pattern found in -‘, find.start()) # the result is – the search string Pattern found in – 21
`re.sub(pattern, repl, string, count=0, flags=0)
re.sub() in Python replaces the occurrences of a pattern in a string with the replace string (repl) in the re.sub module.
import re
# let tray to replace all digits with ‘p’
alter_string = re.sub(r’\d’, ‘P’, ‘vcanhelpsu 7 offer python 9 and java 11 language’)
print(‘\n the altered string is -‘, alter_string) # the result is – vcanhelpsu P offer python P and java P language
Python re module Regular expression patterns.
Regular string text expressions (regex) in Python programs use special characters and sequences to define search patterns. Here are some common pattern types used in string expressions.
.: – It matches any character except newline.
^ – It matches string text at the beginning of the current string.
$ – It matches the end of the search string.
\d – It matches any decimal integer number in Python programs. where searches for decimals equal to [0-9].
\w – Matches any alphanumeric character in the Python program, where matches the string equal to small and capital letters [a-zA-Z0-9_].
\s – It matches any empty space character (space, tab, newline) in the current text string.
[] – Tests any single character value within brackets in the Python program string.
* – Tests zero or more occurrences of the previous pattern in the Python string.
+ – It tests one or more string occurrences of the previous pattern in the Python program.
? – It matches zero or one occurrence of the pattern in the previous Python program.
{m,n} – It tests at least m and at most n search occurrences of the previous pattern in the text string.
re module flags.
The flags in the re module modify the method to match patterns in a Python search string. Here is a description of some of the commonly used flags in the re module in Python.
re.IGNORECASE – It matches case-sensitive information.
re.MULTILINE – Matches the ^ and $ symbols at the start and end of each row.
re.DOTALL – . It matches the current string with any character including newline.
re.VERBOSE – It allows you to use multiline regular expressions with comments.
Python re module example: Extracting email addresses
Here is a Python program using regex to display email addresses from a string.
import re
# let use any string that have at least one email address or more
string = “let use vcanhelpsu@gmail.com or another email address name sample@yahoo.com, and similar test@msn.com”
# let use re.findall() python module to find out number of email addresses in string
emailad = re.findall(r'[\w\.-]+@[\w\.-]+’, string)
print(‘\n number of Email addresses found in string is -‘, emailad) # the result is – [‘vcanhelpsu@gmail.com’, ‘sample@yahoo.com’, ‘test@msn.com’]
In the above email search program example, [\w\.-]+@[\w\.-]+ is a regex string email search pattern. Which searches the number of email addresses in the existing string for one or more alphanumeric characters (\w), dots (.), and hyphens (-) before and after the @ symbol.
Conclusion in re module in Python.
The re module in Python programming language provides a strong environment for pattern matching using regular string expressions. It is a powerful tool for searching string text based on complex program patterns, manipulating strings, and validating them. Here is how to understand regular string expressions in Python programs and how to use them in Python programs with re module.