Collections module (deque, namedtuple, defaultdict, etc.) python
The collections module in Python programming provides many alternatives to the built-in data types (dict, list, tuple, set) with additional programming features. These data structures are customized for special uses, making them powerful tools for multiple programming activities.
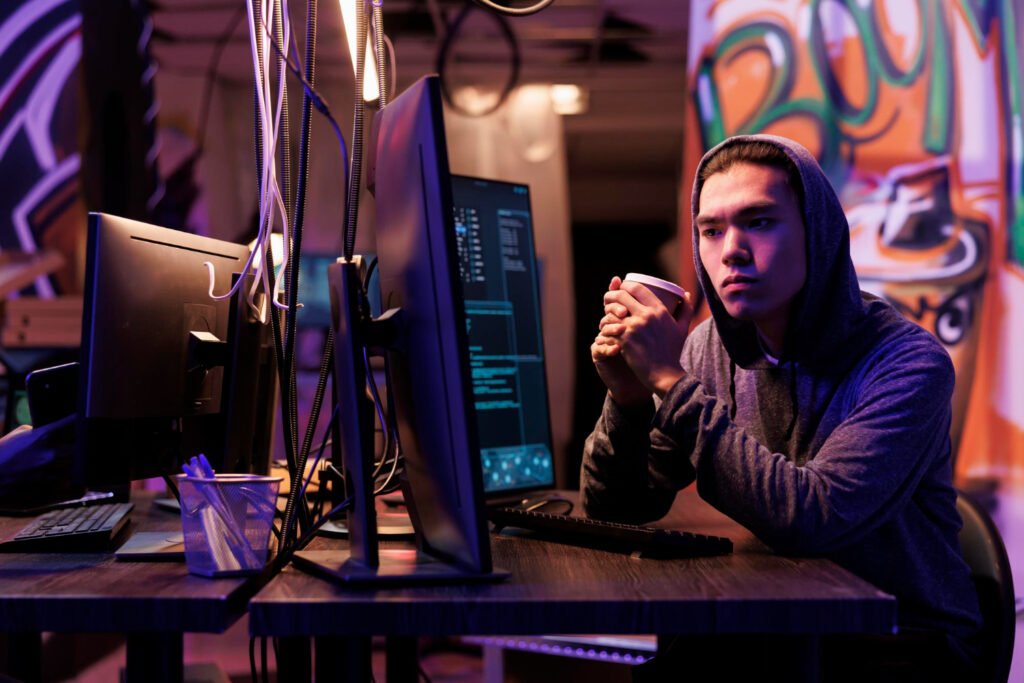
So let’s understand some of the major data structures from the Python program collections module.
python namedtuple
Python namedtuple creates a tuple subclass with named fields. It allows Python programmers to create field access permissions by name instead of condition, which helps Python programmers to create more readable and self-documenting program code.
from collections import namedtuple # import collection from nametuple library
# let define nametuple element
element = namedtuple(‘element’, [‘p’, ‘q’])
from collections import namedtuple # import collection from nametuple library
# let define nametuple element
element = namedtuple(‘element’, [‘p’, ‘q’])
# let create instance for element
e = element(4, 6)
# let display element instance values
print(e.p, e.q) # the result is – 4 6
Dequeue in Python Dequeue (double-ended queue) is a basic process method for creating stack and queue data types. It allows Python programmer to add new elements and remove old elements from both the end points quickly, and dequeue stack is designed to insert new elements and remove existing elements from the stack quickly from its end points.
from Collections import deque # import deque library package
# let Create a new deque
dqstack = deque([7, 9, 2, 3])
# Append right to a Python deque
dqstack.append(10)
print(dqstack) # the result is – ([7, 9, 2, 3, 10])
# Append left to a Python deque
dqstack.appendleft(6)
print(dqstack) # the result is – deque([0, 7, 9, 2, 3, 10])
# Pop a Python deque from the right
print(dqstack.pop()) # the result is – 10
# Pop a Python deque from the left
print(dqstack.popleft()) # the result is – 0
python defaultdict
In Python programming, defaultdict is a data type object similar to a dictionary. Which provides default values for a non-existent key when accessed in a Python program. This makes it easy to create dictionary data types for Python programs that require default values for keys that are not yet defined in the Python program.
from collections import defaultdict # import collection from defaultdict
# let define a defaultdict
default_dict = defaultdict(float)
# let python Increment a non-existent key
default_dict[‘d’] += 2
print(default_dict[‘d’]) # the result is – 2
# Display the default value from accessing a non-existent key in Python
print(default_dict[‘d’])
Python Counter.
Counter in Python library is a dictionary subclass for counting the number of objects available for hash. It is required to count the occurrences of an element in a sequence index.
from collections import Counter # it import counter library
# let Create a new Counter element
count = Counter([‘p’, ‘q’, ‘r’, ‘s’, ‘t’, ‘u’, ‘v’])
print(count)
# Count the occurrences of an element in Python
from collections import Counter # it import counter library
# let Create a new Counter element
count = Counter([‘p’, ‘v’,’q’, ‘p’, ‘r’, ‘t’,’s’, ‘t’, ‘u’, ‘v’])
print(count)
# Python access count of a specific element
print(count[‘v’]) # the result is – 2
python OrderedDict.
OrderedDict is a dictionary subclass in Python programming that remembers the order in which its content information is added. It secures the order of keys as they are inserted into the dictionary.
from collections import OrderedDict # import orderdist in python library
# let Create an OrderedDict element
order_dict = OrderedDict()
# let Add an items in a particular order
order_dict[‘p’] = 4
order_dict[‘q’] = 7
order_dict[‘r’] = 8
order_dict[‘t’] = 11
# Iterating over Python items displays them in insertion order.
for key, value in order_dict.items():
print(key, value)
Uses of the Python Collections module.
Python Named Tuple – Named tuple in Python programs is suitable for lightweight object-oriented programming when you need to design simple, immutable data objects with named fields.
Python Deque – Required for implementing queues and breadth-first search algorithms in Python. Where efficient element append and old element pop operations are needed from both endpoints of the queue.
DefaultDict – This is ideal for applications in Python that need a dictionary with default values for keys not yet defined in Python, such as counting the occurrences of a dictionary element.
Counter – This is good for counting the frequency of elements in a sequence in a Python program, such as counting word frequency in text analysis.