Inheritance in python
Inheritance features is a fundamental programming concept in object-oriented programming (OOP) where you create a new subclass (considered as a derived class made from a subclass or main class) based on a root existing class (considered as a superclass or base parent class) in a Python program. The created subclass automatically inherits the features and methods properties of the main superclass or root class, allowing you to reuse your existing program code efficiently anytime.
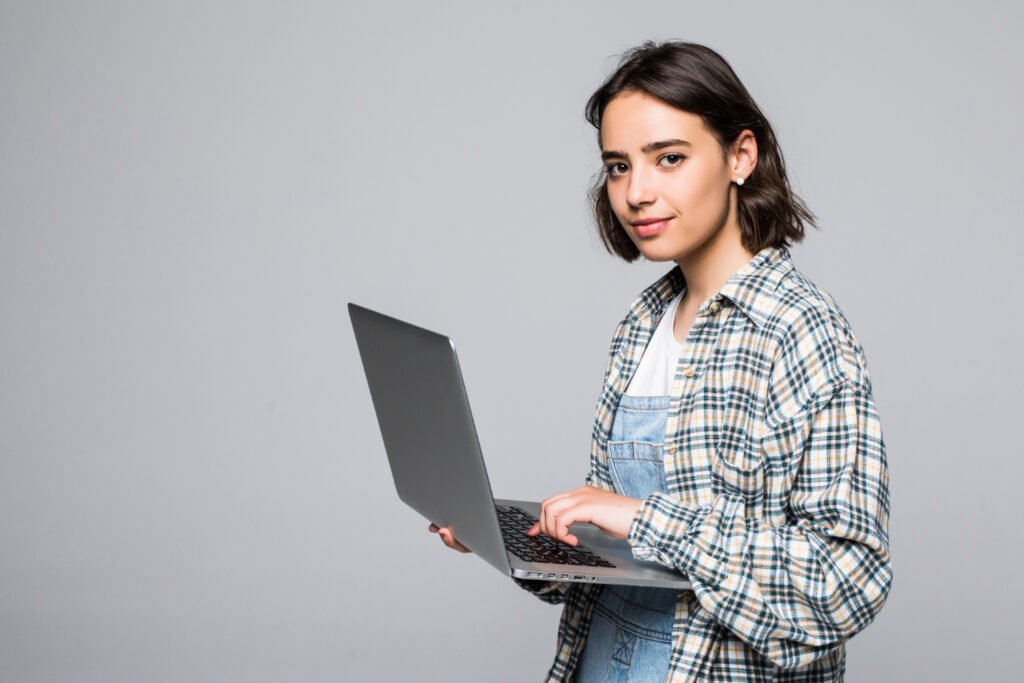
So let’s understand the inheritance properties in Python programming.
The basic syntax of inheritance in Python.
You can define inheritance method in Python programming by following the inheritance class syntax given below.
class Base/rootClass:
# add Base class parameter and attributes
class DerivedClass(Base/root Class):
# it follow attributes of base/derived class
pass
Base class is a superclass or main base class. Which provides base for inherited class with its root class attributes and methods.
Derived class or subclass or derived class is created on the basis of main root class. Which inherits all properties and attributes from main base class to subclass.
Inheritance Program Example in Python.
So let’s create pcourse Java and Python inherit subclass/derived class on the basis of pcourse base class in Python program.
class Base/rootClass:
# add Base class parameter and attributes
pass
class DerivedClass(Base/root Class):
# it follow attributes of base/derived class
pass
A base class is a superclass or main base class which provides a base for inheriting classes with its root class attributes and methods.
A derived class or subclass or derived class is created based on the main root class which inherits all the properties and attributes from the main base class to the subclass.
Inheritance Program Example in Python.
So let’s create pcourse Java and Python inherit subclass/derived class based on the pcourse base class in Python program.
class pcourse: # create pcourse base/root/main class
def __init__(info, name): # define 2 parameter
info.name = name
def select(info):
raise NotImplementedError(“raise error for abstract calss”)
class java(pcourse): # java derived/sub class created
def select(info):
return f”{info.name} you select”
class python(pcourse): # python derived/sub class created
def select(info):
return f”{info.name} you select”
# here Creating instances of derived classes
java = java(“java”)
python = python(“python”)
# call derived class element/parameter
print(java.select()) # result – java you select
print(python.select()) # result – python you select
In the above inherited class example.
In above class pcourse is a base/main class. Which has an __init__ method to initialize the pcourse name attribute and a select method (abstract method) which is overridden in derived classes.
The pcourse base class has subclasses of the Java and Python pcourse superclass. These inherit the name attribute and select method properties from the pcourse class, but the select method has its own class execution method.
Python Method Resolution Order (MRO).
Inheritances in Python programming follows the Method Resolution Order (MRO) method, which decides in which order the properties of multiple inheritance are to be inherited in the Python base class. You can access the MRO object element of a class by using __mro__ attributes or mro() method in a Python program.
print(java.__mro__)
print(python.mro())
Advantages of Python inheritance.
Code Reusability – Python inheritance promotes code reuse in a program by allowing subclasses to inherit attributes and methods from superclasses.
Modularity – Classes in a Python program can be divided into smaller, more manageable units (superclasses and subclasses), which improves program modularity and program maintenance.
Polymorphism – Python inheritance provides the feature of polymorphism, where objects of different multiple classes can be declared as objects of a common superclass, which provides flexibility in programming.
Types of Python Inheritance.
Single inheritance – This is a single subclass follows inheritance from only one super/base class.
Multiple inheritance – This is a subclass follows inheritance from more than one super/base class. Python programming allows multi-inheritance.
Multilevel inheritance – Here in Python a subclass is derived from another subclass, which creates a proper hierarchical inheritance chain.
About Inheritance.
In multiple inheritances in Python, if two super/main classes have a method with the same name, then the method of the super/main class which is at the top in the MRO order is called. If program errors are not managed carefully, this may cause program execution ambiguity.
In Python programs sometimes it may be better or easier to use composition (where an object is composed of other class objects) over inheritance to achieve better flexibility and follow code structure.
Understanding inheritance in Python programming and using it effectively in your Python program code allows you to build on existing program classes, promote reuse of program code, and create hierarchies of related classes.